React Fragments: Eliminating Unnecessary Divs in the DOM
Last Updated :
18 Mar, 2024
React Fragments function as wrapper elements that help us to group multiple components without using any additional DOM node. Fragments in React avoid unnecessary div tags or containers which helps in maintaining an efficient DOM structure.
What are React Fragments?
React Fragments are placeholders in React that let you group multiple components and JSX elements without adding extra unnecessary <div>
elements to the DOM. Instead of always wrapping your components in a <div>
structure, Fragments allow you to return multiple elements directly without an additional container. This helps in optimizing rendering performance and keeping the DOM clean and efficient.
To setup a basic React Application, refer to the Folder Structure for a React JS Project article
Example : This example demonstrates the implementation of React Fragments.
Javascript
// App.js
import React from "react";
function FirstComponent() {
return <h1>I'm from First Component</h1>
}
function SecondComponent() {
return <h1>I'm from Second Component</h1>
}
function ThirdComponent() {
return <h1>I'm from third Component</h1>
}
function App() {
return (
<React.Fragment>
<FirstComponent />
<SecondComponent />
<ThirdComponent />
</ React.Fragment>
);
}
export default App;
Output :
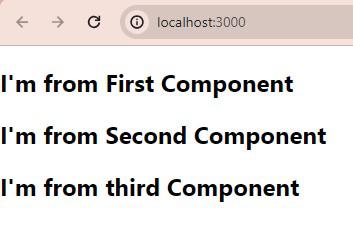
Shorthand Syntax for Fragments
React has a shorthand syntax to represent React fragments, that is by replacing “<React.fragment>” with empty angular brackets
“<>…</>” .
Note: Shorthand syntax cannot accept keys or attributes.
Javascript
// App.js
import React from "react";
function App() {
return (
<>
<FirstComponent />
<SecondComponent />
<ThirdComponent />
</>
);
}
export default App;
React Fragments and their impact on DOM structure
React Fragments helps in avoiding unecessary div’s in the DOM. These unecessary div’s leads to enlarged DOM tree that creates a negative impact on the user experience and on the rendering speed of a page. Fragments has no visual representation on DOM, unlike “div” tags are represented by a block.
A “div” tag returns multiple React elements by creating a block consisting of those elements, In contrast fragements directly returns those elements without creating an extra block for that elements. Fragments are used when there’s a need for grouping multiple elements without creating a block around them.Now let us see how the div tag and fragments are actual represented in source code.
Using div tag :
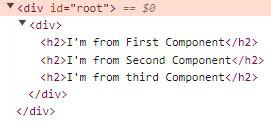
Using React Fragments :
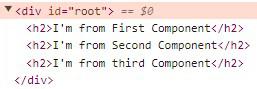
React Fragments vs Regular HTML Elements
React Fragments : React Fragments allow you to return multiple components without the involvement of an additonal DOM node (“div “). Fragments have the feature of passing keys and attributes, but it is not possible to use any styling property with fragments. Fragments consume less memory compared to regular HTML elements, it helps in improvising the rendering speed and also in maintaining a proper DOM structure.
Regular HTML Elements : Regular HTML elements are useful in cases such as applying the same property across multiple elements and adding css styles to the HTML elements. They also allow you to change the properties of HTML elements dynamically, whereas all these features are not available in React fragments.
Using React Fragments :
Javascript
// App.js
import React from "react";
function App() {
return (
<React.Fragment>
<h1>Done using Fragments</h1>
<p>This is a paragraph.</p>
</ React.Fragment>
);
}
export default App;
Output:
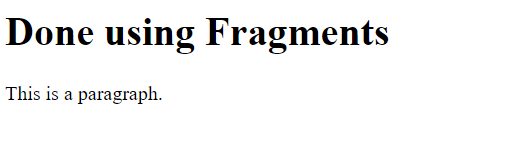
Using Regular HTML elements :
Javascript
// App.js
import React from "react";
function App() {
return (
<div style={{ backgroundColor: "red" }}>
<h1 style={{ color: "white" }}>
Done using Regular HTML elements
</h1>
<p style={{ color: "white" }}>
This is a paragraph.
</p>
</div>
);
}
export default App;
Output :
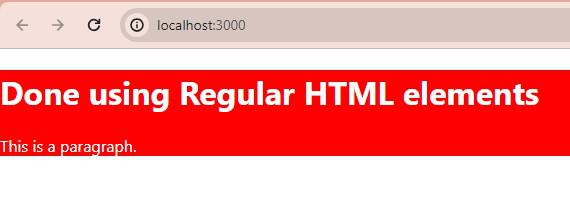
Using Regular HTML elements
Share your thoughts in the comments
Please Login to comment...