PL/SQL, the Procedural Language/Structured Query Language, is a database programming language utilized for database management in Oracle. Within this language, the Null statement plays a crucial role in enhancing code readability and functionality. This article aims to delve into the significance of the Null statement in PL/SQL, exploring its various applications and benefits.
Understanding the Null Statement
The Null statement in PL/SQL is a construct or feature that represents a no-operation or empty statement. It is used when a statement is syntactically necessary but no action needs to be performed on that statement. Essentially, it signifies the placeholder where no code execution occurs.
The NULL statement works like a stand-in within PL/SQL code. It’s needed by the syntax but doesn’t do any particular job. It shows that no specific code has to run at that moment. This helps keep the code in good shape when a statement is a must but the statement does not need to take actual action.
It doesn’t do any task at all, it is just a placeholder signifying that nothing particular needs to be done. It’s like saying, “No code required here!”
In exception handling also this NULL statement tells us that we don’t have to do anything special for certain problems. It’s like a little note saying, “No need to handle this one especially.”
Basic Syntax of PL/SQL Null Statement
The basic syntax of the NULL statement in PL/SQL is very simple. It’s just the keyword NULL followed by a semicolon (;).
— Some PL/SQL code executing other statements
— The NULL statement used as a placeholder
NULL;
— More PL/SQL code
END;
Using PL/SQL NULL Statement to Improve Code Readability
The Null statement proves particularly useful in enhancing code readability and usability. Consider a scenario where a placeholder is required within a block of code, but no specific action is needed to take place:
BEGIN
— Perform certain operations
NULL; — Null statement as a placeholder
— Continue with other operations
END;
By employing the Null statement in such instances, developers can clearly denote sections in the code that require no action, making the code more understandable and readable for other programmers or during future reviews and modifications.
Creating Placeholders for Subprograms
Another significant application of the Null statement is creating placeholders within subprograms. For instance, when defining a procedure or function body, placeholders might be needed:
CREATE OR REPLACE PROCEDURE myProcedure AS
BEGIN
-- Perform some tasks
NULL; -- Placeholder for future functionality
-- Continue with more tasks
END;
In this case, the Null statement serves as a placeholder for potential future modifications or additions to the subprogram’s logic, allowing developers to structure code architecture more effectively.
Using PL/SQL NULL Statement to Provide a Target for a GOTO
The Null statement can also act as a target for a GOTO statement, aiding in controlling program flow:
DECLARE
x INT := 3;
BEGIN
IF x > 5 THEN
DBMS_OUTPUT.PUT_LINE('x is greater than 5');
ELSE
NULL; -- The NULL statement does nothing in the ELSE block
END IF;
END;
By utilizing the Null statement as a target for GOTO in the above code can navigate program execution to a designated placeholder within the code very easily.
Explanation
We set a variable ‘x’ to the value 3. The IF statement checks if ‘x’ is bigger than 5. But, since ‘x’ is not greater than 5 (it’s 3, not more than 5), the condition in the IF statement is not true.
When this happens, the program goes into the ELSE part. Inside that part, there’s a statement that does nothing, called NULL. It’s there just because the IF-ELSE needs something inside the ELSE part.
So, when ‘x’ is not greater than 5, the program sees the NULL inside the ELSE part, and it keeps going to the end of the PL/SQL part without doing anything special in the ELSE part.
Output:
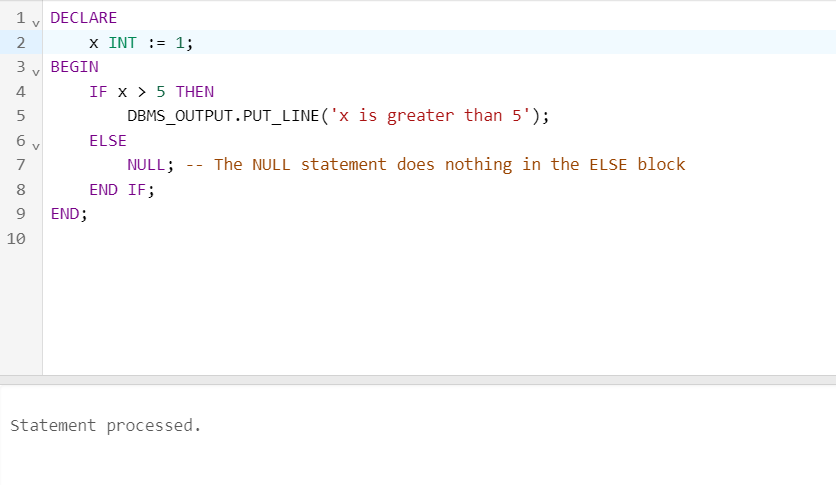
Output
NULL and Exception Handling
In PL/SQL, a NULL statement can act as a placeholder for the program , indicating that no particular action is needed to perform. When incorporated into exception handling, this NULL statement within the EXCEPTION block signifies that no specific action is required to address a certain error situation.
