React.js Blueprint TimezonePicker Props
Last Updated :
10 Aug, 2022
BlueprintJS is a React-based UI toolkit for the web. This library is very optimized and popular for building interfaces that are complex data-dense for desktop applications. TimezonePicker Component provides a way for users to select from a list of timezones. There are different props available to design timezone picker component properly.
TimezonePicker Props:
- buttonProps: It is used to denote the props to spread to the target Button.
- className: It is used to denote a space-delimited list of class names to pass along to a child element.
- date: It is used to denote the date to use when formatting timezone offsets.
- disabled: It is used to indicate whether this component is non-interactive.
- inputProps: It is used to denote the props to spread to the filter InputGroup.
- onChange: It is a callback function that is triggered when the user selects a timezone.
- placeholder: It is used to denote the text to show when no timezone has been selected.
- popoverProps: It is used to denote the props to spread to Popover.
- showLocalTimezone: It is used to indicate whether to show the local timezone at the top of the list of initial timezone suggestions.
- value: It is used to denote the currently selected timezone UTC identifier.
- valueDisplayFormat: It is used for the formatting display date and used it when displaying the selected (or default) timezone within the target element.
Approach: Let us create a React project and install React Blueprint module. Then we will create a UI that will showcase React.js BluePrint TimezonePicker Component Props.
Creating React Project:
Step 1: To create a react app, you need to install react modules through npx command. “npx” is used instead of “npm” because you will be needing this command in your app’s lifecycle only once.
npx create-react-app project_name
Step 2: After creating your react project, move into the folder to perform different operations.
cd project_name
Step 3: After creating the ReactJS application, Install the required module using the following command:
npm install @blueprintjs/core
npm install --save @blueprintjs/timezone
Project Structure: After running the commands mentioned in the above steps, if you open the project in an editor you can see a similar project structure as shown below. The new component user makes or the code changes, we will be performing will be done in the source folder.
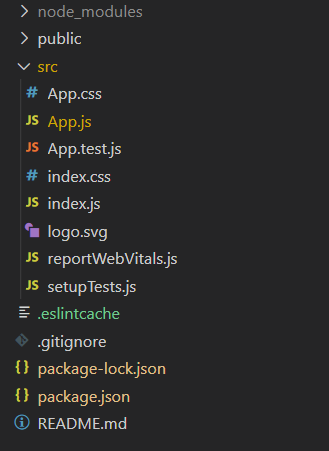
Project Structure
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Example 1: We are creating a UI that shows different React BluePrint TimezonePicker Component Props.
App.js
import React from "react" ;
import "@blueprintjs/core/lib/css/blueprint.css" ;
import { TimezonePicker } from "@blueprintjs/timezone" ;
export default function App() {
const [TimeZone, setTimeZone] = React.useState( "" );
return (
<div style={{ margin: 100, height: 500 }}>
<h1 style={{ color: "green" }}>
GeeksforGeeks
</h1>
<h3>React.js Blueprint TimezonePicker Props</h3>
<br />
<p>Display Format : Name</p>
<TimezonePicker value={TimeZone}
onChange={setTimeZone}
valueDisplayFormat= "name" />
<br /><br />
<p>Disabled Timezone Picker</p>
<TimezonePicker disabled />
</div>
);
}
|
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
Example 2: We are creating a UI that shows React BluePrint TimezonePicker Component Props.
App.js
import React from "react" ;
import "@blueprintjs/core/lib/css/blueprint.css" ;
import { TimezonePicker } from "@blueprintjs/timezone" ;
export default function App() {
const [TimeZone, setTimeZone] = React.useState( "" );
return (
<div style={{ margin: 100, height: 500 }}>
<h1 style={{ color: "green" }}>
GeeksforGeeks
</h1>
<h3>React .js Blueprint TimezonePicker Props</h3>
<br />
<p>Display Format : Name</p>
<TimezonePicker value={TimeZone}
onChange={setTimeZone}
valueDisplayFormat= "name" />
<br /><br />
<p>Display Format : Composite</p>
<TimezonePicker value={TimeZone}
onChange={setTimeZone}
valueDisplayFormat= "composite" />
<br /><br />
<p>Display Format : Abbreviation</p>
<TimezonePicker value={TimeZone}
onChange={setTimeZone}
valueDisplayFormat= "abbreviation" />
<br /><br />
<p>Display Format : Offset</p>
<TimezonePicker value={TimeZone}
onChange={setTimeZone}
valueDisplayFormat= "offset" />
<br /><br />
</div>
);
}
|
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
Reference: https://blueprintjs.com/docs/#timezone/timezone-picker.props
Share your thoughts in the comments
Please Login to comment...