Pagination Component using React Hooks
Last Updated :
01 Apr, 2024
Pagination on websites refers to the way of displaying a large set of data in smaller chunks. In React, it’s super easy to create a pagination component with the help of the `useEffect` and `useState` hooks. we will create a pagination component using React hooks.
Output Preview: Let us have a look at how the final output will look like.
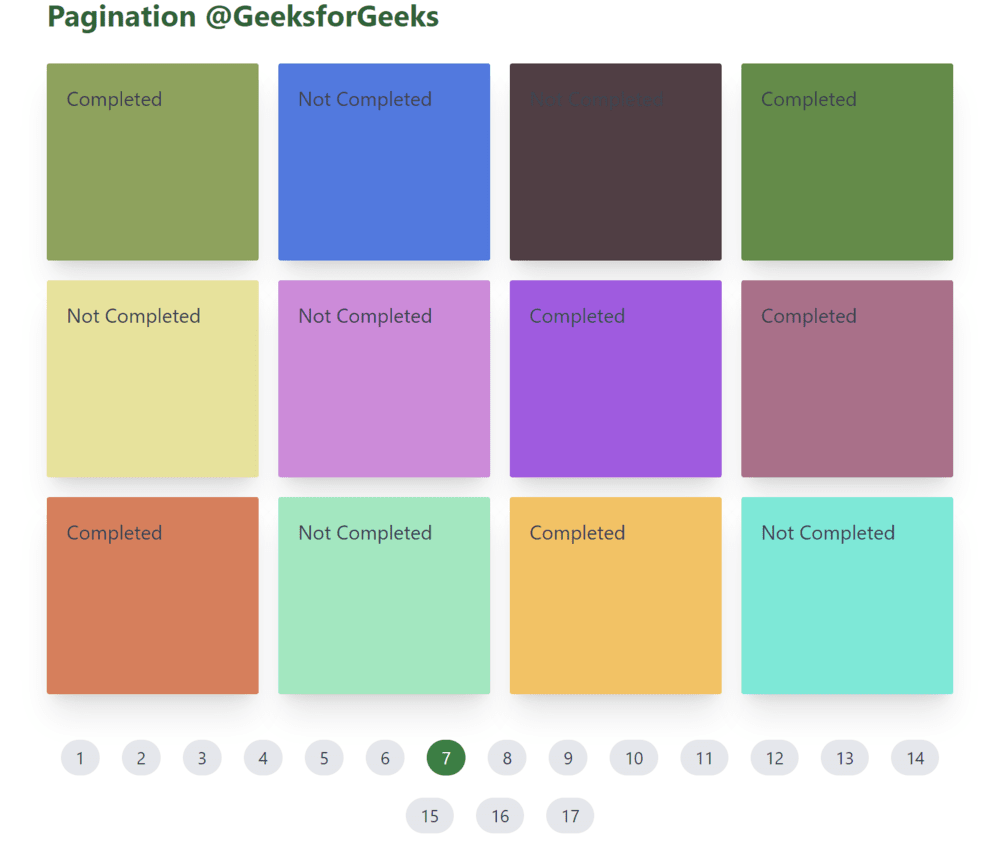
Prerequisites
Approach to create Pagination Component
The approach is very simple. We will count the array length and divide it with the number of elements that we wants to display per page. The resultant will be the total number of pages that will be rendered on a single page. We will utilize useState to store these results for conditional rendering.
Steps to Create React App & Install required modules
Step 1: Create a new react app and enter into the folder created by running the following command.
npx create-react-app react-pagination
cd react-pagination
Step 2: Install tailwindcss and initialize it by running the following command.
npm install tailwindcss
npx tailwindcss init
Step 3: Create a Paginatination Component to write the pagination logic.
Folder Structure:
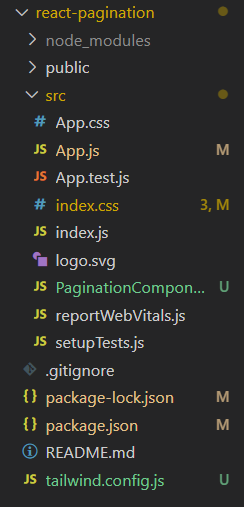
project structure
Dependencies List: Open package.json and verify the following dependencies.
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"tailwindcss": "^3.4.1",
"web-vitals": "^2.1.4"
}
Example Code: Here are the codes for working example of a pagination component with react hooks.
CSS
/* src/index.css */
@tailwind base;
@tailwind components;
@tailwind utilities;
* {
transition: 0.5s ease-in-out;
}
Javascript
// src/PaginationComponent.js
import React, { useState, useEffect } from 'react';
function PaginationComponent() {
const [todos, setTodos] = useState([]);
const [currentPage, setCurrentPage] = useState(1);
const [itemsPerPage] = useState(12);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(
`https://jsonplaceholder.typicode.com/todos`);
const data = await response.json();
setTodos(data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
}, []);
const randomLightColor = () => {
const randomColor = () => Math.floor(Math.
random() * 200) + 56;
return `rgb(${randomColor()},
${randomColor()}, ${randomColor()})`;
};
const indexOfLastItem = currentPage * itemsPerPage;
const indexOfFirstItem = indexOfLastItem - itemsPerPage;
const currentItems = todos.
slice(indexOfFirstItem, indexOfLastItem);
const paginate = (pageNumber) =>
setCurrentPage(pageNumber);
const pageNumbers = [];
for (let i = 1; i <= Math.ceil(todos.length /
itemsPerPage); i++) {
pageNumbers.push(i);
}
return (
<div className="max-w-3xl mx-auto p-4">
<h1 className="text-2xl text-green-800
font-bold mb-6">Pagination @GeeksforGeeks</h1>
<div className="grid grid-cols-4 gap-4">
{currentItems.map((todo) => (
<div key={todo.id}
className={`h-40 text-gray-800 p-4
rounded-sm shadow-xl`}
style={{ backgroundColor: randomLightColor()}}>
<h2 className="text-sm font-semibold mb-2">
{todo.title}
</h2>
<p className="text-gray-700
text-md">{todo.completed ?
