Addition of Two Matrices in PHP
Last Updated :
16 Nov, 2023
Given two matrices mat1 and mat2, the task is to find the sum of both matrices. Matrix addition is possible only when both matrix’s dimensions are the same. If both matrices have different dimensions then addition will not be possible.
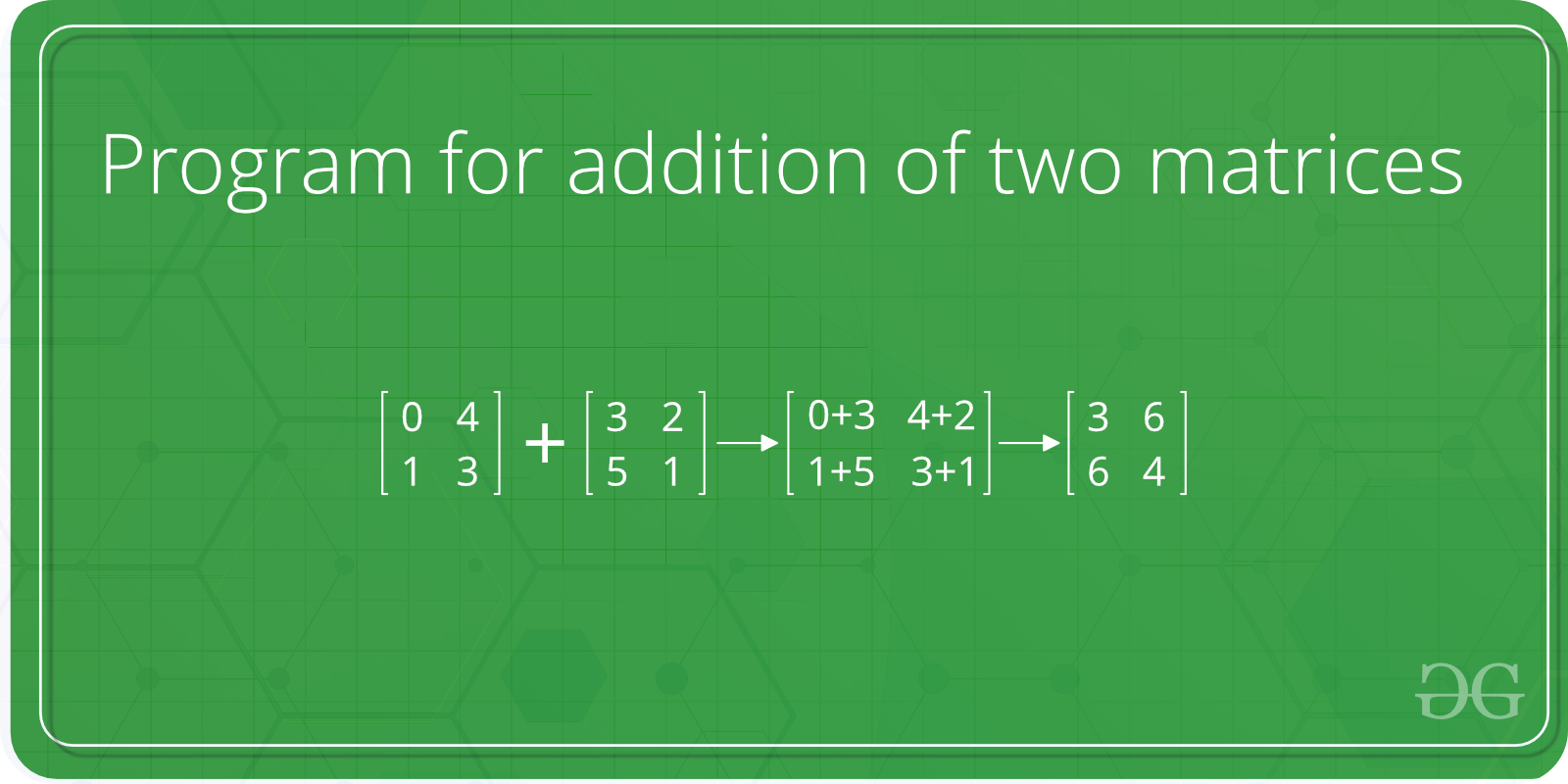
Sum of Two Matrices using for Loop
First, we declare two matrices, matrix1 and matrix2. Next, we check both matrix dimension, if both matrix dimension is same then we proceed for sum of matrices, otherwise display an error message. To add both matrices, we use for loop. For loop iterate each matrix elements one by one, and perform sum on the elements.
Example: Sum of two matrices using for Loop in PHP.
PHP
<?php
function sumTwoMatrices( $mat1 , $mat2 ) {
$rows = count ( $mat1 );
$columns = count ( $mat1 [0]);
$sum = array ();
for ( $i = 0; $i < $rows ; $i ++) {
for ( $j = 0; $j < $columns ; $j ++) {
$sum [ $i ][ $j ] = 0;
}
}
for ( $i = 0; $i < $rows ; $i ++) {
for ( $j = 0; $j < $columns ; $j ++) {
$sum [ $i ][ $j ] = $mat1 [ $i ][ $j ] + $mat2 [ $i ][ $j ];
}
}
return $sum ;
}
$matrix1 = array (
array (26, 14, 31),
array (41, 61, 11),
array (14, 12, 19)
);
$matrix2 = array (
array (11, 8, 27),
array (21, 15, 25),
array (22, 15, 21)
);
$rows1 = count ( $matrix1 );
$columns1 = count ( $matrix1 [0]);
$rows2 = count ( $matrix2 );
$columns2 = count ( $matrix2 [0]);
if ( $rows1 != $rows2 || $columns1 != $columns2 ) {
echo "Matrix dimension not Equal. Sum not possible" ;
}
else {
$sum = sumTwoMatrices( $matrix1 , $matrix2 );
foreach ( $sum as $row ) {
echo implode( "\t" , $row ) . "\n" ;
}
}
?>
|
Output
37 22 58
62 76 36
36 27 40
Share your thoughts in the comments
Please Login to comment...