BEGIN
-- Some operations or code execution
-- Exception handling block
BEGIN
-- Code that might raise an exception
DECLARE
numerator INT := 10;
denominator INT := 0;
result INT;
BEGIN
result := numerator / denominator; -- This line may raise a division by zero exception
EXCEPTION
WHEN ZERO_DIVIDE THEN
-- NULL statement used as a placeholder for no specific action needed
NULL; -- Placeholder: No action required for this specific condition
END;
END;
END;
Explanation
Within the catch part, the code tries dividing a number where the bottom bit is put to zero, which might cause a ZERO_DIVIDE error.
In case of a ZERO_DIVIDE error, the EXCEPTION WHEN ZERO_DIVIDE THEN part catches it.
Instead of doing anything particular for this issue, the NULL thing is used as a placeholder, showing that nothing needs to be done or planned for this specific error.
Output:
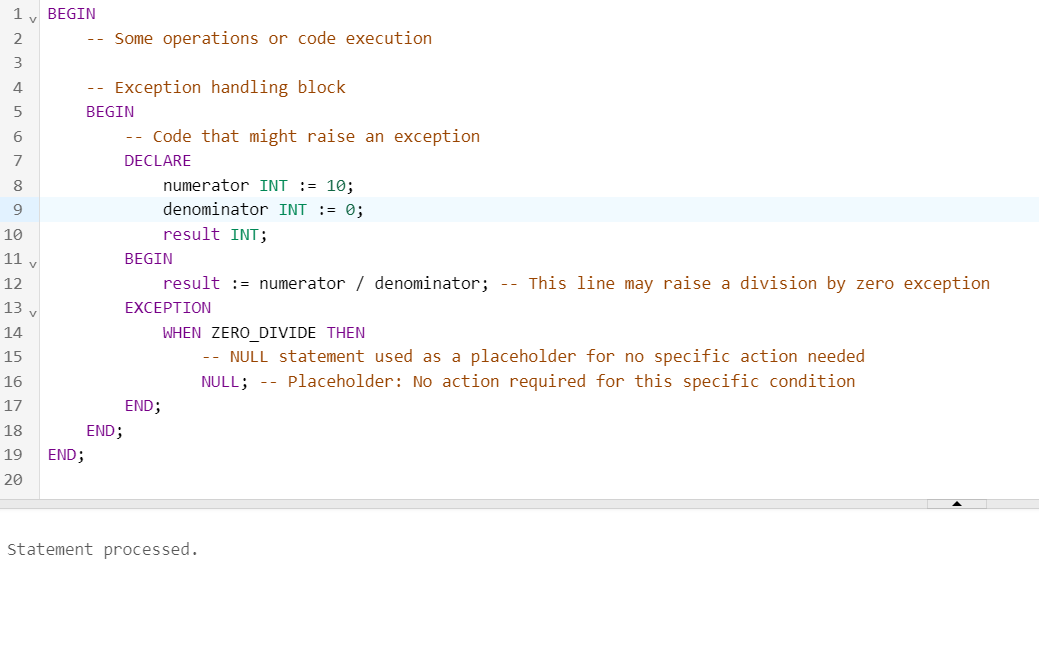
Output
Conclusion
In summary, the null statement in PL/SQL serves as a useful tool to enhance code clarity and functionality by acting as a placeholder within subprograms, aiding in flow control. of code, Its flexibility in serving as a placeholder where no specific action is needed makes it a beneficial construct for structuring and maintaining code in a more organized manner. Incorporating the Null statement judiciously can significantly enhance the clarity and maintainability of PL/SQL codebases.
Share your thoughts in the comments
Please Login to comment...