PL/SQL GOTO Statement
Last Updated :
01 Jan, 2024
PL/SQL also known as Procedural Language/Structured Query Language, PL/SQL is a powerful programming language used in Oracle databases to do interaction with data. One of its features is the GOTO statement, which helps control how a program flows by allowing it to jump to specific statements within the same part of the program.
What is the GOTO Statement in PL/SQL?
The GOTO statement within PL/SQL serves as an important programming construct, allowing the redirection of code execution to a labeled section within the same block. GOTO statement allows us to modify the sequential order of our code, providing a means to alter the program’s flow. However, excessive usage of the GOTO statement can result in various drawbacks that impact code quality and readability.
How Does the GOTO Statement Work?
The GOTO statement within PL/SQL serves as a programming feature instructing a program to shift control to a designated portion of code identified by a label. Usually, this label denotes a distinct line or block of code within the program. Upon encountering the GOTO statement during program execution, the control flow skips to the labeled position, enabling the code to resume execution from that particular point
GOTO Statements Work
- Labeling: Programmers typically place labels at specific points in their code, marking particular locations or sections for better understanding for jumping.
- Execution Flow: Upon encountering a GOTO statement, the program immediately redirects its execution to the line or block of code identified by the label. This non-sequential execution can make the code harder to comprehend and follow for the programmer.
- Control Flow Considerations: Excessive use of GOTO statements can result in what’s termed “spaghetti code,” where the flow of execution becomes convoluted, making it challenging to trace. Debugging and maintaining such code becomes more difficult as the program’s behavior becomes less predictable.
- Modern Practices: Most contemporary programming languages discourage or completely avoid GOTO statements due to their potential to generate intricate and error-prone code. Instead, structured programming constructs like loops, conditional statements (if-else), and functions/methods are preferred. These constructs offer improved readability, maintainability, and control flow without relying on unconditional jumps.
- Exceptions: Despite the overall discouragement of GOTO statements, specific scenarios, such as error handling in certain languages or low-level programming, might still necessitate the judicious use of GOTO-like constructs.
GOTO Statement in PL/SQL
The GOTO statement in PL/SQL allows for an unconditional transfer of control within the same subprogram to a labeled statement. Its syntax is as follows:
GOTO label;
…
…
<< label >>
statement;
Restrictions with the GOTO Statement
Understanding the limitations of the GOTO statement is crucial:
- Control Flow Limitations: GOTO cannot jump into IF, CASE, LOOP, or sub-blocks.
- Branching Limitations: It cannot jump between different clauses within IF or CASE statements.
- Block and Exception Handling Restrictions: GOTO cannot move from an outer block to a sub-block or out of a subprogram. Additionally, it cannot branch back from an exception handler into the current block.
- Debugging Challenges: Programs with GOTO statements are often harder to debug, as the flow of control becomes less predictable.
- Exception Handling Issues: In structured languages with exception handling mechanisms, GOTO statements often cannot branch back from an exception handler into the current block. This restriction prevents unstructured or unexpected control flow, ensuring that exception handling maintains its predictability.
PL/SQL Code Using the GOTO Statement Along with an Explanation
DECLARE
a NUMBER := 1;
BEGIN
<<my_label>>
-- Displaying values from 1 to 5
WHILE a <= 5 LOOP
DBMS_OUTPUT.PUT_LINE('Value of a: ' || a);
a := a + 1;
IF a = 4 THEN
GOTO my_label; -- Redirects control to the label 'my_label'
END IF;
END LOOP;
END;
Output
Value of a: 1
Value of a: 2
Value of a: 3
Value of a: 4
Value of a: 5
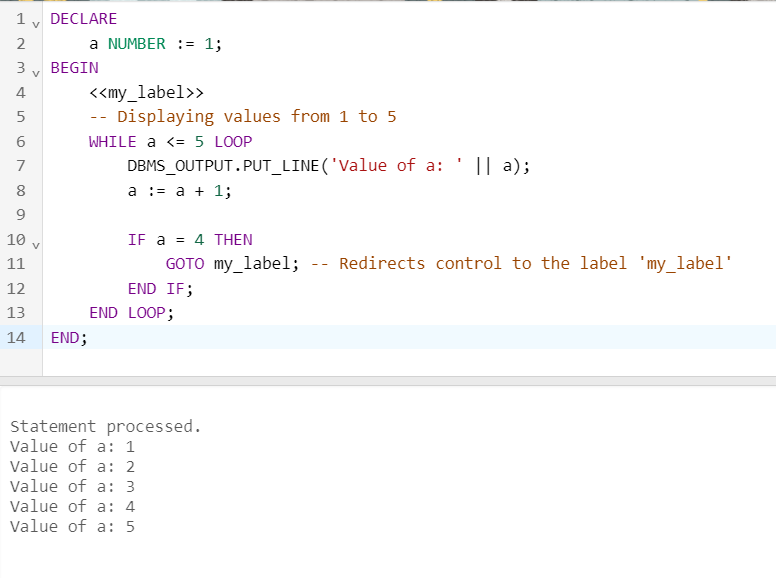
Output
Explanation
- This PL/SQL block initializes The variable a with a value of 1.
