Program to Print Right Half Pyramid Pattern (Star Pattern)
Last Updated :
29 Jan, 2024
Given an integer N, print N rows of right half pyramid pattern. In right half pattern of N rows, the first row has 1 star, second row has 2 stars and so on till the Nth row which has N stars. All the stars are left aligned.
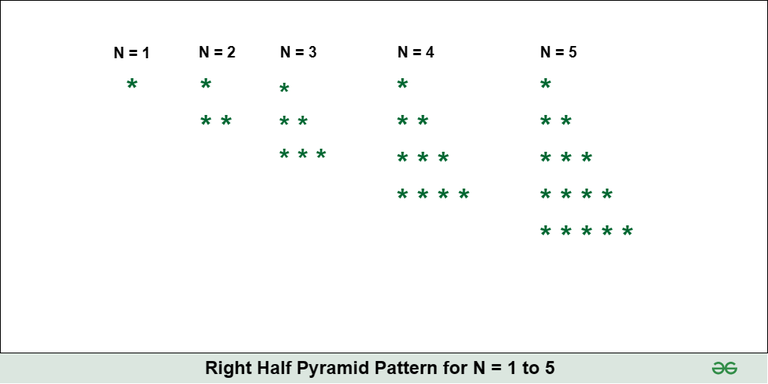
Examples:
Input: 3
Output:
*
**
***
Input: 5
Output:
*
**
***
****
*****
Approach:
The problem can be solved using two nested loops. The outer loop will run for the rows and the inner loop will print the stars.
Step-by-step approach:
- Run an outer loop from i = 1 to the number of rows.
- Run an inner loop from j = 1 to i.
- Print an asterisk in each iteration of the inner loop.
- Print a newline character (“\n”) to move to the next row.
- After N iterations, we will have the right half pyramid pattern.
Below is the implementation of the above approach:
C++
#include <iostream>
using namespace std;
int main()
{
int N = 5;
for ( int i = 1; i <= N; i++) {
for ( int j = 1; j <= i; j++) {
cout << "*" ;
}
cout << "\n" ;
}
return 0;
}
|
Java
import java.util.Scanner;
public class Main {
public static void main(String[] args)
{
int N = 5 ;
for ( int i = 1 ; i <= N; i++) {
for ( int j = 1 ; j <= i; j++) {
System.out.print( "*" );
}
System.out.println();
}
}
}
|
Python3
N = 5
for i in range ( 1 , N + 1 ):
for j in range ( 1 , i + 1 ):
print ( "*" , end = "")
print ()
|
C#
using System;
class Program
{
static void Main()
{
int N = 5;
for ( int i = 1; i <= N; i++)
{
for ( int j = 1; j <= i; j++)
{
Console.Write( "*" );
}
Console.WriteLine();
}
}
}
|
Javascript
let N = 5;
for (let i = 1; i <= N; i++) {
for (let j = 1; j <= i; j++) {
process.stdout.write( "*" );
}
console.log();
}
|
Output
*
**
***
****
*****
Time Complexity: O(N2), where N is the number of rows in the pattern.
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...