PyTest: Interactive Output instead of pure ASCII
Last Updated :
08 Feb, 2024
There are numerous benefits of testing the code. It assures that modifications to the code won’t result in regressions and boosts the confidence that the code acts as we anticipate. We should make the most of all the tools to make writing and maintaining tests as comfortable as we can because it is an exacting job. There are a number of different advantages of testing such as bug detection, functional verification, refactoring, maintenance, collaboration, documentation, and supporting CI/CD practices.
PyTest in Python
The PyTest is a third-party managed unit testing library developed for Python developers. It is an open-source tool with a good user base. All different types of testing are supported in the Pytest framework such as integration testing, end-to-end run testing, functional testing, etc.
Advantages of using Pytest
- It is a free and open-source framework.
- It is incredibly simple to start using because of its straightforward syntax.
- The execution time of the test suite is shortened by its ability to run several tests concurrently.
- It can detect the test file and test functions automatically.
- It allows you to run and skip a subset of the entire test suite.
The output for pytest if run without any added plugins gives a very raw output in ASCII format and mentions just the general information about unit tests. This pytest output can be enhanced and decorated with the use of multiple different plugins such as pytest-html and pytest-cov.
Pytest-html
Pytest-html plugin creates HTML reports for all the tests that we run. It offers a simple method for producing comprehensive reports with eye-catching visuals that list the test results. The simple ASCII format of pytest output is improved using this generated HTML file which includes details about the ran tests, their statuses, any collected output, and other pertinent information.
Pytest-cov
Pytest-cov plugin provides Python test suite code coverage analysis for pytest. It effortlessly integrates with Pytest and creates coverage reports, enabling you to evaluate the efficacy of your tests by identifying the sections of your code that are and are not covered.
How to Run PyTest in Python?
Here is a step-by-step guide to running PyTest in Python.
Step 1: The first step to use PyTest in Python is to install it on the system. We can do so by executing the following command in our terminal:
pip install pytest
Step 2: For this article, we will take a simple Python Program as an example. We will write 6 different basic maths functions for addition, multiplication, subtraction, and division. It also contains two geometry functions to calculate the area and perimeter of the rectangle. All the functions are very much self-explanatory. We will name this file “maths.py“.
Python3
def calc_addition(a, b):
return a + b
def calc_multiply(a, b):
return a * b
def calc_substraction(a, b):
return a - b
def calc_division(a, b):
return a / b
def area_of_rectangle(width, height):
area = width * height
return area
def perimeter_of_rectangle(width, height):
perimeter = 2 * (width + height)
return perimeter
|
Step 3: In order to test these functions, we will create a new Python file “test.py“, import our above maths functions file and write test cases for those functions. The pytest of division function is not added intentionally so that we can capture that part of code in the test coverage and then analyze the generated coverage report.
Python3
import maths
def test_calc_addition():
output = maths.calc_addition( 2 , 4 )
assert output = = 6
def test_calc_substraction():
output = maths.calc_substraction( 2 , 4 )
assert output = = - 2
def test_calc_multiply():
output = maths.calc_multiply( 2 , 4 )
assert output = = 8
def test_area():
output = maths.area_of_rectangle( 2 , 5 )
assert output = = 11
def test_perimeter():
output = maths.perimeter_of_rectangle( 2 , 5 )
assert output = = 14
|
Step 4: Now, we run Pytest on our Python program. To run Pytest, we need to run the following command.
pytest test.py
Output:
As we can see, the output that we have got for the given test file is very plain (pure ASCII) and not very easy to understand and debug-friendly. Hence, we use Pytest to generate interactive output.
