Python is known for its clean and readable syntax, which makes it an excellent language for beginners, but also powerful enough for advanced applications. In this article, we will learn about the basic elements of Python syntax.
Prerequisites: Before diving into Python syntax, ensure you have:
- Install Python (preferably Python 3.x).
- A text editor or IDE (Integrated Development Environment) like VSCode, PyCharm, or even IDLE (Python’s built-in IDE).
What is Python Syntax?
Python syntax refers to the set of rules that defines the combinations of symbols that are considered to be correctly structured programs in the Python language. These rules make sure that programs written in Python should be structured and formatted, ensuring that the Python interpreter can understand and execute them correctly. Here are some aspects of Python syntax:
Running Basic Python Program
There are different ways of running Python programs. Below are some most widely used and common ways to run the Python program.
Python Hello World
This is the simple printing of hello world program in Python.
Python3
print("Hello, World!")
Output:
Hello, World!
Python Interactive Shell
- Start the Python interpreter by simply typing python (or python3 on some systems) in the terminal or command prompt. We can then run Python commands interactively.
- To exit, we can type exit() or press Ctrl + D.
Example of Python Interactive Shell
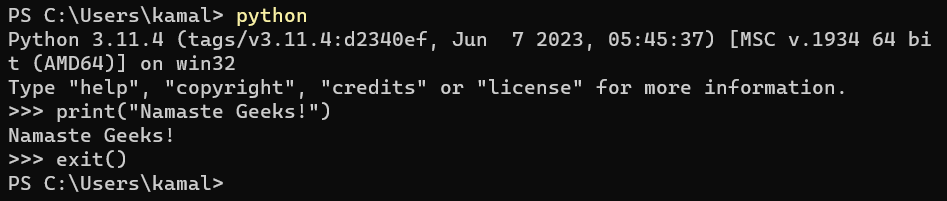
Running a Python Script
Create a script with the .py extension, e.g., Namaste.py.
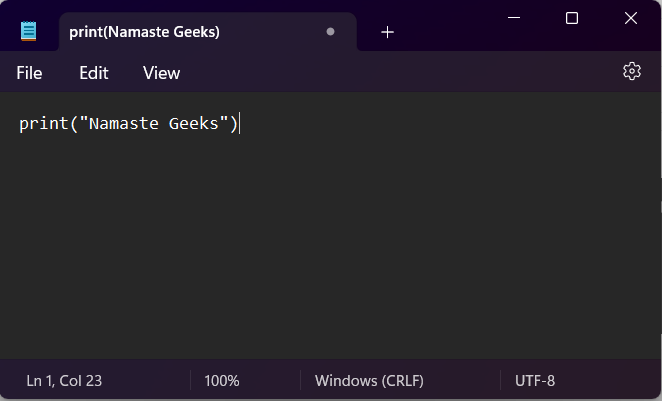
Run it from the terminal or command prompt using: python script.py (or python Namaste.py).

Indentation in Python
Python Indentation refers to the use of whitespace (spaces or tabs) at the beginning of a line of code in Python. It is used to define the code blocks. Indentation is crucial in Python because, unlike many other programming languages that use braces “{}” to define blocks, Python uses indentation. It improves the readability of Python code, but on other hand it became difficult to rectify indentation errors. Even one extra or less space can leads to identation error.
Python3
if 10 > 5:
print("This is true!")
if 10 > 5:
print("This is true!")
Python Variables
Variables in Python are essentially named references pointing to objects in memory. Unlike some other languages, in Python, you don’t need to declare a variable’s type explicitly. Based on the value assigned, Python will dynamically determine the type. In the below example, we create a variable ‘a’ and initialize it with interger value so, type of ‘a’ is int then we store the string value in ‘a’ it become ‘str’ type. It is called dynamic typing which means variable’s data type can change during runtime.
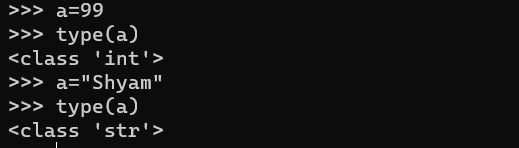
Python Identifiers
In Python, identifiers are the building blocks of a program. Identifiers are unique names that are assigned to variables, functions, classes, and other entities. They are used to uniquely identify the entity within the program. They should start with a letter (a-z, A-Z) or an underscore “_” and can be followed by letters, numbers, or underscores. In the below example “first_name” is an identifier that store string value.
first_name = "Ram"
For naming of an identifier we have to follows some rules given below:
- Identifiers can be composed of alphabets (either uppercase or lowercase), numbers (0-9), and the underscore character (_). They shouldn’t include any special characters or spaces.
