Grouping the Tests in Pytest
Last Updated :
16 Oct, 2023
Testing is an essential part of software development, ensuring that your code works as expected and preventing regressions. Pytest is a popular testing framework for Python that makes writing and running tests easier and more efficient. In this article, we are going to look at ‘Grouping the tests in Pytest’.
PyTest – Grouping the Tests
Grouping in Pytest can be done in several ways. In this article, we are going to group the tests in Pytest using the following methods in Python:
- Making separate classes of the tests.
- Applying markers on the test.
- Making another test file.
Throughout this article, we are going to refer to the following module and test file.
Test File: mathfuncs.py
Python3
class Algebra:
def square(x):
return x * * 2
def cube(x):
return x * * 3
class Geometry:
def is_triangle(a, b, c):
return a + b + c = = 180
def is_quadrilateral(w, x, y, z):
return w + x + y + z = = 360
|
Test File: test_mathfuncs.py
Python3
import mathfuncs
def test_square():
assert mathfuncs.Algebra.square( 40 ) = = 1600
assert mathfuncs.Algebra.square( 5 ) = = 25
def test_cube():
assert mathfuncs.Algebra.cube( 40 ) = = 64000
assert mathfuncs.Algebra.cube( 5 ) = = 125
def test_is_triangle():
assert mathfuncs.Geometry.is_triangle( 120 , 40 , 20 ) = = True
assert mathfuncs.Geometry.is_triangle( 45 , 67 , 99 ) = = False
def test_is_quadrilateral():
assert mathfuncs.Geometry.is_quadrilateral( 350 , 5 , 5 , 0 ) = = True
assert mathfuncs.Geometry.is_quadrilateral( 11 , 22 , 33 , 44 ) = = False
|
Making Separate Classes of the Tests
To group the tests, we can make separate classes of the tests and then select the class and run it, so that only the tests inside that class are run. In the above example, in the “mathfuncs” module, we have 4 functions, of which two of them belong to the ‘Algebra’ class and rest of them belong to the ‘Geometry’ class. However, in test file, we have not made any classes. So running the test file results in testing all the four test functions. To group these test functions, we can make separate classes as shown below:
Python3
import mathfuncs
class Test_Algebra:
def test_square( self ):
assert mathfuncs.Algebra.square( 40 ) = = 1600
assert mathfuncs.Algebra.square( 5 ) = = 25
def test_cube( self ):
assert mathfuncs.Algebra.cube( 40 ) = = 64000
assert mathfuncs.Algebra.cube( 5 ) = = 125
class Test_Geometry:
def test_is_triangle( self ):
assert mathfuncs.Geometry.is_triangle( 120 , 40 , 20 ) = = True
assert mathfuncs.Geometry.is_triangle( 45 , 67 , 99 ) = = False
def test_is_quadrilateral( self ):
assert mathfuncs.Geometry.is_quadrilateral( 350 , 5 , 5 , 0 ) = = True
assert mathfuncs.Geometry.is_quadrilateral( 11 , 22 , 33 , 44 ) = = False
|
Now to run just one of the test class, we write the following command:
pytest test_file.py::test_class -v
For example, if we have to run only the test functions inside the ‘Test_Algebra’ class, we would write the following command:
pytest test_mathfuncs.py::Test_Algebra -v
Output

Test Results
Above, we can see that only two items were collected. That means, we just ran the tests inside the Test_Algebra class. In this way, we can group the tests in Pytest by creating separate test classes.
Applying Markers on the Test
To group the tests, we can apply markers on the test functions that we want to group. These markers will be custom, i.e., we will create them. These markers will offer no functionality. We will use them just to group the tests and run them.
