Program to Print Butterfly Pattern (Star Pattern)
Last Updated :
10 Feb, 2024
Given an integer N, print N rows of Butterfly pattern.
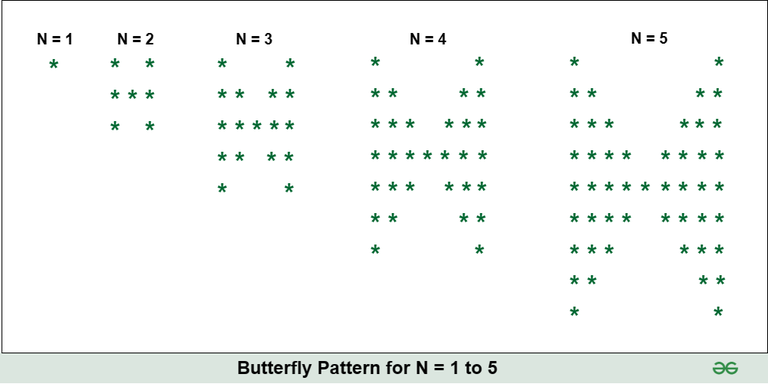
Examples:
Input: 3
Output:
* *
** **
*****
** **
* *
Input: 5
Output:
* *
** **
*** ***
**** ****
*********
**** ****
*** ***
** **
* *
Approach:
The problem can be solved using three nested loops inside an outer loop. The outer loop will run for the rows, the first inner loop will print the stars, the second inner loop will print the spaces and the third inner loop will again print the stars.
Step-by-step algorithm:
- Maintain two variable spaces = 2 * N – 1 and stars = 0 to store the number of spaces and stars for each row.
- Run an outer loop from i = 1 to the number of rows (2 * N – 1).
- If we are in the upper half of the butterfly, decrease number of spaces by 2 and increase number of stars by 1.
- If we are in the lower half of the butterfly, increase number of spaces by 2 and decrease number of stars by 1.
- Run an inner loop from j = 1 to stars.
- Print an asterisk in each iteration of the inner loop.
- Run an inner loop from j = 1 to spaces.
- Print a space in each iteration of the inner loop.
- Run an inner loop from j = 1 to stars.
- Print an asterisk in each iteration of the inner loop.
- Print a newline character (“\n”) to move to the next row.
- After N iterations, we will have the right half pyramid pattern.
Below is the implementation of the above approach:
C++
#include <iostream>
using namespace std;
int main()
{
int N = 5;
int spaces = 2 * N - 1;
int stars = 0;
for ( int i = 1; i <= 2 * N - 1; i++) {
if (i <= N) {
spaces = spaces - 2;
stars++;
}
else {
spaces = spaces + 2;
stars--;
}
for ( int j = 1; j <= stars; j++) {
cout << "*" ;
}
for ( int j = 1; j <= spaces; j++) {
cout << " " ;
}
for ( int j = 1; j <= stars; j++) {
if (j != N) {
cout << "*" ;
}
}
cout << "\n" ;
}
return 0;
}
|
Java
public class ButterflyPattern {
public static void main(String[] args) {
int N = 5 ;
int spaces = 2 * N - 1 ;
int stars = 0 ;
for ( int i = 1 ; i <= 2 * N - 1 ; i++) {
if (i <= N) {
spaces = spaces - 2 ;
stars++;
}
else {
spaces = spaces + 2 ;
stars--;
}
for ( int j = 1 ; j <= stars; j++) {
System.out.print( "*" );
}
for ( int j = 1 ; j <= spaces; j++) {
System.out.print( " " );
}
for ( int j = 1 ; j <= stars; j++) {
if (j != N) {
System.out.print( "*" );
}
}
System.out.println();
}
}
}
|
Python3
N = 5
spaces = 2 * N - 1
stars = 0
for i in range ( 1 , 2 * N):
if i < = N:
spaces = spaces - 2
stars + = 1
else :
spaces = spaces + 2
stars - = 1
for j in range ( 1 , stars + 1 ):
print ( "*" , end = "")
for j in range ( 1 , spaces + 1 ):
print ( " " , end = "")
for j in range ( 1 , stars + 1 ):
if j ! = N:
print ( "*" , end = "")
print ()
|
C#
using System;
class Program
{
static void Main()
{
int N = 5;
int spaces = 2 * N - 1;
int stars = 0;
for ( int i = 1; i <= 2 * N - 1; i++)
{
if (i <= N)
{
spaces = spaces - 2;
stars++;
}
else
{
spaces = spaces + 2;
stars--;
}
for ( int j = 1; j <= stars; j++)
{
Console.Write( "*" );
}
for ( int j = 1; j <= spaces; j++)
{
Console.Write( " " );
}
for ( int j = 1; j <= stars; j++)
{
if (j != N)
{
Console.Write( "*" );
}
}
Console.WriteLine();
}
}
}
|
Javascript
const N = 5;
let spaces = 2 * N - 1;
let stars = 0;
for (let i = 1; i <= 2 * N - 1; i++) {
if (i <= N) {
spaces = spaces - 2;
stars++;
}
else {
spaces = spaces + 2;
stars--;
}
for (let j = 1; j <= stars; j++) {
process.stdout.write( "*" );
}
for (let j = 1; j <= spaces; j++) {
process.stdout.write( " " );
}
for (let j = 1; j <= stars; j++) {
if (j !== N) {
process.stdout.write( "*" );
}
}
process.stdout.write( "\n" );
}
|
Output
* *
** **
*** ***
**** ****
*********
**** ****
*** ***
** **
* *
Time Complexity: O(N^2), where N is the number of rows in the pattern.
Auxiliary Space: O(1)
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...