In this article, we will learn about how Python API is used to retrieve data from various sources. Also, we will cover all concepts related to Python API from basic to advanced. Various websites provide weather data, Twitter provides data for research purposes, and stock market websites provide data for share prices.
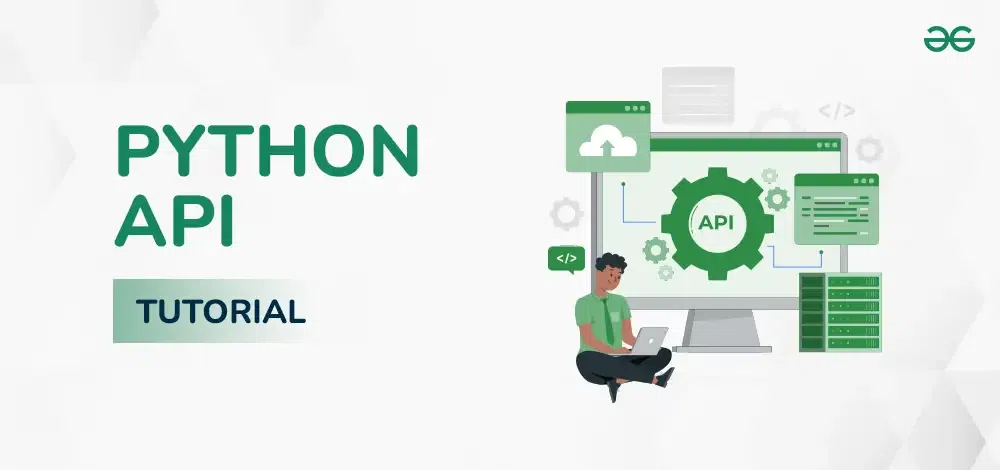
Python API Tutorial
What is an API?
API stands for “Application Programming Interface.” In simple terms, it’s a set of rules and protocols that allow how different software applications can communicate and interact with each other. APIs define the methods and data formats that applications can use to request and exchange information. To retrieve data from a web server, a client application initiates a request, and the server responds with the requested data. APIs facilitate this communication by serving as intermediaries, allowing seamless integration between diverse software systems. In essence, APIs act as bridges that enable the smooth exchange of data and functionality, enhancing interoperability across various applications.
Prerequisites: Python, API
Making API Requests in Python
In order to work with API some tools are required such as requests so we need to first install them in our system.
Command to install ‘requests’:
pip3 install requests
Once we have installed it, we need to import it in our code to use it.
Command to import ‘requests’:
import requests
Let us understand the working of API with examples. First let us take a simple example.
Example 1: Extracting stock price by the help of API
In this Python program fetches the live stock data for “IBM” from the Alpha Vantage API using the 5-minute interval and prints the opening price.Here we make use of ‘requests’ to make a call and it is checked with the help of status code that whether our request was successful or not.Then the response is converted to python dictionary and the respected data is stored .
Python3
import requests
import json
def get_stock_data():
response = requests.get(url)
if response.status_code = = 200 :
data = response.json()
last_refreshed = data[ "Meta Data" ][ "3. Last Refreshed" ]
price = data[ "Time Series (5min)" ][last_refreshed][ "1. open" ]
return price
else :
return None
stock_prices = {}
price = get_stock_data()
symbol = "IBM"
if price is not None :
stock_prices[symbol] = price
print (f "{symbol}: {price}" )
|
Output:

API
API Status Codes
As we know Status code tells us about what happened with our request, whether it was successfully executed or other was some error while processing it. They are returned with every request we place.
- 200 OK: The server successfully processed the request, and the requested data is returned.
- 201 Created: A new resource is created on the server as a result of the request.
- 204 No Content: The request is successful, but there is no additional data to return.
- 300 Multiple Choices: The requested resource has multiple representations, each with its own URL.
- 302 Found (Temporary Redirect): The requested resource is temporarily located at a different URL.
- 304 Not Modified: The client’s cached copy of the resource is still valid, and no re-download is necessary.
- 400 Bad Request: The request has malformed syntax or contains invalid data, making it incomprehensible to the server.
- 401 Unauthorized: Authentication is required, and the client’s credentials (e.g., API key) are missing or invalid.
- 500 Internal Server Error: An unexpected server error occurred during request processing.
- 502 Bad Gateway: Acting as a gateway or proxy, the server received an invalid response from an upstream server.