To apply the markers on the test functions, it is important that we are importing pytest in the beginning of our test file. We need to write the following line above our test function in order to apply marker to a particular test:
@pytest.mark.marker_name
Now, look at the program below:
Python3
import mathfuncs
import pytest
@pytest .mark.algebra
def test_square():
assert mathfuncs.Algebra.square( 40 ) = = 1600
assert mathfuncs.Algebra.square( 5 ) = = 25
@pytest .mark.algebra
def test_cube():
assert mathfuncs.Algebra.cube( 40 ) = = 64000
assert mathfuncs.Algebra.cube( 5 ) = = 125
@pytest .mark.geometry
def test_is_triangle():
assert mathfuncs.Geometry.is_triangle( 120 , 40 , 20 ) = = True
assert mathfuncs.Geometry.is_triangle( 45 , 67 , 99 ) = = False
@pytest .mark.geometry
def test_is_quadrilateral():
assert mathfuncs.Geometry.is_quadrilateral( 350 , 5 , 5 , 0 ) = = True
assert mathfuncs.Geometry.is_quadrilateral( 11 , 22 , 33 , 44 ) = = False
|
Here-in, we have two markers, one named as ‘algebra’ and the other named as ‘geometry’. Now, suppose, we have to only run the tests with marker ‘geometry’, we have to enter the following command:
pytest test_mathfuncs.py -vm geometry
Output
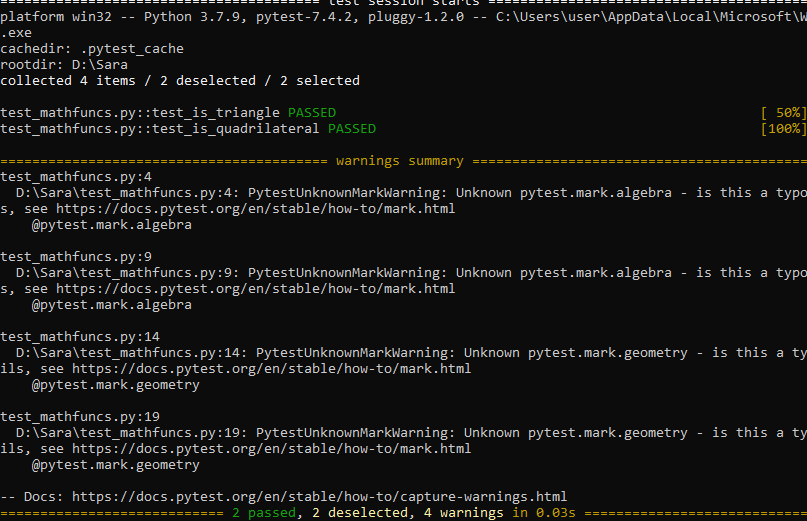
Test Results
After we run the tests, we can see that 2 tests were selected while 2 were deselected. Only the two tests with the marker “geometry” were ran. Thus, we ran the tests by grouping them. Also, we can see some warnings below. We can remove the warnings by following the steps given below:
- Making a “pytest.ini” file.
- Writing the following contents in the file:
[pytest]
markers =
algebra : custom marker for grouping the test functions inside the Algebra class
geometry : custom marker for grouping the test functions inside the Geometry class
The above file registers our markers, thus not throwing any warnings. And now, if we run the same command, we get the following output:
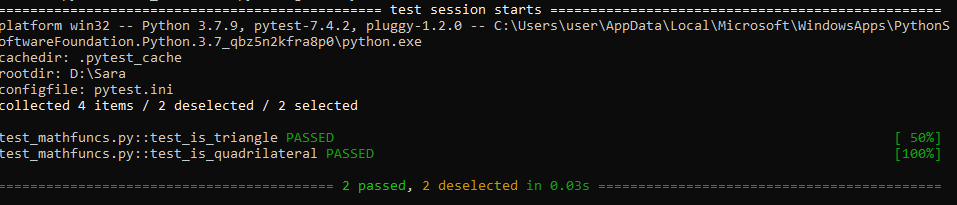
Test Results
With this, we now cannot see any type of warning. Applying markers on test functions is thus another way to group the tests.
Making another Test File
Another method to group the tests is to make separate test files for them. For example, now, we have all our tests situated in the same test file. But in case, if we want to group them in two groups, viz. Algebra and Geometry, we can make separate test files for them. Now, lets say that we want to group the tests belonging to class ‘Algebra’. For this, we will make a separate test file for it. This test file is as follows:
Python3
import mathfuncs
def test_square():
assert mathfuncs.Algebra.square( 40 ) = = 1600
assert mathfuncs.Algebra.square( 5 ) = = 25
def test_cube():
assert mathfuncs.Algebra.cube( 40 ) = = 64000
assert mathfuncs.Algebra.cube( 5 ) = = 125
|
To run the tests in this particular file only, we would write the following command:
pytest test_algebra.py -v
Output
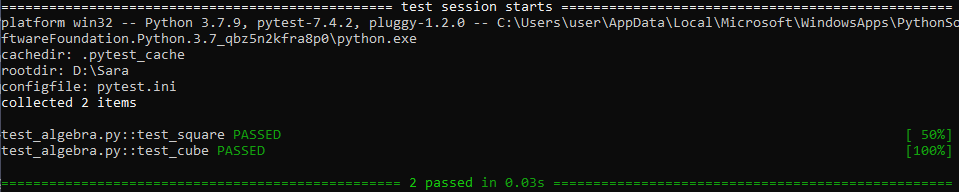
Test results
This is how, we can group the tests by making separate files.
Share your thoughts in the comments
Please Login to comment...