Printing triangle star pattern using a single loop
Last Updated :
23 Dec, 2023
Given a number N, the task is to print the star pattern in single loop.
Examples:Â
Input: N = 9
Output:
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * * *
* * * * * * * * *
Input: N = 5
Output:
*
* *
* * *
* * * *
* * * * *
Please Refer article for printing the pattern in two loops as:
Triangle pattern in Java
Approach 1:
Approach: The idea is to break a column into three parts and solve each part independently of the others.
- Case 1: Spaces before the first *, which takes care of printing white spaces.
- Case 2: Starting of the first * and the ending of the last * in the row, which takes care of printing alternating white spaces and *.
- Case 3: The ending star essentially tells to print a new line or end the program if we have already finished n rows.
Refer to the image below
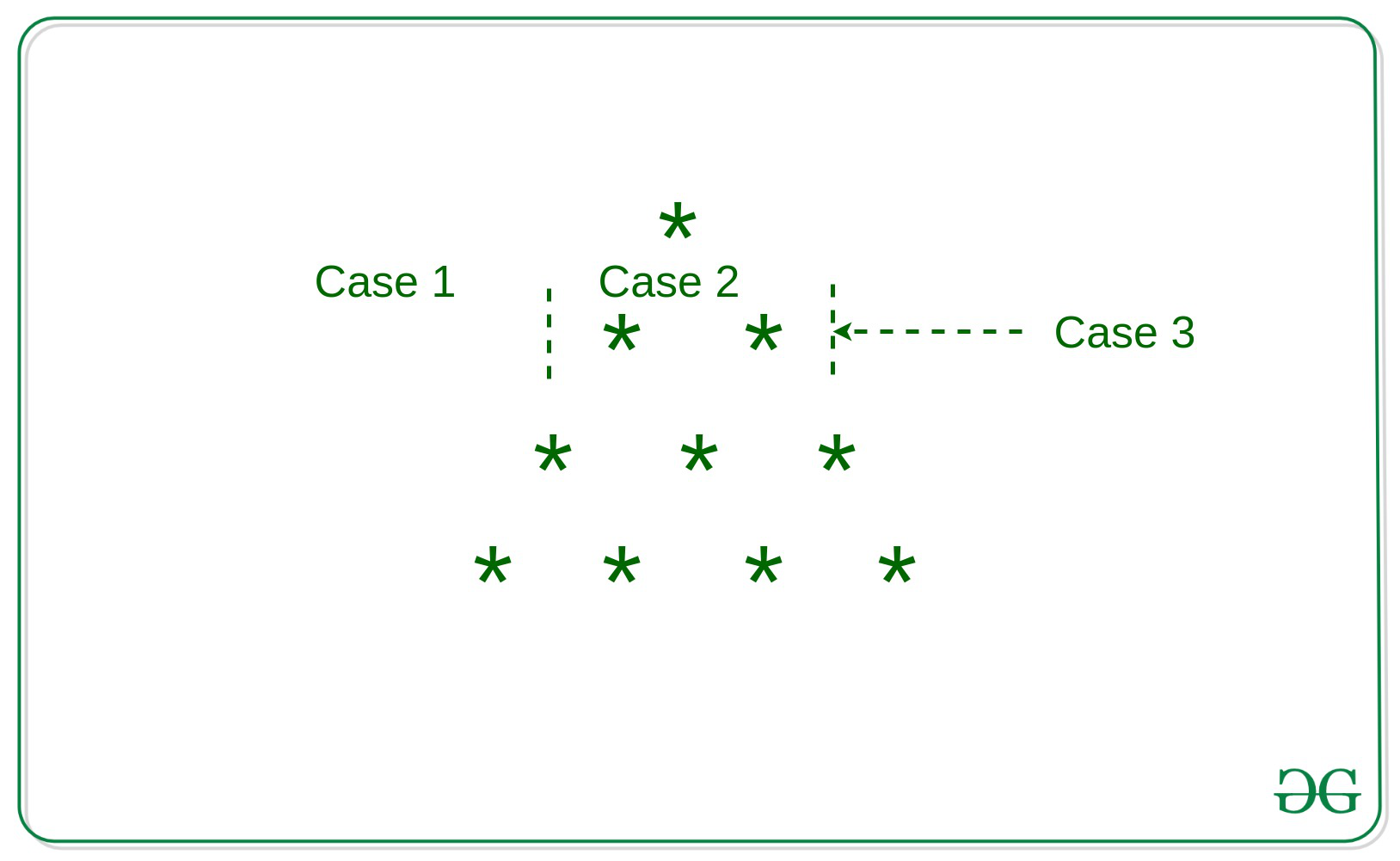
Below is the implementation of the above approach:
C++
#include <iostream>
using namespace std;
void pattern( int n)
{
int i, k, flag = 1;
for (i = 1, k = 0; i <= 2 * n - 1; i++) {
if (i < n - k)
cout << " " ;
else {
if (flag)
cout << "*" ;
else
cout << " " ;
flag = 1 - flag;
}
if (i == n + k) {
k++;
cout << endl;
if (i == 2 * n - 1)
break ;
i = 0;
flag = 1;
}
}
}
int main()
{
int n = 6;
pattern(n);
return 0;
}
|
Java
import java.util.*;
class GFG {
static void pattern( int n)
{
int i, k, flag = 1 ;
for (i = 1 , k = 0 ; i <= 2 * n - 1 ; i++) {
if (i < n - k)
System.out.print( " " );
else {
if (flag == 1 )
System.out.print( "*" );
else
System.out.print( " " );
flag = 1 - flag;
}
if (i == n + k) {
k++;
System.out.println();
if (i == 2 * n - 1 )
break ;
i = 0 ;
flag = 1 ;
}
}
}
public static void main(String[] args)
{
int n = 6 ;
pattern(n);
}
}
|
Python3
def pattern(n):
flag = 1
i = 1
k = 0
while i < = 2 * n - 1 :
if (i < n - k):
print ( " " , end = "")
else :
if (flag):
print ( "*" , end = "")
else :
print ( " " , end = "")
flag = 1 - flag
if (i = = n + k):
k + = 1
print ()
if (i = = 2 * n - 1 ):
break
i = 0
flag = 1
i + = 1
if __name__ = = "__main__" :
n = 6
pattern(n)
|
C#
using System;
class GFG {
static void pattern( int n)
{
int i, k, flag = 1;
for (i = 1, k = 0; i <= 2 * n - 1; i++) {
if (i < n - k)
Console.Write( " " );
else {
if (flag == 1)
Console.Write( "*" );
else
Console.Write( " " );
flag = 1 - flag;
}
if (i == n + k) {
k++;
Console.WriteLine();
if (i == 2 * n - 1)
break ;
i = 0;
flag = 1;
}
}
}
public static void Main()
{
int n = 6;
pattern(n);
}
}
|
Javascript
<script>
function pattern(n) {
var i,
k,