These status codes help communicate the outcome of API requests and guide developers and clients in understanding the results, errors, or necessary actions.
API Documentation
API Documentation is very essential and it helps in effective interaction. Here, we are using NewsAPI that provides us the information regarding various news of various countries and celebrities. To get news updates from NewsAPI, we need a special key called an API key. Think of it as a digital passcode that lets us access their news database. We’ve stored this key in a place called API_KEY
.
Next, we’ve built a specific web address (or URL) that tells NewsAPI exactly what kind of news we want – in this case, top business headlines from the United States. It’s like telling a librarian you’re interested in the business section of a newspaper from a particular city.
After setting up this request, our code then sends a message to NewsAPI using this URL. It’s similar to clicking on a link to see a webpage. Once we send this message, NewsAPI replies with a status update. This status tells us if our request was successful or if there was any problem. We then simply print out this status to see if everything worked as expected.
Python3
import requests
API_KEY = '3805f6bbabcb42b3a0c08a489baf603d'
response = requests.get(url)
print (response.status_code)
|
Output
200
A successful request yields a ‘200’ status code, signifying success. The documentation also mentions that the API response comes in JSON format. Now, we will use response.json() method to get complete output in next section (Using API with Query).
Working with JSON Data
While working with APIs, it is very essential to know how to work with JSON data. Json universally works as the language of APIs that helps in providing a way to encode the data structures in a format that is easily interpreted by machines. Imagine browsing a news website. The data we see there—headlines, descriptions, images—is often structured in a format called JSON. It’s like the universal language that APIs speak.
Now, to make sense of this digital jigsaw puzzle, we’ve written a Python script. This script acts like a digital news curator: it reaches out to NewsAPI using a library called requests and fetches the latest business headlines from the US. Once it has this data, Python steps in, sorting and presenting it in a neat list. Think of it as a friendly librarian picking out the top three articles for us from a vast collection. This whole process not only gives us a glimpse into how APIs and JSON work hand in hand but also underscores the magic Python brings to the table in managing such tasks effortlessly.
Python3
import json
import requests
def fetch_and_print_articles(api_url):
response = requests.get(api_url)
if response.status_code = = 200 :
articles = response.json().get( 'articles' , [])
for index, article in enumerate (articles[: 3 ], start = 1 ):
print (f "Article {index}:\n{json.dumps(article, sort_keys=True, indent=4)}\n" )
else :
print (f "Error: {response.status_code}" )
API_KEY = '3805f6bbabcb42b3a0c08a489baf603d'
fetch_and_print_articles(api_endpoint)
def jprint(obj):
print (json.dumps(obj, sort_keys = True , indent = 4 ))
|
Output
Article 1:
{
“author”: “SamMobile, Asif Iqbal Shaik”,
“content”: “As tensions between China and the US escalate, the two countries are trying to counter each other through certain measures. The USA banned US-based chipmakers from exporting advanced chips and chip m\u2026 [+2001 chars]”,
“description”: “As tensions between China and the US escalate, the two countries are trying to counter each other through certain measures. …”,
“publishedAt”: “2023-12-18T09:07:00Z”,
“source”: {
“id”: null,
“name”: “SamMobile”
},
“title”: “China bans Apple and Samsung phones from government offices and firms – SamMobile – Samsung news”,
“url”: “https://www.sammobile.com/news/china-bans-apple-iphone-samsung-phones-government-offices-firms/”,
“urlToImage”: “https://www.sammobile.com/wp-content/uploads/2023/08/Samsung-Galaxy-Z-Flip-5-Fold-5-China-720×405.jpg”
}
Article 2:
{
“author”: “Katrina Bishop”,
“content”: “The 10-year Treasury note yield slipped further on Monday, as the final full trading week of 2023 gets underway.\r\nTraders continue to digest the unexpectedly dovish tone of the U.S. Federal Reserve l\u2026 [+1438 chars]”,
“description”: “The 10-year Treasury note yield continued to slip Monday as the final full trading week of 2023 gets underway.”,
