Object-Oriented Programming System (OOPs) is a way of writing computer programs where we organize code into small, reusable pieces called objects. These objects represent things or concepts in the real world, like cars, animals, or people. Each object has its data and behaviors, and we can use them to build complex programs by connecting them. It’s like building with LEGO bricks, where each brick is a piece of code that we can snap together to create something bigger and more interesting.
What is Object-Oriented Programming (OOPs)?
Imagine you’re playing a video game with a bunch of characters. Each character has its special abilities, like strength, speed, and weapons. In OOP, these characters are called “objects.”
OOP is a way of organizing your code so that each object has its own data (like its name, age, and abilities) and its own actions (like attacking or defending). This makes your code easier to understand and change.
How do OOPs work?
To create an object, you need a “class.” A class is like a blueprint that defines what kind of data and actions an object will have. For example, you might have a class called “Hero” that defines the data and actions for all the heroes in your game.
Example:
Let’s imagine we’re playing with toy cars. Each toy car is an “object”. Now, think of a “class” as a toy car factory blueprint. This blueprint defines what every car that comes out of the factory will look like and what it can do.
The blueprint (class) might say that each car will have four wheels, a color, and the ability to move forward or backward. So, when we create a toy car (object) using this blueprint, we can decide its color, and we know it will have four wheels and can move.
Why use OOPs?
OOP has several advantages:
- Reusability: You can reuse objects in different parts of your code, which saves time and effort.
- Efficiency: OOP helps you organize your code in a way that makes it more efficient.
- Maintainability: OOP makes it easier to change and update your code over time.
Basic Concepts of OOPs:
Here are the basic concepts of Object-Oriented Programming (OOP) explained simply with examples in Python.
- Classes and Objects: Classes are like blueprints that describe how to make objects, which are like things you can see and touch in real life.
- Abstraction: Abstraction in programming is like using a TV remote without knowing its inner workings. It lets us focus on essential actions without needing to understand complex details, making code easier to use and understand.
- Encapsulation: Encapsulation is like putting things in a box and only letting certain people access them, so they stay safe and organized.
- Inheritance: Inheritance is like passing down traits from parents to children; objects can inherit characteristics and behaviors from other objects.
- Polymorphism: Polymorphism is like a superhero changing their powers based on the situation; objects can behave differently depending on the context.
What is Object in OOPs?
An Object can be anything that has attributes means characteristics and perform actions means methods. This world of objects is like superheroes with unique abilities. They have features, known as, suppose a car has attributes(characteristics) like color, size, and speed while the actions(methods) of the car can be starting the car or changing its gear.
What is Class in OOPS?
A Class defines the methods and attributes that an object must have and a Class is also known as a blueprint in Object- Oriented Programming. We will understand the concept of Class by taking an example of the “Cat” that is defined as “Class“. This Cat class has attributes that are characteristics such as age, breed, and name, along with methods that are actions like Meowing, sleeping, and eating. when we can produce an object from the class, we call it an instance. So, if we produce an instance of the Cat class called “Mate”, It must be having its own unique set of attributes and can perform the defined styles or we can say actions. A combination of similar types of objects is known as Class.
Example for Class and Object in OOPS:
Now, we will understand about class and object with the help of an example Imagine that we have a “Magic wand” in our hand and this wand can perform special tricks and has magical powers. Now we will talk about Class and Objects by using this Magical Wand. An Object is like an actual magic wand that has its abilities and characteristics. It can make things disappear, create sparks, or grant wishes.
Now let’s think of a magic school similar to Hogwarts School from Harry Potter where every wizard learns to use and handle these magical wands. In this Hogwarts school, there is one unique Classroom called “Wand” in which all these wands are created. We can think of this “Wand” Classroom as a “Class” that provides instructions on how to create these magical wands. A class is a blueprint or a set of instructions. So, in summary, A class is like an instruction or blueprint to create these magical wands, and an Object is an actual magical wand with its unique powers and abilities.
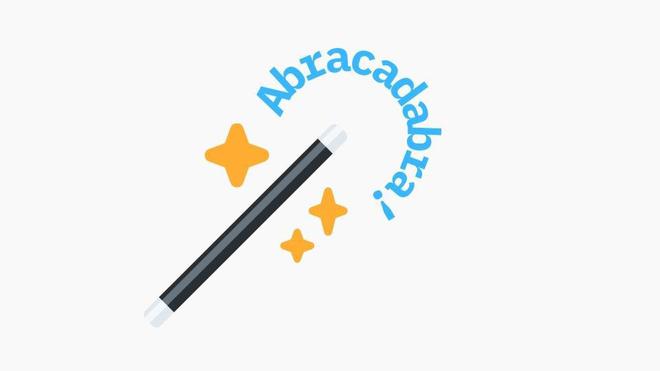
Example of Class and Object
Implementation of Class and Object Method:
In this, we create an object called ‘my-wand’ using the ‘Wand’ class. Objects and classes are like the magic wands and Hogwarts School. The class is where we define how a wand should be created, and the object is the actual wand we can use to perform magical spells. Just like in a magic school, we can create different wands with their colors and lengths, and each wand can perform its unique spells.
