Php Program to multiply two matrices
Last Updated :
26 Dec, 2021
Given two matrices, the task to multiply them. Matrices can either be square or rectangular.
Examples:Â
Input : mat1[][] = {{1, 2},
{3, 4}}
mat2[][] = {{1, 1},
{1, 1}}
Output : {{3, 3},
{7, 7}}
Input : mat1[][] = {{2, 4},
{3, 4}}
mat2[][] = {{1, 2},
{1, 3}}
Output : {{6, 16},
{7, 18}}
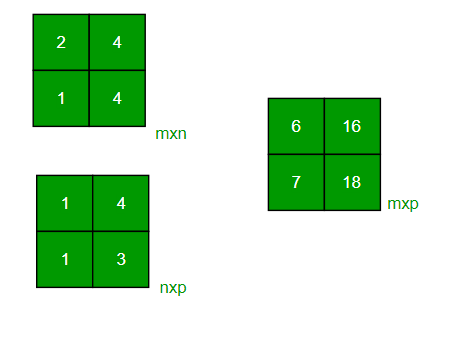
Multiplication of Square Matrices :Â
The below program multiplies two square matrices of size 4*4, we can change N for different dimensions.Â
PHP
<?php
function multiply(& $mat1 , & $mat2 , & $res )
{
$N = 4;
for ( $i = 0; $i < $N ; $i ++)
{
for ( $j = 0; $j < $N ; $j ++)
{
$res [ $i ][ $j ] = 0;
for ( $k = 0; $k < $N ; $k ++)
$res [ $i ][ $j ] += $mat1 [ $i ][ $k ] *
$mat2 [ $k ][ $j ];
}
}
}
$mat1 = array ( array (1, 1, 1, 1),
array (2, 2, 2, 2),
array (3, 3, 3, 3),
array (4, 4, 4, 4));
$mat2 = array ( array (1, 1, 1, 1),
array (2, 2, 2, 2),
array (3, 3, 3, 3),
array (4, 4, 4, 4));
multiply( $mat1 , $mat2 , $res );
$N = 4;
echo ("Result matrix is
");
for ( $i = 0; $i < $N ; $i ++)
{
for ( $j = 0; $j < $N ; $j ++)
{
echo ( $res [ $i ][ $j ]);
echo ( " " );
}
echo ("
");
}
?>
|
Output
Result matrix is
10 10 10 10
20 20 20 20
30 30 30 30
40 40 40 40
Time complexity: O(n3). It can be optimized using Strassen’s Matrix Multiplication
Auxiliary Space: O(n2)
Multiplication of Rectangular Matrices :Â
We use pointers in C to multiply to matrices. Please refer to the following post as a prerequisite of the code.
How to pass a 2D array as a parameter in C?Â
PHP
<?php
function multiply( $m1 , $m2 , $mat1 ,
$n1 , $n2 , $mat2 )
{
for ( $i = 0; $i < $m1 ; $i ++)
{
for ( $j = 0; $j < $n2 ; $j ++)
{
$res [ $i ][ $j ] = 0;
for ( $x = 0; $x < $m2 ; $x ++)
{
$res [ $i ][ $j ] += $mat1 [ $i ][ $x ] *
$mat2 [ $x ][ $j ];
}
}
}
for ( $i = 0; $i < $m1 ; $i ++)
{
for ( $j = 0; $j < $n2 ; $j ++)
{
echo $res [ $i ][ $j ] . " " ;
}
echo "
";
}
}
$mat1 = array ( array ( 2, 4 ), array ( 3, 4 ));
$mat2 = array ( array ( 1, 2 ), array ( 1, 3 ));
$m1 = 2;
$m2 = 2;
$n1 = 2;
$n2 = 2;
multiply( $m1 , $m2 , $mat1 , $n1 , $n2 , $mat2 );
?>
|
Time complexity: O(n3). It can be optimized using Strassen’s Matrix Multiplication
Auxiliary Space: O(m1 * n2)
Please refer complete article on Program to multiply two matrices for more details!
Share your thoughts in the comments
Please Login to comment...