“publishedAt”: “2023-12-18T08:04:15Z”,
“source”: {
“id”: null,
“name”: “CNBC”
},
“title”: “10-year Treasury yield slip further after Fed’s ‘big shift’ – CNBC”,
“url”: “https://www.cnbc.com/2023/12/18/10-year-treasury-yield-slip-further-after-feds-big-shift.html”,
“urlToImage”: “https://image.cnbcfm.com/api/v1/image/107347379-17025005642023-12-13t204555z_488020625_rc2jw4a133y9_rtrmadp_0_usa-stocks.jpeg?v=1702500622&w=1920&h=1080”
}
Article 3:
{
“author”: “Hakyung Kim”,
“content”: “U.S. stock futures climbed on Monday morning after the three major averages notched their seventh straight week of gains. The Dow Jones Industrial Average recorded a new intraday record, and the Nasd\u2026 [+1488 chars]”,
“description”: “The Dow Jones Industrial Average recorded a new intraday record on Friday, and the Nasdaq 100 had a new closing high.”,
“publishedAt”: “2023-12-18T05:43:00Z”,
“source”: {
“id”: null,
“name”: “CNBC”
},
“title”: “Stock futures are all rise after major averages rally for seven consecutive weeks: Live updates – CNBC”,
“url”: “https://www.cnbc.com/2023/12/17/stock-futures-are-little-changed-after-major-averages-rally-for-seven-consecutive-weeks-live-updates.html”,
“urlToImage”: “https://image.cnbcfm.com/api/v1/image/107347379-17025005642023-12-13t204555z_488020625_rc2jw4a133y9_rtrmadp_0_usa-stocks.jpeg?v=1702500622&w=1920&h=1080”
}
Using API with Query
When interacting with an API, especially one as popular as NewsAPI, it’s essential to know how to specify and tailor the data you want to retrieve. In this code snippet, we’re making use of the NewsAPI to fetch top headlines specifically for the United States. To communicate with the NewsAPI, we have the endpoint URL defined as API_URL
. Additionally, each developer or user is provided with an API key for authentication purposes. Here, the API key is stored in the API_KEY
variable.
However, simply knowing the endpoint and having an API key isn’t enough. Often, APIs allow for a level of customization, letting users specify certain parameters to refine their request. This is where the params
dictionary comes into play. In this case, we’re interested in fetching news from the U.S., so we set the country
parameter to “us”. Additionally, to ensure our request is authenticated and linked to our account, we include the API key in the parameters.
Python3
import requests
API_KEY = "3805f6bbabcb42b3a0c08a489baf603d"
params = {
"country" : "us" ,
"apiKey" : API_KEY
}
response = requests.get(API_URL, params = params)
if response.status_code = = 200 :
print (response.json())
else :
print (f "Error: {response.status_code}" )
|
Output
{
“status”: “ok”,
“totalResults”: 37,
“articles”: [
{
“source”: {
“id”: null,
“name”: “BBC News”
},
“author”: null,
“title”: “Iceland volcano erupts on Reykjanes peninsula – BBC.com”,
“description”: “The eruption, in south-west Iceland, led to half the sky being \”lit up in red\”, an eyewitness says.”,
“url”: “https://www.bbc.com/news/world-europe-67756413”,
“urlToImage”: “https://ichef.bbci.co.uk/news/1024/branded_news/13806/production/_132087897_helicopterstill.jpg”,
“publishedAt”: “2023-12-19T08:24:57Z”,
“content”: “By Marita Moloney & Oliver SlowBBC News\r\nSpectacular helicopter shots show the eruption on the island’s coast\r\nA volcano has erupted on the Reykjanes peninsula of south-west Iceland after weeks o… [+5343 chars]”
},
{
“source”: {
“id”: null,
“name”: “[Removed]”
},
“author”: null,
“title”: “[Removed]”,
“description”: “[Removed]”,
“url”: “https://removed.com”,
“urlToImage”: null,
“publishedAt”: “1970-01-01T00:00:00Z”,
“content”: “[Removed]”
},
{
“source”: {
“id”: “financial-times”,
“name”: “Financial Times”
},
“author”: null,
“title”: “Google to pay $700mn in antitrust settlement over Android app store – Financial Times”,
“description”: “Tech giant agrees to alternative billing methods for Play Store in ‘unprecedented’ case from US states”,
“url”: “https://www.ft.com/content/e7f7c7d6-79b4-4de4-aa4b-f656546a91ca”,
“urlToImage”: null,
“publishedAt”: “2023-12-19T06:41:18Z”,
“content”: “Keep abreast of significant corporate, financial and political developments around the world. Stay informed and spot emerging risks and opportunities with independent global reporting, expert comment… [+30 chars]”
}
]
}
Example 2: How To Track ISS (International Space Station) Using Python?
In this example we will discuss how to track the current location of ISS(International Space Station) and then maps the location.
