Java Program to Goto a Link Using Applet
Last Updated :
29 Dec, 2023
In this article, we shall be animating the applet window to show the goto link in Java Applet. A Goto link is basically, any particular link that redirects us from one page to another.
Approach to Implement Goto a Link using Applet
- Create an applet with the two buttons.
- Add ActionListener to the buttons.
- Form the complete URL of the page.
- Use the Desktop class of java.awt package to open the link.
Note: Java Version : 1.8 and above required
Goto a Link using Java Applet
Below is the implementation of the above method:
Java
import java.applet.*;
import java.awt.*;
import java.awt.Desktop.*;
import java.awt.event.*;
import java.net.*;
public class GotoLink
extends Applet implements ActionListener {
public void init()
{
setBackground(Color.white);
Button b1 = new Button( "google" );
Button b2 = new Button( "facebook" );
this .add(b1);
this .add(b2);
b1.addActionListener( this );
b2.addActionListener( this );
}
public void actionPerformed(ActionEvent e)
{
String button = e.getActionCommand();
try {
Desktop.getDesktop().browse( new URI(link));
}
catch (Exception ex) {
ex.printStackTrace();
}
}
}
|
Executing the Applet Program
Save the file with the name GotoLink.java
javac GotoLink.java
Applet Viewer GotoLink.java
Output
Test case 1: Applet Output
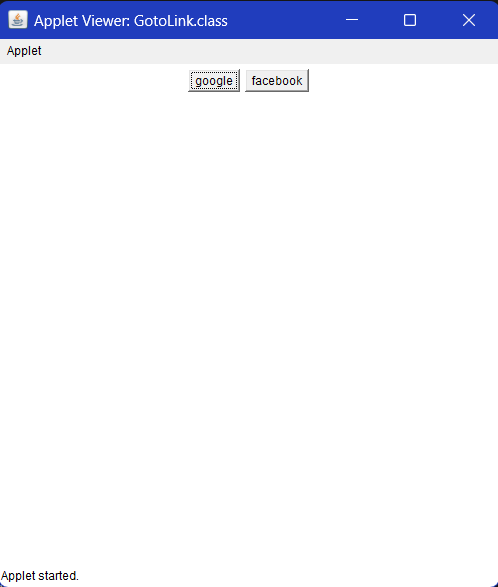
Test Case 2 : When user click on google button
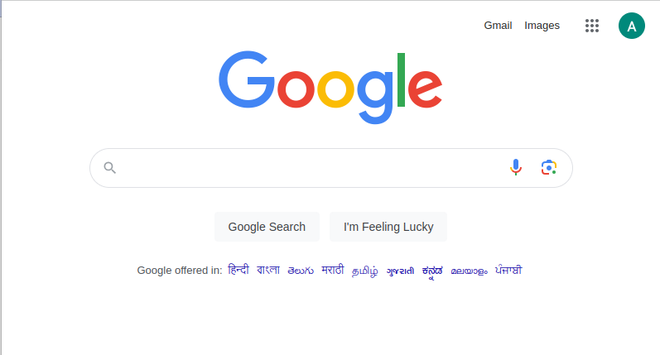
Test Case 2 : When user click on facebook button
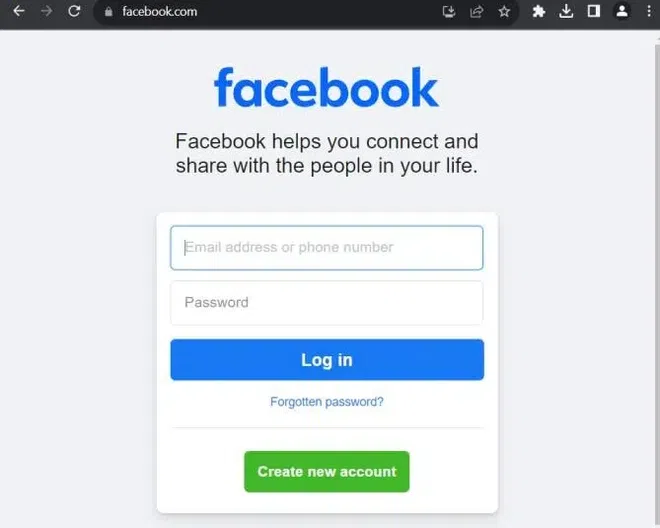
Explaination of the Above Program
- For Example: https://www.geeksforgeeks.org/ or https://www.google.com/
- Use Desktop class to handle the URI (Uniform Resource Identifier) file.
- Use method browse(URI) to open the link in the default browser of the system eg. Google Chrome.
- public void init() function is used to initialize the applet.
- public void actionPerformed(ActionEvent e) fucntion is used to check on button click what logic we have to perform in our case it will take us to external url like google.com or facebook.com
- Action Listener is used to check which button is selected.
Share your thoughts in the comments
Please Login to comment...