Python Program to find if a character is vowel or Consonant
Last Updated :
17 Aug, 2023
Given a character, check if it is vowel or consonant. Vowels are ‘a’, ‘e’, ‘i’, ‘o’ and ‘u’. All other characters (‘b’, ‘c’, ‘d’, ‘f’ ….) are consonants.
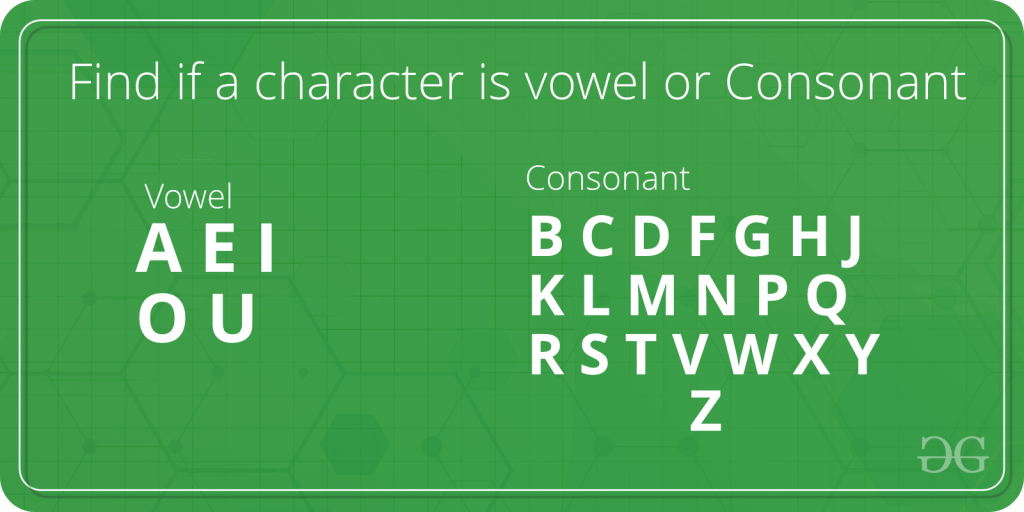
Examples:
Input : x = 'c'
Output : Consonant
Input : x = 'u'
Output : Vowel
We check whether the given character matches any of the 5 vowels. If yes, we print “Vowel”, else we print “Consonant”.
Python3
def vowelOrConsonant(x):
if (x = = 'a' or x = = 'e' or
x = = 'i' or x = = 'o' or x = = 'u' ):
print ( "Vowel" )
else :
print ( "Consonant" )
vowelOrConsonant( 'c' )
vowelOrConsonant( 'e' )
|
Time Complexity: O(1)
Auxiliary Space: O(1)
How to handle capital letters as well?
Python3
def vowelOrConsonant(x):
if (x = = 'a' or x = = 'e' or x = = 'i' or
x = = 'o' or x = = 'u' or x = = 'A' or
x = = 'E' or x = = 'I' or x = = 'O' or
x = = 'U' ):
print ( "Vowel" )
else :
print ( "Consonant" )
if __name__ = = '__main__' :
vowelOrConsonant( 'c' )
vowelOrConsonant( 'E' )
|
Time Complexity: O(1)
Auxiliary Space: O(1)
Python Program to find if a character is vowel or Consonant using switch case
Python3
def isVowel(ch):
switcher = {
'a' : "Vowel" ,
'e' : "Vowel" ,
'i' : "Vowel" ,
'o' : "Vowel" ,
'u' : "Vowel" ,
'A' : "Vowel" ,
'E' : "Vowel" ,
'I' : "Vowel" ,
'O' : "Vowel" ,
'U' : "Vowel"
}
return switcher.get(ch, "Consonant" )
print ( 'a is ' + isVowel( 'a' ))
print ( 'x is ' + isVowel( 'x' ))
|
Output
a is Vowel
x is Consonant
Time Complexity: O(1)
Auxiliary Space: O(1)
Another way is to find() the character in a string containing only Vowels.