Required Module
Python3
import json
import turtle
import urllib.request
import time
import webbrowser
import geocoder
|
Explanation of Library Usage:
- json: Handling JSON data received from the ISS API.
- turtle: Creating a simple map to display the ISS location.
- urllib.request: Fetching data from the ISS API.
- time: Adding time-related functionality, such as delays.
- webbrowser: Opening the map in a web browser.
- geocoder: Retrieving the latitude and longitude of locations.
Getting Started With API Key
So now there is a problem with tracking ISS because it travels at a speed of almost 28000km/h. Thus, it takes only 90 minutes to complete 1 rotation around the earth. At such a speed, it becomes quite difficult to lock the exact coordinates. So here comes the API to solve this issue. API acts as an intermediate between the website and the program, thus providing the current time data for the program.
In our case, API will provide us with the current location of ISS in earth’s orbit, so visit the link below as an API link for astronaut info.
url = "http://api.open-notify.org/astros.json"
Accessing Data
Use urllib.request.urlopen() function inorder to open the API url and json.loads(.read()) function to read the data from the URL.
Python3
import json
import turtle
import urllib.request
import time
import webbrowser
import geocoder
response = urllib.request.urlopen(url)
result = json.loads(response.read())
result
|
Output:
{"number": 10, "people": [{"craft": "ISS", "name": "Sergey Prokopyev"},
{"craft": "ISS", "name": "Dmitry Petelin"},
{"craft": "ISS", "name": "Frank Rubio"},
{"craft": "Tiangong", "name": "Jing Haiping"},
{"craft": "Tiangong", "name": "Gui Haichow"},
{"craft": "Tiangong", "name": "Zhu Yangzhu"},
{"craft": "ISS", "name": "Jasmin Moghbeli"},
{"craft": "ISS", "name": "Andreas Mogensen"},
{"craft": "ISS", "name": "Satoshi Furukawa"},
{"craft": "ISS", "name": "Konstantin Borisov"}], "message": "success"}
Create.txt file for astronauts info
Create iss.text file using an open() function in write mode and write the result(names & numbers of astronauts) as data inside the file.
Python3
file = open ( "iss.txt" , "w" )
file .write(
"There are currently " + str (result[ "number" ]) +
" astronauts on the ISS: \n\n" )
people = result[ "people" ]
for p in people:
file .write(p[ 'name' ] + " - on board" + "\n" )
|
Current Latitude and Longitude of User
Use geocoder.ip(‘me’) to know your current location in terms of latitude and longitude and after that using write the data in the file and then close the file using the file.close() function.
Python3
g = geocoder.ip( 'me' )
file .write( "\nYour current lat / long is: " + str (g.latlng))
file .close()
webbrowser. open ( "iss.txt" )
|
Setting Up The World Map
Use turtle.screen() function to get access to the screen, then use screen.setup() to set the size and position of the output window. Use screen.setworldcoordinates() function to set the coordinates of all 4 corners on x, y-axis so that when iss reach out from reaches they appear again from another edge.
Python3
screen = turtle.Screen()
screen.setup( 1280 , 720 )
screen.setworldcoordinates( - 180 , - 90 , 180 , 90 )
|
Set map as background pic using screen.bgpic() function and set iss image as turtle shape using screen.register_shape() function. Use it as an object and assign it as a shape using iss.shape() function, then set the angle of shape using iss.setheading() function. iss.penup() function indicates that their drawings. Thus, the turtle stops. The file can be downloaded:
Code:
Python3
screen.bgpic( "images\map.gif" )
screen.register_shape( "images\iss.gif" )
iss = turtle.Turtle()
iss.shape( "images\iss.gif" )
iss.setheading( 45 )
iss.penup()
|
Access the current status of ISS using the API below:
url = "http://api.open-notify.org/iss-now.json"
Extract the current location of ISS in terms of latitude and longitude from the above API. This script below runs inside the while loop so you can see the updated position and movement of the ISS until you stop the program.
Python3
response = urllib.request.urlopen(url)
result = json.loads(response.read())
location = result[ "iss_position" ]
lat = location[ 'latitude' ]
lon = location[ 'longitude' ]
lat = float (lat)
lon = float (lon)
print ( "\nLatitude: " + str (lat))
print ( "\nLongitude: " + str (lon))
|
Update the position of ISS every 5 seconds by refreshing the latitude and longitude value from API.