- It enters a loop that will display the value of a and increment it by 1 in each iteration.
- Within the loop, there is an IF condition that checks if a equals 4. When a is 4, it triggers the GOTO statement.
- The GOTO statement redirects the program flow back to the labeled statement <<my_label>>, effectively restarting the loop.
- The loop continues until a reaches 5.
Example
DECLARE
num NUMBER;
BEGIN
DBMS_OUTPUT.PUT_LINE('Welcome to the Program');
num := -2;
IF num > 0 THEN
DBMS_OUTPUT.PUT_LINE('You entered a positive number.');
GOTO end_of_program;
ELSE
DBMS_OUTPUT.PUT_LINE('You entered either zero or a negative number.');
END IF;
<<end_of_program>>
DBMS_OUTPUT.PUT_LINE('End of the Program');
END;
Output:
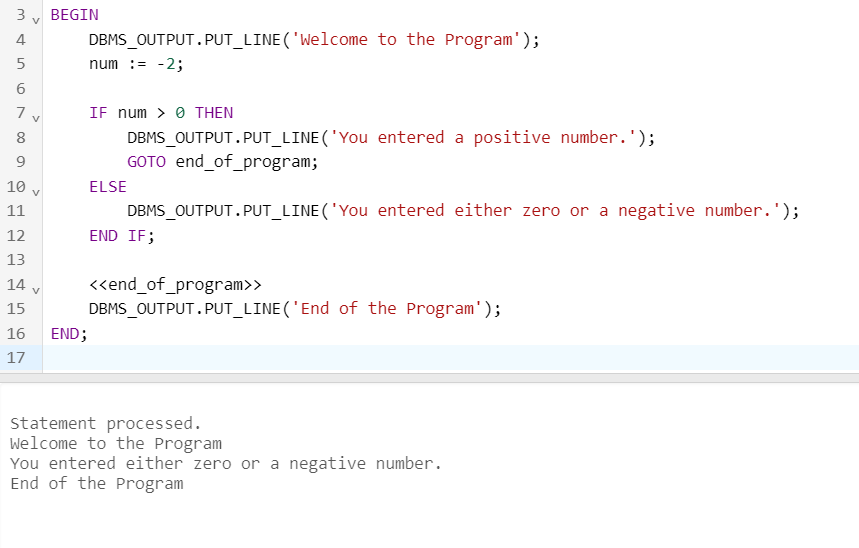
Output
Explanation of the Code
- Displays a welcome message to the user when the program starts.
- Input a number.
- The program checks if the input number is less than or equal to zero. If it is, the program jumps using the GOTO statement.
- If the number is positive (not zero or negative), this line gets executed, indicating the input is a positive number.
- If the input number by user is zero or negative, this line gets executed, indicating that the input is either zero or a negative number.
- Marks the end of the program and displays a concluding message.
Conclusion
In conclusion, the GOTO statement in PL/SQL provides a means to control program flow by transferring to labeled statements. However, its usage is discouraged due to its potential to complicate code readability and maintainability in future and if someone else other than the person who has written the code tries to read the code it becomes tough for him the understand. It’s essential to employ structured programming techniques wherever possible to enhance code quality.
Share your thoughts in the comments
Please Login to comment...