- The starting character of an identifier must be an alphabet or an underscore.
- Certain words in Python, known as reserved Identifier, have specific functions and meanings. As such, these words can’t be chosen as identifiers. For instance, the term “print” already serves a specific purpose in Python and can’t be used as an identifier.
- Within its specific scope or namespace, each identifier should have a distinct name.
Python keywords
Keywords in Python are reserved words that have special meanings. For example if, else, while, etc. They cannot be used as identifiers. Below is the list of keywords in Python.
False await else import pass
None break except in raise
True class finally is return
and continue for lambda try
as def from nonlocal while
assert del global not with
async elif if or yield
With different versions of Python some keywords may vary. We can see all the keywords of current version of Python using below code.
>>>import keyword
>>>print(keyword.kwlist)

Comments in Python are statements written within the code. They are meant to explain, clarify, or give context about specific parts of the code. The purpose of comments is to explain the working of a code, they have no impact on the execution or outcome of a program.
Python Single Line Comment
Single line comments are preceded by the “#” symbol. Everything after this symbol on the same line is considered a comment and not considered as the part of execution of code. Below is the example of single line comment and we can see that there is no effect of comment on output.
Python3
first_name = "Reddy"
last_name = "Anna"
# print full name
print(first_name, last_name)
Python Multi-line Comment
Python doesn’t have a specific syntax for multi-line comments. However, programmers often use multiple single-line comments, one after the other, or sometimes triple quotes (either ”’ or “””), even though they’re technically string literals. Below is the example of multiline comment.
Python3
# This is a Python program
# to explain multiline comment.
'''
Functions to print table of
any number.
'''
def print_table(n):
for i in range(1,11):
print(i*n)
print_table(4)
Output4
8
12
16
20
24
28
32
36
40
Multiple Line Statements
Writing a long statement in a code is not feasible or readable. Writing a long single line statement in multiple lines by breaking it is more readable so, we have this feature in Python and we can break long statement into different ways such as:
Using Backslashes (\)
In Python, you can break a statement into multiple lines using the backslash (\). This method is useful, especially when we are working with strings or mathematical operations.
Python3
sentence = "This is a very long sentence that we want to " \
"split over multiple lines for better readability."
print(sentence)
# For mathematical operations
total = 1 + 2 + 3 + \
4 + 5 + 6 + \
7 + 8 + 9
print(total)
OutputThis is a very long sentence that we want to split over multiple lines for better readability.
45
Using Parentheses
For certain constructs, like lists, tuples, or function arguments, we can split statements over multiple lines inside the parentheses, without needing backslashes.
Python3
# Create list
numbers = [1, 2, 3,
4, 5, 6,
7, 8, 9]
def total(num1, num2, num3, num4):
print(num1+num2+num3+num4)
# Function call
total(23, 34,
22, 21)
Triple Quotes for Strings
If we are working with docstrings or multiline strings, we can use triple quotes (single ”’ or double “””).
Python3
text = """GeeksforGeeks Interactive Live and Self-Paced
Courses to help you enhance your programming.
Practice problems and learn with live and online
recorded classes with GFG courses. Offline Programs."""
print(text)
OutputGeeksforGeeks Interactive Live and Self-Paced
Courses to help you enhance your programming.
Practice problems and learn with live and online
recorded classes with GFG courses. Offline Programs.
Quotation in Python
In Python, strings can be enclosed using single (‘), double (“), or triple (”’ or “””) quotes. Single and double quotes are interchangeable for defining simple strings, while triple quotes allow for the creation of multiline strings. That we have used in above example. The choice of quotation type can simplify inserting one type of quote within a string without the need for escaping, for example, using double quotes to enclose a string that contains a single quote. Below is the example of using single and double quotes.