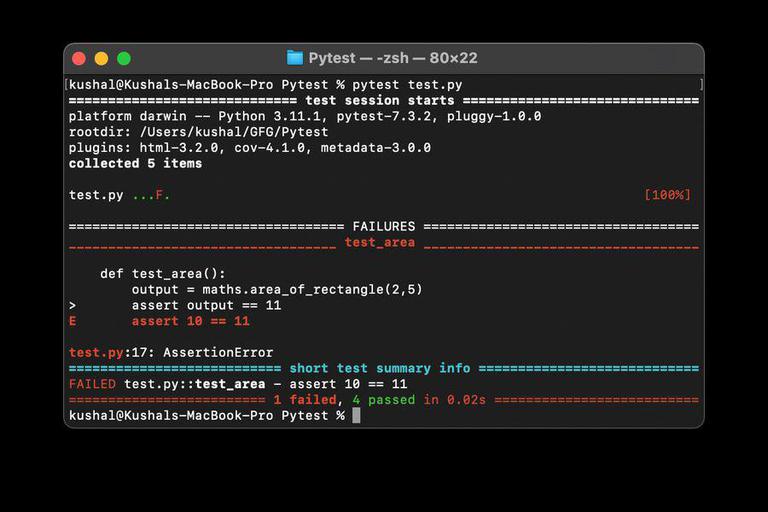
Command to run pytest
How to Generate Interactive Output Instead of Pure ASCII?
To enable interactive output instead of pure ASCII output in PyTest, we will use the pytest-html plugin along with the pytest-cov plugin. The pytest-html is a plugin for pytest which can generate an HTML report for the test results.
Generate Interactive Output using Pytest-html Plugin
Let us see the steps to generate an HTML report for test results using pytest-html.
Step 1: First, install the Pytest HTML plugin using the following command.
pip install pytest-html
Step 2: Add the following options to the existing pytest command.
- –showlocals: It will show local variables in traceback.
- –html=report.html: Running this command will generate a report.html file for the output of the tests.
- –self-contained-html – In order to respect the Content Security Policy (CSP), several assets such as CSS and images are stored separately by default. Passing this flag would create a self-contained report.
pytest --showlocals --html=report.html --self-contained-html test.py
Output:
Observe the HTML output of pytest tests generated in report.html file.
-1024.jpg)
Generate Interactive Output using Pytest-cov Plugin
Let us see the steps to generate a coverage report for test results using pytest-cov.
Step 1: First, install the pytest-cov plugin using the following command.
pip install pytest-cov
Step 2: Add the following options to the existing pytest command.
- –cov=PATH: Measure coverage for the filesystem path.
- –cov-report=html: To specify that the HTML report is to be generated.
pytest --showlocals --html=report.html --self-contained-html
--cov=maths --cov-report=html test.py
Output:
Observe the HTML output of pytest tests generated in htmlcov/index.html file.
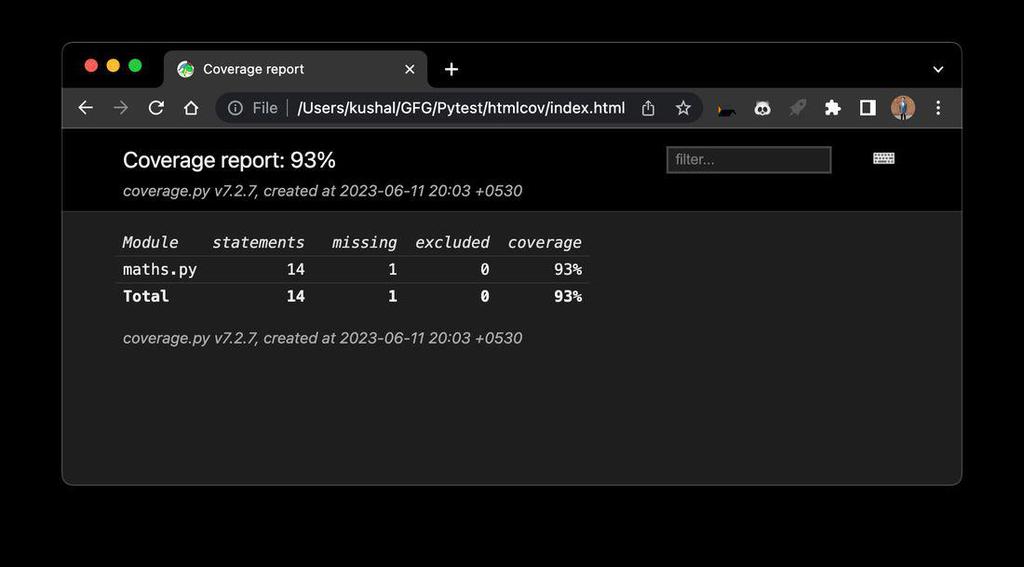
Coverage report for python tests
Coverage report per line will be displayed when we click on the module name in index.html
.jpg)
Coverage report per line
Share your thoughts in the comments
Please Login to comment...