'Completed': 'Not Completed'}</p>
</div>
))}
</div>
<ul className="flex gap-2 justify-center
flex-wrap mt-8">
{pageNumbers.map((number) => (
<li key={number} className="">
<button
onClick={() => paginate(number)}
className={`px-3 py-1 rounded-full
${currentPage === number
? 'bg-green-700 text-white'
: 'bg-gray-200 text-gray-700'
}`}
>
{number}
</button>
</li>
))}
</ul>
</div>
);
}
export default PaginationComponent;
Javascript
// src/App.js
import './App.css';
import PaginationComponent from './PaginationComponent';
function App() {
return (
<div className="App">
<PaginationComponent/>
</div>
);
}
export default App;
Javascript
// tailwind.config.js
/** @type {import('tailwindcss').Config} */
module.exports = {
content: ["./src/**/*.{html,js}"],
theme: {
extend: {},
},
plugins: [],
}
To Run the Application: Open terminal at the root of project and run the following command to start the server.
npm start
Output:
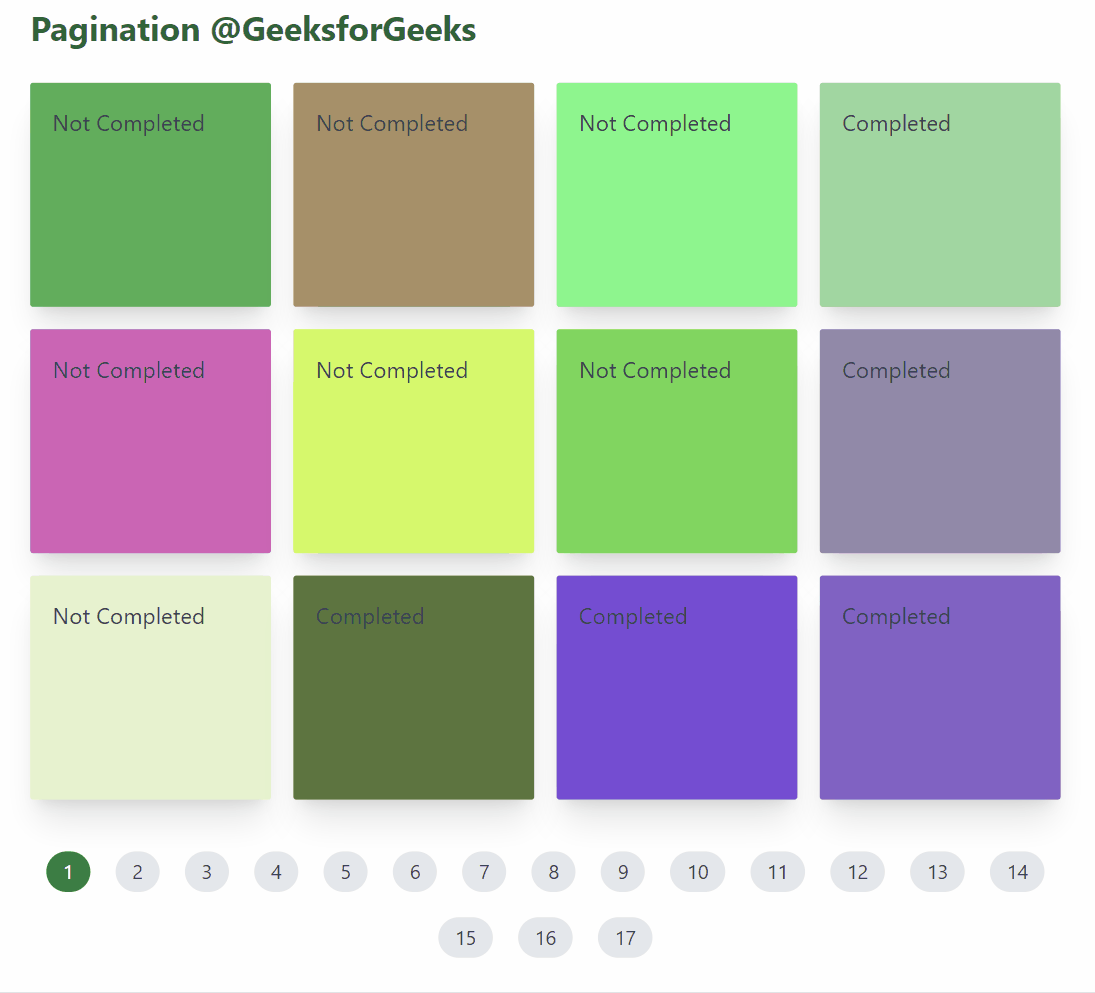
Share your thoughts in the comments
Please Login to comment...