POTD Solutions | 28 Oct’ 23 | Bleak Numbers
Last Updated :
31 Oct, 2023
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Number Theory and Bit manipulation but will also help you build up problem-solving skills.
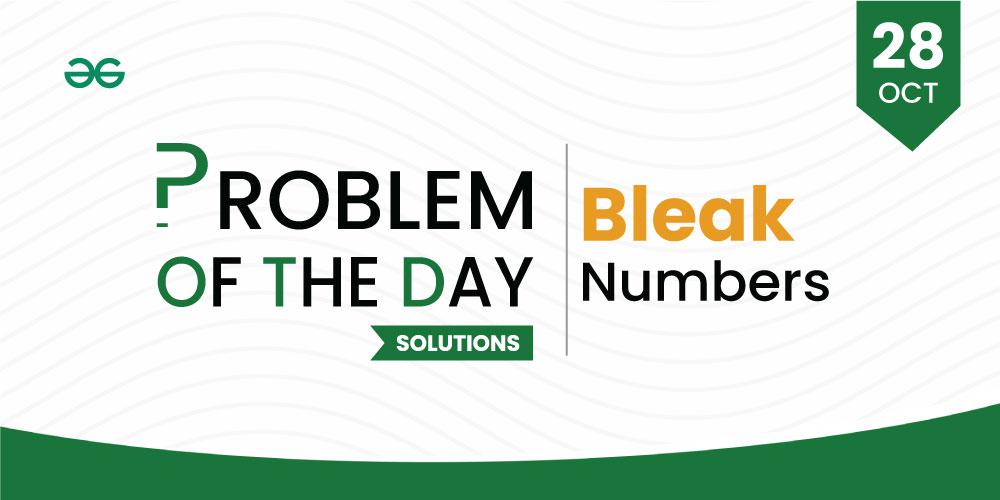
POTD 28 Oct
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 28 October: Bleak Numbers
Given an integer, check whether it is Bleak or not. A number n is called Bleak if it cannot be represented as a sum of a positive number x and set bit count in x, i.e., x + countSetBits(x) is not equal to n for any non-negative number x.
Example:
Input: n = 3
Output: false
Explanation: 3 is not Bleak as it can be represented
as 2 + countSetBits(2).
Input: n = 4
Output: true
Explanation: 4 is t Bleak as it cannot be represented
as sum of a number x and countSetBits(x)
for any number x.
The idea is based on the fact that the largest count of set bits in any number smaller than n cannot exceed ceiling of Log2n. So we need to check only numbers from range n – ceilingLog2(n) to n.
Step-by-step approach:
- Implement a function countSetBits() to count the number of set bits in a given integer.
- Iterate through all possible values of x from (n-logn) to n.
- Check if i + countSetBits(i) is equal to n, then return 0.
- If no such x is found, return 1.
Below is the implementation of the above approach:
C++
class Solution {
public:
int is_bleak(int n)
{ // itereate from n-log2(n) to n
for (int i = n - log2(n); i < n; i++) {
// count the number of set bits in i
int x = __builtin_popcount(i);
// condition for not being a bleak number
if (i + x == n)
return 0;
}
return 1;
}
};
Java
class Solution
{
public int is_bleak(int n)
{
for (int i = n - (int)(Math.log(n) / Math.log(2)); i < n; i++) {
// Count the number of set bits in i
int x = Integer.bitCount(i);
// Condition for not being a bleak number
if (i + x == n) {
return 0;
}
}
return 1;
}
}
Python3
import math
class Solution:
def is_bleak(self, n):
# Iterate from n - math.floor(log2(n)) to n
for i in range(n - math.floor(math.log2(n)), n):
# Count the number of set bits in i
x = bin(i).count('1')
# Condition for not being a bleak number
if i + x == n:
return 0
return 1