POTD Solutions | 07 Nov’ 23 | Sum of upper and lower triangles
Last Updated :
22 Nov, 2023
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Matrix but will also help you build up problem-solving skills.
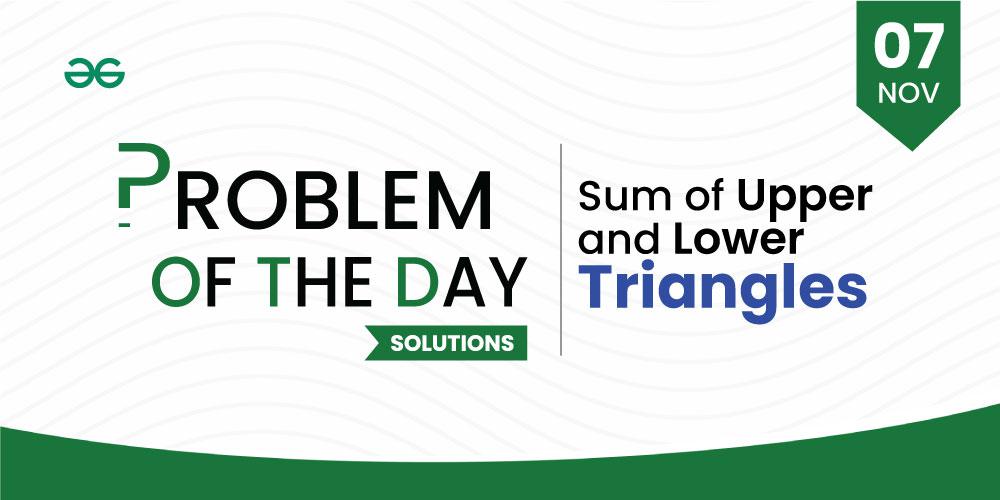
POTD Solutions 07 November 2023
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 07 November: Sum of upper and lower triangles
Given a square matrix of size N*N, print the sum of upper and lower triangular elements. Upper Triangle consists of elements on the diagonal and above it. The lower triangle consists of elements on the diagonal and below it.
Examples:
Input: {{6, 5, 4}, {1, 2, 5}, {7, 9, 7}}
Output: 29 32
Explanation: Sum of upper triangle = 6 + 5 + 4 + 2 + 5 + 7 = 29 and Sum of lower triangle = 6 + 1 + 2 + 7 + 9 + 7 = 32
Input: {{1, 1, 1}, {2, 2, 2}, {3, 3, 3}}
Output: 10 14
Explanation: Sum of upper triangle = 1 + 1 + 1 + 2 + 2 + 3 = 10 and Sum of lower triangle = 1 + 2 + 2 + 3 + 3 + 3 = 14
Traverse the matrix and calculate the sum for upper and lower triangles accordingly. For upper triangle, sum all the cells which have row <= column and for lower triangle, sum all the cells which have row >= column.
Below is the implementation of the above approach:
C++
class Solution {
public :
vector< int >
sumTriangles( const vector<vector< int > >& matrix, int n)
{
int i, j;
int upper_sum = 0;
int lower_sum = 0;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
if (i <= j) {
upper_sum += matrix[i][j];
}
}
}
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
if (j <= i) {
lower_sum += matrix[i][j];
}
}
}
return vector< int >{ upper_sum, lower_sum };
}
};
|
C
int * sumTriangles( int n, int matrix[][n])
{
int * ans = ( int *) malloc (2 * sizeof ( int ));
int i, j;
int upper_sum = 0;
int lower_sum = 0;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
if (i <= j) {
upper_sum += matrix[i][j];
}
}
}
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
if (j <= i) {
lower_sum += matrix[i][j];
}
}
}
ans[0] = upper_sum;
ans[1] = lower_sum;
return ans;
}
|
Java
class Solution {
static ArrayList<Integer> sumTriangles( int matrix[][],
int n)
{
ArrayList<Integer> ans = new ArrayList<Integer>();
int i, j;
int upper_sum = 0 ;
int lower_sum = 0 ;
for (i = 0 ; i < n; i++) {
for (j = 0 ; j < n; j++) {
if (i <= j) {
upper_sum += matrix[i][j];
}
}
}
for (i = 0 ; i < n; i++) {
for (j = 0 ; j < n; j++) {
if (j <= i) {
lower_sum += matrix[i][j];
}
}
}
ans.add(upper_sum);
ans.add(lower_sum);
return ans;
}
}
|
Python3
class Solution:
def sumTriangles( self , matrix, n):
i, j = 0 , 0
upper_sum = 0
lower_sum = 0
ans = [ 0 , 0 ]
for i in range (n):
for j in range (n):
if (i < = j):
upper_sum + = matrix[i][j]
for i in range (n):
for j in range (n):
if (j < = i):
lower_sum + = matrix[i][j]
ans[ 0 ] = upper_sum
ans[ 1 ] = lower_sum
return ans
|
Javascript
class Solution
{
sumTriangles(mat, N)
{
let i, j;
let upper_sum = 0;
let lower_sum = 0;
let ans = [0, 0];
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
if (i <= j) {
upper_sum += mat[i][j];
}
}
}
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
if (j <= i) {
lower_sum += mat[i][j];
}
}
}
ans[0] = upper_sum;
ans[1] = lower_sum;
return ans;
}
}
|
Time Complexity: O(N*N), where N is the number of rows or columns in the matrix
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...