flag = 1;
for (i = 1, k = 0; i <= 2 * n - 1; i++)
{
if (i < n - k)
document.write( " " );
else {
if (flag)
document.write( "*" );
else
document.write( " " );
flag = 1 - flag;
}
if (i == n + k) {
k++;
document.write( "<br>" );
if (i == 2 * n - 1) break ;
i = 0;
flag = 1;
}
}
}
var n = 6;
pattern(n);
</script>
|
Output
*
* *
* * *
* * * *
* * * * *
* * * * * *
Time complexity: O(n^2) for given n
Auxiliary space: O(1)
Approach 2:
The idea is to break a column into two parts and solve each part independently of the others in a much simpler manner.
Case 1: Spaces before the first *, which takes care of printing white spaces.
Case 2: Starting of the first * and the ending of the last * in the row, which takes care of printing alternating white spaces and *.
Below is the implementation of the above approach:
C++
#include <iostream>
void pattern( int n)
{
for ( int i = 0; i < n; i++) {
for ( int j = 0; j < n - i - 1; j++) {
std::cout << " " ;
}
for ( int star = 0; star < i + 1; star++) {
if (star != 0) {
std::cout << " " ;
}
std::cout << "*" ;
}
std::cout << std::endl;
}
}
int main()
{
int n = 6;
pattern(n);
return 0;
}
|
Java
import java.io.*;
class GFG {
static void pattern( int n)
{
for ( int i = 0 ; i < n; i++) {
for ( int j = 0 ; j < n - i - 1 ; j++) {
System.out.print( " " );
}
for ( int star = 0 ; star < i + 1 ; star++) {
if (star != 0 ) {
System.out.print( " " );
}
System.out.print( "*" );
}
System.out.println( "" );
}
}
public static void main(String[] args)
{
int n = 6 ;
pattern(n);
}
}
|
Python3
def pattern(n):
for i in range (n):
for j in range (n - i - 1 ):
print ( " " , end = " " )
for star in range (i + 1 ):
if star ! = 0 :
print ( " " , end = " " )
print ( "*" , end = "")
print ()
if __name__ = = "__main__" :
n = 6
pattern(n)
|
C#
using System;
class Program {
static void Pattern( int n)
{
for ( int i = 0; i < n; i++) {
for ( int j = 0; j < n - i - 1; j++) {
Console.Write( " " );
}
for ( int star = 0; star < i + 1; star++) {
if (star != 0) {
Console.Write( " " );
}
Console.Write( "*" );
}
Console.WriteLine();
}
}
static void Main()
{
int n = 6;
Pattern(n);
}
}
|
Javascript
function pattern(n) {
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
process.stdout.write( " " );
}
for (let star = 0; star < i + 1; star++) {
if (star !== 0) {
process.stdout.write( " " );
}
process.stdout.write( "*" );
}
console.log();
}
}
const n = 6;
pattern(n);
|
Output
*
* *
* * *
* * * *
* * * * *
* * * * * *
Time complexity: O(n^2) for given n
Auxiliary space: O(1)
Share your thoughts in the comments
Please Login to comment...