How to Use Pytest for Unit Testing
Last Updated :
02 Oct, 2023
Unit Testing is an important method when it comes to testing the code we have written. It is an important process, as with testing, we can make sure that our code is working right. In this article, we will see how to use Pytest for Unit Testing in Python.
Pytest for Unit Testing
Step 1: Installation
To use Pytest for unit testing, we first need to install it. We can install Pytest by writing the following command in the command prompt:
pip install pytest
To check if the pytest is installed correctly, we can type the following command in the command prompt:
pytest --version
If these commands output the version of the Pytest, then we can say that the Pytest is installed correctly otherwise there might be something wrong while installing the package.
Step 2: Naming test files and functions in Pytest
When we create certain test files for testing a module, we can run the tests in the command prompt by entering the command ‘Pytest’. But for this, it is necessary that our current working directory is the one in which our test files are stored. When we run the command ‘Pytest’ (in the directory in which the test files are stored), it itself detects the test files in that directory and its sub-directories.
For this test-files detection mechanism to work, there are rules to name the test files in Pytest. The test files in Pytest should either start with ‘test_’ or end with ‘_test’. For example, for Pytest to detect the test files, the names ‘test_file’ or ‘file_test’ are valid. If the test files do not follow these conditions, Pytest ignores those tests and doesn’t run them.
Also, the test functions/methods in the test files have some conditions of naming for their detection. The test functions/methods’ name should start with ‘test_’ or ‘test’. For example, ‘test_function’ or ‘testmethod’ are valid names for test functions/methods. In case we are making a test class, we need to start its name with ‘Test’ or ‘Test_.
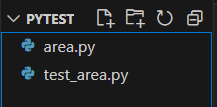
File Structure
Step 3: Writing Test Code (area.py)
Now, let’s suppose, we have to test the ‘area’ module with Pytest. We are making a file app.py that will be later on imported for the testing purposes. The following is the ‘area’ module that we have to test:
Python3
def circle(radius):
return 3.14 * radius * * 2
def square(side):
return side * * 2
def rectangle(length, breadth):
return length * breadth
def parallelogram(base, height):
return base * height
def triangle(base, height):
return 1 / 2 * (base * height)
|
Step 4: Writing Main Test file (test_area.py)
Now, to test area module inside area.py, we’ll make a test-file. For this example, we’ll make ‘test_area’ file as this name is descriptive and says that this is a test file for testing the area module.
After this, we’ll start off by writing test functions and test cases. To do the unit testing, we can either group the tests together by making a collective test class or we can make different function for the tests. Let’s consider making a test class for this one.
Python3
import area
class Test_Area:
def test_circle( self ):
assert area.circle( 14 ) = = 615.44
def test_square( self ):
assert area.square( 4 ) = = 16
def test_rectangle( self ):
assert area.rectangle( 6 , 9 ) = = 54
def test_parallelogram( self ):
assert area.parallelogram( 7 , 5 ) = = 35
def test_triangle( self ):
assert area.triangle( 12 , 10 ) = = 60
|
Step 5: Running Tests
Now after this, to run these tests, we will enter the command ‘pytest’ in the terminal. Like given below
pytest
Output

Test Results
In the above test-file, we have added only one test-case for each function in the module. We can add more test-cases as per need. The more complex the test cases, the more is the perfection in testing.
Failing of Test Cases in Unit Testing
Let us now see what happens when we fail a test-case. We are changing the area.py where we are writing area module in such a way that it fails test cases. In the module ‘area’, we have changed the return statement of circle function from ‘3.14 * radius ** 2’ to ‘3.14 * radius’, i.e., from ‘????r2’ to ‘????r’. Due to this, we shall now fail a test-case.
Python3
def circle(radius):
return 3.14 * radius
def square(side):
return side * * 2
def rectangle(length, breadth):
return length * breadth
def parallelogram(base, height):
return base * height
def triangle(base, height):
return 1 / 2 * (base * height)
|
Run the following command in the command prompt:
pytest
Output
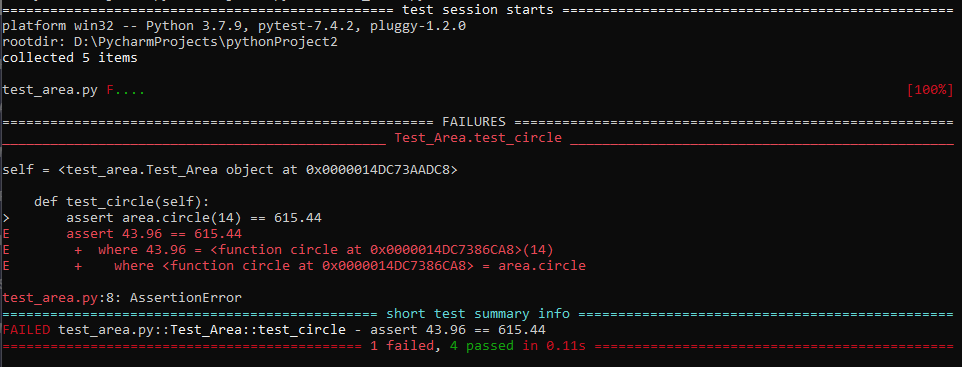
Test Results
In the above output, we can see that Pytest collected 5 items, i.e., the 5 test functions that we defined in the test file, ‘test_area’. Then we can see the name of the test file, i.e., ‘test_area.py’ which is followed by a red ‘F’ and 4 green dots. The red ‘F’ means the test that failed. The other 4 green dots mean that four tests passed. After that, Pytest shows us the failed test, i.e., method ‘test_circle’. Then we can see a summary of the test, which shows the assertion error. And finally, we can see the number of tests passed as well as the number of tests failed and the time taken to execute those tests.
Share your thoughts in the comments
Please Login to comment...