Python
class Wand:
def __init__(self, color, length):
self.color = color
self.length = length
def cast_spell(self):
print("Abracadabra! The wand casts a magical spell!")
# Create a wand object
my_wand = Wand("Purple", 10)
# Use the wand's method
my_wand.cast_spell()
OutputAbracadabra! The wand casts a magical spell!
Abstraction:
In simple words, Data abstraction ensures controlled data access and helps us to mainly focus on crucial information and ignore other complicated stuff of the code.
For example, if we take an example of Data Abstraction in terms of cars that means we are hiding the complex inner working of the car such as the car’s engine and other components of the car. We can think about Data abstraction like we are using a car but without knowing it’s other technical details.
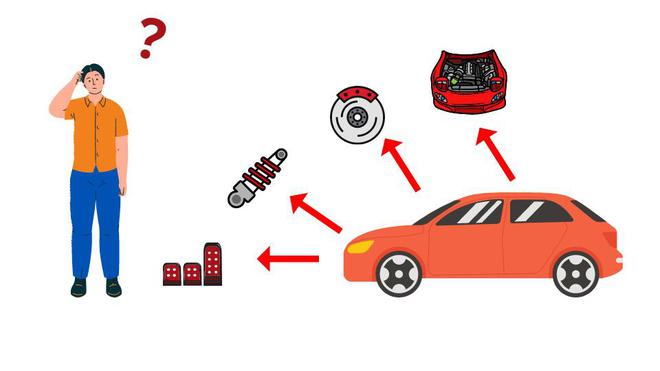
Example of Abstraction
Implementation of Abstraction Methods:
In this example, The “Car” class abstracts the inner workings of other systems and car engines. Users can start the car without needing to know about the inner technical details.
Python
class Car:
def __init__(self, brand, color):
self.brand = brand
self.color = color
def start(self):
print("The " + self.color + " " + self.brand + " car starts.")
def drive(self):
print("The " + self.color + " " + self.brand + " car is driving.")
my_car = Car("Tesla", "red")
my_car.start()
my_car.drive()
OutputThe red Tesla car starts.
The red Tesla car is driving.
In this example, The “Car” class abstracts the inner workings of other systems and car engines. Users can start the car without needing to know about the inner technical details.
Encapsulation:
If we talk about Encapsulation it means that we usually bind up the data and methods together and it only provides access through a public interface.
For example, In the context of cars, it means that we are bundling related data and functionality together, like putting a protective proper shield around a car.
Implementation of Encapsulation Methods:
In this example, the “Car” class encapsulates the brand, color, and fuel level of the car. The car can be refueled and driven but the fuel level is protected and only covert through defined methods.
Python
class Car:
def __init__(self, brand, color):
# Initialize car with brand, color, and full fuel tank (fuel_level = 100)
self.brand = brand
self.color = color
self.fuel_level = 100
def refuel(self):
# Refuel the car, setting the fuel level back to 100
self.fuel_level = 100
print("The " + self.color + " " + self.brand + " car has been refueled.")
def drive(self):
# Check if the car has fuel
if self.fuel_level > 0:
# If fuel level is greater than 0, drive the car and reduce fuel level by 10
self.fuel_level -= 10
print("The " + self.color + " " + self.brand + " car is driving.")
else:
# If out of fuel, print a message indicating so
print("The car is out of fuel!")
# Create a Car object
my_car = Car("Toyota", "blue")
# Refuel the car and drive it
my_car.refuel()
my_car.drive()
OutputThe blue Toyota car has been refueled.
The blue Toyota car is driving.
In this example, the “Car” class encapsulates the brand, color, and fuel level of the car. The car can be refueled and driven but the fuel level is protected and only covert through defined methods.
Inheritance:
In OOPS, It’s just the same as passing down family traits from one generation to the next. We can create new classes, known as subclasses that inherit attributes and methods from a parent class, known as a superclass.
For Example, We again take the context of the car means we are creating a unique car with a specialization of classes that inherit similar and common properties and behaviors from a base car class. It permits us to define different car types with their unique features.
