POTD Solutions | 23 Oct’ 23 | Maximum Sum Increasing Subsequence
Last Updated :
22 Nov, 2023
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Dynamic Programming but will also help you build up problem-solving skills.
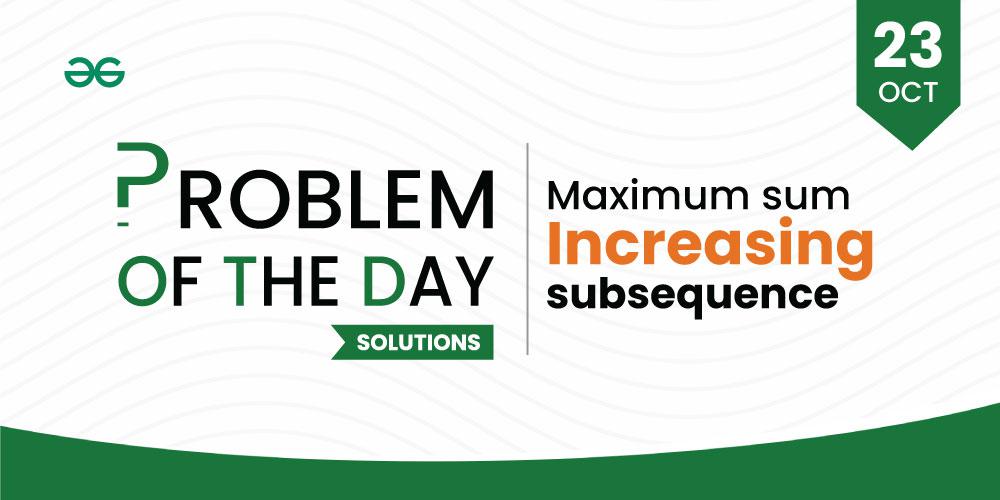
POTD Banner Image
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 23 October: Maximum sum increasing subsequence
Given an array of n positive integers. Find the sum of the maximum sum subsequence of the given array such that the integers in the subsequence are sorted in strictly increasing order i.e. a strictly increasing subsequence.
Example 1:
Input: N = 5, arr[] = {1, 101, 2, 3, 100}
Output: 106
Explanation: The maximum sum of a increasing sequence is obtained from {1, 2, 3, 100}
Example 2:
Input: N = 4, arr[] = {4, 1, 2, 3}
Output: 6
Explanation: The maximum sum of a increasing sequence is obtained from {1, 2, 3}.
This problem is a variation of the standard Longest Increasing Subsequence (LIS) problem. We need a slight change in the Dynamic Programming solution of LIS problem. All we need to change is to use sum as a criteria instead of a length of increasing subsequence.
Step-by-step algorithm:
- Maintain a msis[] array, such that msis[i] stores the maximum sum of the increasing subsequence having last element as arr[i].
- Initialize msis[i] with arr[i]
- Iterate from i = 1 to i = N-1
- Check for all the indices j less than i such that (arr[i] > arr[j]) and update msis[i] is we can get greater sum using the subsequence ending at j.
- After iterating over the entire array, return the maximum element in msis.
Below is the implementation of above approach:
C++
class Solution {
public :
int maxSumIS( int arr[], int n)
{
int i, j, max = 0;
int msis[n];
for (i = 0; i < n; i++)
msis[i] = arr[i];
for (i = 1; i < n; i++)
for (j = 0; j < i; j++)
if (arr[i] > arr[j]
&& msis[i] < msis[j] + arr[i])
msis[i] = msis[j] + arr[i];
for (i = 0; i < n; i++)
if (max < msis[i])
max = msis[i];
return max;
}
};
|
Java
class Solution {
public int maxSumIS( int arr[], int n)
{
int i, j, max = 0 ;
int msis[] = new int [n];
for (i = 0 ; i < n; i++)
msis[i] = arr[i];
for (i = 1 ; i < n; i++)
for (j = 0 ; j < i; j++)
if (arr[i] > arr[j]
&& msis[i] < msis[j] + arr[i])
msis[i] = msis[j] + arr[i];
for (i = 0 ; i < n; i++)
if (max < msis[i])
max = msis[i];
return max;
}
}
|
Python3
class Solution:
def maxSumIS( self , Arr, n):
max = 0
msis = [ 0 for x in range (n)]
for i in range (n):
msis[i] = Arr[i]
for i in range ( 1 , n):
for j in range (i):
if (Arr[i] > Arr[j] and
msis[i] < msis[j] + Arr[i]):
msis[i] = msis[j] + Arr[i]
for i in range (n):
if max < msis[i]:
max = msis[i]
return max
|
Javascript
class Solution {
maxSumIS(arr,n){
let i, j, max = 0;
let msis = new Array(n);
for (i = 0; i < n; i++)
msis[i] = arr[i];
for (i = 1; i < n; i++)
for (j = 0; j < i; j++)
if (arr[i] > arr[j] &&
msis[i] < msis[j] + arr[i])
msis[i] = msis[j] + arr[i];
for (i = 0; i < n; i++)
if (max < msis[i])
max = msis[i];
return max;
}
}
|
Time Complexity: O(N ^ 2), where N is the length of the input array
Auxiliary Space: O(N)
Share your thoughts in the comments
Please Login to comment...