Python | Convert LaTeX Matrices into SymPy Matrices using Python
Last Updated :
25 Sep, 2023
A matrix is a rectangular two-dimensional array of data like numbers, characters, symbols, etc. which we can store in row and column format. We can perform operations on elements by using an index (i,j) where ‘i’ and ‘j’ stand for an index of row and column respectively.
In the Python programming language, we can represent them in many formats like LaTeX matrices, Numpy arrays, Python 2D lists, Sympy Matrix, etc. From this, we will be using LaTeX Matrix to convert it into Sympy Matrix without using any in-built functions like latex2sympy2 and sympy because sometimes this won’t work properly for some cases like fractions and symbols. In this article, we’ll explore how to convert LaTeX matrices into SymPy matrices using Python.
What is LaTeX Matrices?
LaTeX has the ability to write matrices in many formats like matrix (Like arrays), pmatrix (Parentheses), bmatrix [Square brackets], Bmatrix {Curly Brackets}, etc. but we will be using normal arrays like a matrix for conversion as given below.
Note: You can also use different formats of matrices by making a few changes in regex and input.
Syntax Examples
![Rendered by QuickLaTeX.com \left[\begin{matrix} \frac{1}{2} & -8 & 5 \\ 2.3 & -\frac{9}{5} & 0 \\ -4 & -6.2 & 99 \end{matrix}\right]](https://www.geeksforgeeks.org/wp-content/ql-cache/quicklatex.com-4839eea6a5a7a194bf6b3b64ceff390d_l3.png)
![Rendered by QuickLaTeX.com \left[\begin{matrix} 1 & 2 \\ 3 & 4 \end{matrix}\right]](https://www.geeksforgeeks.org/wp-content/ql-cache/quicklatex.com-495081e5b58032eac68ae06af83ff6fc_l3.png)
![Rendered by QuickLaTeX.com \left[\begin{matrix} a & b & c \\ d & e & f \\ g & h & i \end{matrix}\right]](https://www.geeksforgeeks.org/wp-content/ql-cache/quicklatex.com-4d062b62494b66a142f4213b2f0722c2_l3.png)
What is SymPy Matrices
Sympy is a Python library that allows us to perform mathematical operations over matrices, algebraic expressions, trigonometric equations, calculus equations, and many more. Our goal is to convert the LaTeX matrix into a Sympy matrix. Sympy matrices are outputs of converted LaTeX matrix which we discussed in the above section.
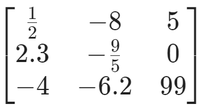
3×3 Number Matrix
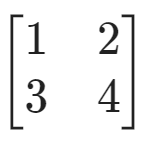
2×2 Number Matrix
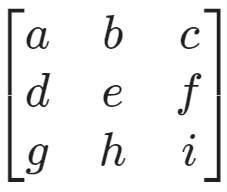
-200.png)
3×3 Matrix
Converting LaTeX to SymPy
Dowload Sympy library: We need to download sympy library to convert our elements into sympy objects. Just head over to your terminal, type following command and hit ENTER.
pip install sympy
Algorithm
- Import re, it is used for data extraction. Here we will extract elements from input i.e latex matrix.
- From sympy import Matrix and parse_latex. Matrix is used to create sympy matrix from python 2D-lists and parse_latex is used to convert python objects into sympy objects.
- from pprint import pprint, which will help us to read our output in terminal clearly and well formated.
Note: This will print our input in pythonic way, we can also print it in sympy object format using pprint from sympy module. - Extract data (rows and columns) from a latex matrix using search() function in re and using appropriate regular expression.
- Split this extracted data using the “\\\\” delimiter in split() function and store it into rows i.e 2D List.
- Initalize empty list to store elements present in latex matrix.
- Traverse each row from rows list and split this row into list of elements using the “&” delimiter.
- Append each row of elements into python matrix i.e 2D List while traversing.
- Initalize empty list to store sympy matrix.
- Iterate over the list of elements referred to as python_matrix.
- Using list comprehension transform each elements (Python Objects) into Sympy objects using the parse_latex function.
- Store above list of sympy ojects in a list named sympy_row.
- Once the first iteration is completed, append the sympy_row list to another list named sympy_matrix.
- Convert sympy_matrix i.e python 2D-list into a Sympy object using the Matrix() function from the Sympy library.
- Return this output which will return us the sympy matrix.
- Print this output using pprint.
Code Implemenation
Below function converts latex matrix into sympy matrix which take input in string format and give output in sympy matrix.
Python3
import re
from sympy import Matrix
from pprint import pprint
from sympy.parsing.latex import parse_latex
def latex_to_matrix(latex_matrix: str ) - > Matrix():
pattern = r "\\left\[\\begin\{matrix\}(.*?)\\end\{matrix\}\\right\]"
data = re.search(pattern, latex_matrix)[ 1 ]
rows = data.split( "\\\\" )
python_matrix = []
for row in rows:
elements_list = row.split( "&" )
python_matrix.append(elements_list)
sympy_matrix = []
for row in python_matrix:
sympy_row = [parse_latex(element) for element in row]
sympy_matrix.append(sympy_row)
return Matrix(sympy_matrix)
input = r "\left[\begin{matrix}1 & \sqrt[4]{9} & \frac{\frac{11}{2}}{5} \\ -\frac{2}{5} & -1.8 & 2^{4} \\ g & -100 & i\end{matrix}\right]"
pprint(latex_to_matrix( input ))
|
Output
Matrix([
[ 1, 9**(1/4), (11/2)/5],
[-2/5, -1.8, 2**4],
[ g, -100, i]])
Test on Different Inputs
Example 1: 3×3 Matrix of Numbers (Integers, decimals, fractions).
Input:
![Rendered by QuickLaTeX.com \left[\begin{matrix}\frac{1}{2} & -8 & 5 \\ 2.3 & -\frac{9}{5} & 0 \\ -4 & -6.2 & 99\end{matrix}\right]](https://www.geeksforgeeks.org/wp-content/ql-cache/quicklatex.com-ed585b9376b5f3a761321ff7f1380874_l3.png)
Output:
Matrix([
[1/2, -8, 5],
[2.3, -9/5, 0],
[ -4, -6.2, 99]])
Example 2: 2×2 Matrix of Integers.
Input:
Output:
Matrix([
[1, 2],
[3, 4]])
Example 3: 3×3 Matrix of alphabets.
Input:
Output:
Matrix([
[a, b, c],
[d, e, f],
[g, h, i]])
Time Complexity :
O(m*n), where m and n represents rows and columns respectively.
Conclusion
Conversion of LaTeX matrices into Sympy matrices allows us to perform mathematical opeartions on them which we won’t be able to do it with LaTeX matrices. With the help of Sympy parse_latex we easily convert python objects into sympy objects and store them in python 2D-list and further convert it into sympy object which gives us sympy matrix.
Share your thoughts in the comments
Please Login to comment...