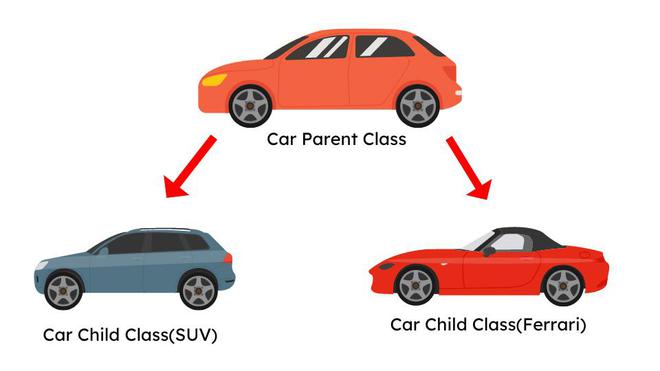
Example of Inheritance
Implementation of Inheritance Methods:
In this example, the”Car” class is the base class from which the “SportsCar” and “SUV” classes inherit. Each subclass has its unique features and behaviors while inheriting the common “start” method from the base class.
Python
class Car:
def __init__(self, brand, color):
self.brand = brand
self.color = color
def start(self):
print("The " + self.color + " " + self.brand + " car starts.")
class SportsCar(Car):
def accelerate(self):
print("The " + self.color + " " + self.brand + " sports car accelerates quickly.")
class SUV(Car):
def off_road(self):
print("The " + self.color + " " + self.brand + " SUV tackles rough terrains with ease.")
my_sports_car = SportsCar("Ferrari", "red")
my_sports_car.start()
my_sports_car.accelerate()
my_suv = SUV("Jeep", "black")
my_suv.start()
my_suv.off_road()
OutputThe red Ferrari car starts.
The red Ferrari sports car accelerates quickly.
The black Jeep car starts.
The black Jeep SUV tackles rough terrains with ease.
Polymorphism:
In the magical world of OOP, Objects can take on different forms. Polymorphism enables us to use objects of different subclasses interchangeably if they share a common superclass. It’s like having different disguises but the same superpower!
For example, In this context there are cars means that we are treating different car objects as if they were the same type, based on a similar interface. It allows us to use different car types interchangeably.
-660.jpg)
Example of Polymorphism
Implementation of Polymorphism Methods:
In this, the “Car” class defines the standard interface with the” drive” method. The “Sedan” and “Convertible” Classes inherit from “Car” and provide their implementation of the drive method. We can store both types of cars in a list and iterate over them, treating them as Car objects and calling the drive method on each of them.
Python
class Car:
def __init__(self, brand, color):
self.brand = brand
self.color = color
def drive(self):
# Raise NotImplementedError if drive method is not implemented in subclass
raise NotImplementedError(
"drive method must be implemented in each car subclass")
class Sedan(Car):
def drive(self):
# Override drive method for Sedan subclass
print("The", self.color, self.brand, "sedan is driving smoothly.")
class Convertible(Car):
def drive(self):
# Override drive method for Convertible subclass
print("The", self.color, self.brand,
"convertible is driving with the top down.")
# Create instances of Sedan and Convertible
my_sedan = Sedan("Honda", "silver")
my_convertible = Convertible("BMW", "blue")
cars = [my_sedan, my_convertible]
# Iterate through cars list and call drive method for each car
for car in cars:
car.drive()
Output('The', 'silver', 'Honda', 'sedan is driving smoothly.')
('The', 'blue', 'BMW', 'convertible is driving with the top down.')
Benefits of OOPS:
- Organization: OOPS helps keep code neat and tidy by organizing it into reusable blocks called “objects.”
- Creativity: Kids can create objects with their own special features and actions, making programming more fun and creative.
- Modularity: OOPS breaks big projects into smaller parts, making them easier to understand and work on.
- Reusability: Once kids create objects, they can use them again and again in different parts of their projects.
- Collaboration: OOPS allows kids to work together on coding projects by dividing tasks into separate objects.
Applications of OOPS:
- Games: Kids can create their own video games using OOPS, with objects representing characters, obstacles, and game features.
- Apps: OOPS can be used to build apps for smartphones and tablets, where each app feature is represented by an object.
- Robots: OOPS helps kids program robots by defining objects for different robot actions like moving, sensing, and interacting with the environment.
- Websites: OOPS can also be used to build websites, where objects represent different web elements like buttons, forms, and menus.
Conclusion:
Great! You had embarked into the incredible world of OOPs (Object Oriented programming structure) and uncovered its amazing magical secrets. Learning and Understanding the concepts of inheritance, data abstraction, polymorphism, objects, and classes is essential, as they form the foundation of any programming language. Hope your Coding journey is filled with wonder, excitement, and the joy of creating something truly magical!
OOPs(Object Oriented Programming) – FAQs
1. What are the 4 methods in OOPS?
The 4 methods of OOPS are Inheritance, Abstraction, Encapsulation and Polymorphism.
2. Who is the father of OOPS?
Alan Kay is known to be father of OOPS.
3. Is OOPS a language?
No, Object oriented programming is not a language, it is a programming approach which can be used in different coding or programming languages.
4. Which language is considered to be the first OOPS language?
Simula is considered to be the first OOPS language.
5. What is the size of a class?
Difference of the upper limit and the lower limit of a class interval is called size of class.
Share your thoughts in the comments
Please Login to comment...