Python3
def isVowel(ch):
str = "aeiouAEIOU"
return ( str .find(ch) ! = - 1 )
print ( 'a is ' + str (isVowel( 'a' )))
print ( 'x is ' + str (isVowel( 'x' )))
|
Output
a is True
x is False
Time Complexity: O(N)
Auxiliary Space: O(1)
Most efficient way to check Vowel using bit shift :
In ASCII these are the respective values of every vowel both in lower and upper cases.
Vowel |
DEC |
HEX |
BINARY |
a
|
97 |
0x61 |
01100001 |
e
|
101 |
0x65 |
01100101 |
i
|
105 |
0x69 |
01101001 |
o
|
111 |
0x6F |
01101111 |
u
|
117 |
0x75 |
01110101 |
|
A
|
65 |
0x41 |
01000001 |
E
|
69 |
0x45 |
01000101 |
I
|
73 |
0x49 |
01001001 |
O
|
79 |
0x4F |
01001111 |
U
|
85 |
0x55 |
01010101 |
As very lower and upper case vowels have the same 5 LSBs. We need a number 0x208222 which gives 1 in its LSB after right-shift 1, 5, 19, 15 otherwise gives 0. The numbers depend on character encoding.
DEC |
HEX |
BINARY |
31 |
0x1F |
00011111 |
2130466 |
0x208222 |
1000001000001000100010 |
Python3
def isVowel(ch):
return ( 0x208222 >> ( ord (ch) & 0x1f )) & 1
print ( 'a is ' + str (isVowel( 'a' )))
print ( 'x is ' + str (isVowel( 'x' )))
|
Time Complexity: O(1)
Auxiliary Space: O(1)
*We can omit the ( ch & 0x1f ) part on X86 machines as the result of SHR/SAR (which is >> ) masked to 0x1f automatically.
*For machines bitmap check is faster than table check, but if the ch variable stored in register than it may perform faster.
Use regular expressions:
Using a regular expression to check if a character is a vowel or consonant. Regular expressions are a powerful tool for pattern matching and can be used to quickly and easily check if a given character matches a specific pattern.
To check if a character is a vowel using a regular expression, you can use the following code:
Python3
import re
def is_vowel(char):
if re.match(r '[aeiouAEIOU]' , char):
return True
return False
print (is_vowel( 'a' ))
print (is_vowel( 'b' ))
|
Time complexity: O(1), as the re.match function has a time complexity of O(1) for small strings. In this case, the string char is a single character and therefore has a small constant size.
Auxiliary space: O(1), as we are only using a constant amount of memory for the char argument and the return value.
The regular expression r'[aeiouAEIOU]’ matches any character that is a lowercase or uppercase vowel. The re.match() function searches for a match at the beginning of the string, so it will return True if the given character is a vowel and False otherwise.
This approach has the advantage of being concise and easy to understand, and it can be easily modified to check for other patterns as well. However, it may not be as efficient as some of the other approaches mentioned in the article, particularly for large inputs.
Please refer complete article on Program to find if a character is vowel or Consonant for more details!
Using ord() method:
Python3
la = [ 97 , 101 , 105 , 111 , 117 ]
ua = [ 65 , 69 , 73 , 79 , 85 ]
def isVowel(ch):
if ord (ch) in la or ord (ch) in ua:
return True
return False
print ( 'a is ' + str (isVowel( 'a' )))
print ( 'x is ' + str (isVowel( 'x' )))
|
Output
a is True
x is False
Time Complexity: O(1)
Auxiliary Space: O(1)
Using operator.countOf() method
Python3
import operator as op
vowels = "aeiouAEIOU"
def is_vowel(char):
if op.countOf(vowels, char)> 0 :
return True
return False
print (is_vowel( 'a' ))
print (is_vowel( 'b' ))
|
Time Complexity: O(n)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...