Python3
iss.goto(lon, lat)
time.sleep( 5 )
|
Below is the full implementation.
Python3
import json
import turtle
import urllib.request
import time
import webbrowser
import geocoder
response = urllib.request.urlopen(url)
result = json.loads(response.read())
file = open ( "iss.txt" , "w" )
file .write( "There are currently " +
str (result[ "number" ]) + " astronauts on the ISS: \n\n" )
people = result[ "people" ]
for p in people:
file .write(p[ 'name' ] + " - on board" + "\n" )
g = geocoder.ip( 'me' )
file .write( "\nYour current lat / long is: " + str (g.latlng))
file .close()
webbrowser. open ( "iss.txt" )
screen = turtle.Screen()
screen.setup( 1280 , 720 )
screen.setworldcoordinates( - 180 , - 90 , 180 , 90 )
screen.bgpic( "images/map.gif" )
screen.register_shape( "images\iss.gif" )
iss = turtle.Turtle()
iss.shape( "images\iss.gif" )
iss.setheading( 45 )
iss.penup()
while True :
response = urllib.request.urlopen(url)
result = json.loads(response.read())
location = result[ "iss_position" ]
lat = location[ 'latitude' ]
lon = location[ 'longitude' ]
lat = float (lat)
lon = float (lon)
print ( "\nLatitude: " + str (lat))
print ( "\nLongitude: " + str (lon))
iss.goto(lon, lat)
time.sleep( 5 )
|
Output:
Crew Information: Here is info on the onboarded crew members along with their names.
.png)
API
ISS Location: Here is a screenshot of the moving ISS i.e orbiting around the earth. You can see it by zooming in on the screenshot.
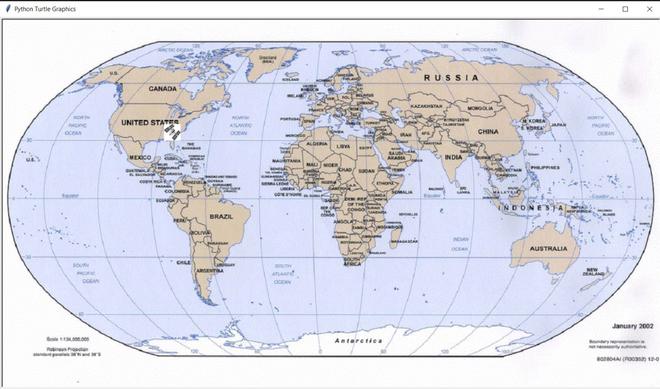
API
ISS Moving look: Here you can see the ISS moving every 5 seconds.
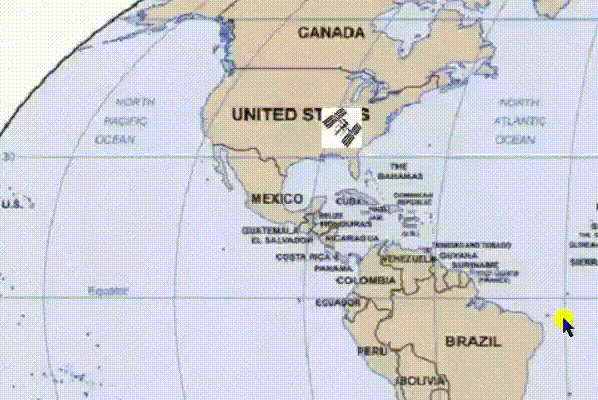
API
Reasons to use API
Here the question arises why use API? if we can get data in the a form of CSV file from the resource. To understand this let us look at the examples below:
- Change in Data: Suppose we are using API to collect data about the temperature of all the cities in India. As the temperature changes with time, a new CSV file is to be downloaded every time we want the information and it will require a lot of bandwidth and a lot of time to process the same. The same data can be collected with the API very easily and in less time.
- Size of data: At certain times we require only a small part of the large data. For example, if we want to get the comments on a tweet then we don’t need to download the whole dataset of Twitter. The required task can be done very easily with the help of Twitter API. APIs enable the extraction of specific, targeted data, minimizing unnecessary data transfer and optimizing the process for tasks that require only a fraction of the available information.
Conclusion
In conclusion , an API (Application Programming Inferface) serves a protocol and tools that enables different software applications to communicate and protocols interact with each other. APIs are instrumental in enabling the interoperability of applications, fostering innovation, and streamlining the development process by providing standardized interfaces for communication and data exchange.
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...