Python3
# Embedded single quote inside double.
text1 = "He said, 'I learned Python from GeeksforGeeks'"
# Embedded double quote inside single.
text2 = 'He said, "I have created a project using Python"'
print(text1)
print(text2)
OutputHe said, 'I learned Python from GeeksforGeeks'
He said, "I have created a project using Python"
Continuation of Statements in Python
In Python, statements are typically written on a single line. However, there are scenarios where writing a statement on multiple lines can improve readability or is required due to the length of the statement. This continuation of statements over multiple lines is supported in Python in various ways:
Implicit Continuation
Python implicitly supports line continuation within parentheses (), square brackets [], and curly braces {}. This is often used in defining multi-line lists, tuples, dictionaries, or function arguments.
Python3
# Line continuation within square brackets '[]'
numbers = [
1, 2, 3,
4, 5, 6,
7, 8, 9
]
# Line continuation within parentheses '()'
result = max(
10, 20,
30, 40
)
# Line continuation within curly braces '{}'
dictionary = {
"name": "Alice",
"age": 25,
"address": "123 Wonderland"
}
print(numbers)
print(result)
print(dictionary)
Output[1, 2, 3, 4, 5, 6, 7, 8, 9]
40
{'name': 'Alice', 'age': 25, 'address': '123 Wonderland'}
Explicit Continuation
If you’re not inside any of the above constructs, you can use the backslash ‘\’ to indicate that a statement should continue on the next line. This is known as explicit line continuation.
Python3
# Explicit continuation
s = "GFG is computer science portal " \
"that is used by geeks."
print(s)
OutputGFG is computer science portal that that is used by geeks.
Note: Using a backslash does have some pitfalls, such as if there’s a space after the backslash, it will result in a syntax error.
Strings
Strings can be continued over multiple lines using triple quotes (”’ or “””). Additionally, if two string literals are adjacent to each other, Python will automatically concatenate them.
Python3
text = '''A Geek can help other
Geek by writing article on GFG'''
message = "Hello, " "Geeks!"
print(text)
print(message)
OutputA Geek can help other
Geek by writing article on GFG
Hello, Geeks!
String Literals in Python
String literals in Python are sequences of characters used to represent textual data. Here’s a detailed look at string literals in Python. String literals can be enclosed in single quotes (‘), double quotes (“), or triple quotes (”’ or “””).
Python3
string1 = 'Hello, Geeks'
string2 = "Namaste, Geeks"
multi_line_string = '''Ram learned Python
by reading tutorial on
GeeksforGeeks'''
print(string1)
print(string2)
print(multi_line_string)
OutputHello, Geeks
Namaste, Geeks
Ram learned Python
by reading tutorial on
GeeksforGeeks
Command Line Arguments
In Python, command-line arguments are used to provide inputs to a script at runtime from the command line. In other words, command line arguments are the arguments that are given after the name of program in the command line shell of operating system. Using Python we can deal with these type of argument in various ways. Below are the most common ways:
Example: In this example we write the program to sum up all the numbers provided in command line. Firstly we import sys module. After that we checks if there are any numbers passed as arguments. Iterates over the command-line arguments, skipping the script name i.e. “sys.argv[0]” and then converts each argument to a float and then add them. We have used try-except block to handle the “ValueError”.
Python3
import sys
# Check if at least one number is provided
if len(sys.argv) < 2:
print("Please provide numbers as arguments to sum.")
sys.exit(1) # Exit with an error status code
try:
# Convert arguments to floats and sum them
total = sum(map(float, sys.argv[1:]))
print(f"Sum: {total}")
except ValueError:
print("All arguments must be valid numbers.")
OutputPlease provide numbers as arguments to sum.
Output: In the output we can see that the program returns the total of numbers passed as an arguments.
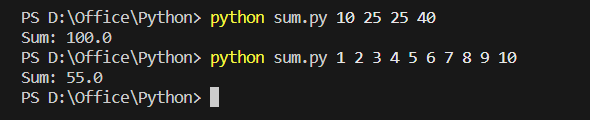
The input() function in Python is used to take user input from the console. The program execution halts until the user provides input and presses “Enter”. The entered data is then returned as a string. We can also provide an optional prompt as an argument to guide the user on what to input.
Example: In this example, the user will see the message “Please enter your name: “. After entering their name and pressing “Enter”, they’ll receive a greeting with the name they provided.
Python3
# Taking input from the user
name = input("Please enter your name: ")
# Print the input
print(f"Hello, {name}!")
Output:
Please enter your name:
Sagar
Hello, Sagar!
Share your thoughts in the comments
Please Login to comment...