Responsive Column Layout using React and Tailwind
Last Updated :
17 Dec, 2023
Column Layout is commonly employed on web pages to present content side by side on larger screens, allowing the efficient display of substantial information. On smaller screens where side-by-side viewing is impractical, a row format is adopted using the responsiveness concept.
Preview of final output: Let us have a look at how the final layout will look like:
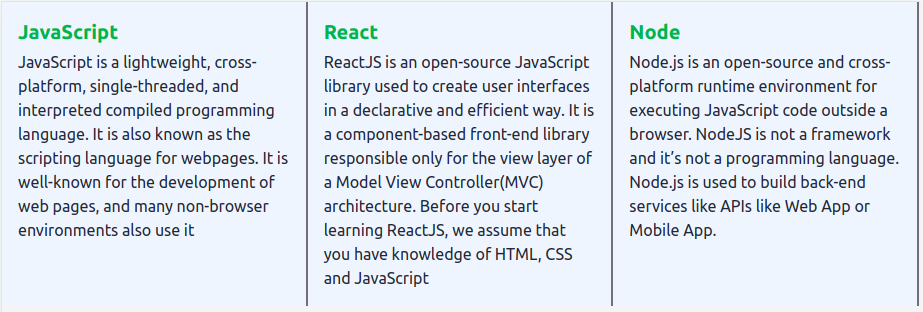
What is responsive design in web development?
A responsive column layout maximizes screen space efficiency, ensuring optimal information display while maintaining a positive User Experience. Additionally, it serves well for facilitating comparisons between multiple components.
Why is a responsive column layout useful?
A responsive column layout maximizes screen space efficiency, guaranteeing optimal information display without compromising the User Experience. Furthermore, it proves effective for facilitating comparisons between various components.
Prerequisites:
Approach to create Responsive Column Layout:
- Set up a basic react project and install the required dependencies.
- Create the basic layout consisting of Columns and add content to it.
- Style the content using Tailwind.
- Add Tailwind classes to make the column layout responsive.
Steps to Create React Application And Installing Module:
Step 1: Set up the project using the command
npx create-react-app <<Project_Name>>
Step 2: Navigate to the folder using the command
cd <<Project_Name>>
Step 3: Install the required dependencies using the command
npm install -D tailwindcss
Step 4: Create the tailwind config file using the command
npx tailwindcss init
Step 5: Rewrite the tailwind.config.js file as folllows
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Project Structure:
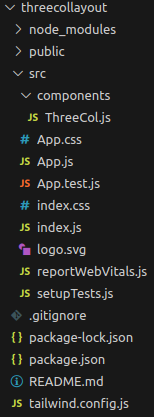
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Write the following code in respective files:
- App.js: This file imports all the components
- ThreeCol.js: This file creates the responsive design for column layout
- index.css: This file includes tailwind css classes
Javascript
import logo from "./logo.svg" ;
import "./App.css" ;
import ThreeCol from "./components/ThreeCol" ;
function App() {
return (
<div className= "bg-gray-100 h-screen" >
<ThreeCol />
</div>
);
}
export default App;
|
Javascript
export default function ThreeCol() {
return (
<div className= "flex flex-col md:flex-row bg-blue-50" >
<div className= "w-full md:w-1/3 p-4
border-b-2 md:border-r-2
md:border-b-0 border-zinc-500" >
<h3 className= "text-xl text-green-600 font-semibold mb-1" >
JavaScript
</h3>
<p className= "text-gray-800" >
JavaScript is a lightweight, cross-platform,
single-threaded, and interpreted compiled programming
language. It is also known as the scripting language for
webpages. It is well-known for the development of web pages,
and many non-browser environments also use it
</p>
</div>
<div className= "w-full md:w-1/3 p-4
border-b-2 md:border-r-2
md:border-b-0 border-zinc-500" >
<h3 className= "text-xl text-green-600 font-semibold mb-1" >
React
</h3>
<p className= "text-gray-800" >
ReactJS is an open-source JavaScript library used to create
user interfaces in a declarative and efficient way. It is a
component-based front-end library responsible only for the
view layer of a Model View Controller(MVC) architecture.
Before you start learning ReactJS, we assume that you have
knowledge of HTML, CSS and JavaScript
</p>
</div>
<div className= "w-full md:w-1/3 p-4
border-b-2 md:border-r-2
md:border-b-0 border-zinc-500" >
<h3 className= "text-xl text-green-600 font-semibold mb-1" >
Node
</h3>
<p className= "text-gray-800" >
Node.js is an open-source and cross-platform runtime
environment for executing JavaScript code outside a browser.
NodeJS is not a framework and it’s not a programming
language. Node.js is used to build back-end services like
APIs like Web App or Mobile App.
</p>
</div>
</div>
);
}
|
CSS
@tailwind base;
@tailwind components;
@tailwind utilities;
body {
margin : 0 ;
font-family : -apple-system, BlinkMacSystemFont, 'Segoe UI' ,
'Roboto' , 'Oxygen' , 'Ubuntu' , 'Cantarell' ,
'Fira Sans' , 'Droid Sans' , 'Helvetica Neue' ,
sans-serif ;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
}
code {
font-family : source-code-pro, Menlo, Monaco, Consolas, 'Courier New' ,
monospace ;
}
|
Steps to Run the application:
Step 1: Type the following command in terminal of project directory
npm start
Output:
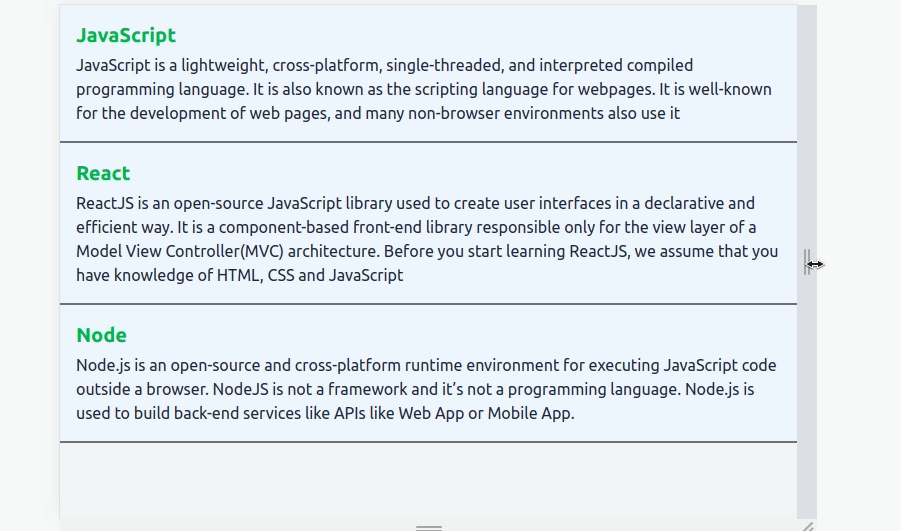
Share your thoughts in the comments
Please Login to comment...