NPM React Router Dom
Last Updated :
09 May, 2024
React Router DOM is a powerful routing library for React applications that enables navigation and URL routing. In this article, we’ll explore React Router DOM in-depth, covering its installation, basic usage, advanced features, and best practices.
What is React Router DOM?
React Router DOM is a collection of navigational components for React applications that allows developers to declaratively define the application’s UI based on the URL. It provides a way to synchronize the UI with the URL, enabling client-side routing in single-page applications (SPAs).
Steps to Implement React-router-dom
Step 1: Create a React application using the following command:
npx create-react-app myapp
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd myapp
Project Structure:
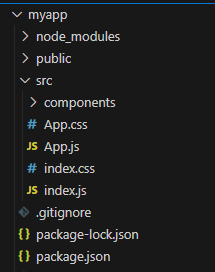
Step 3: Next, install react-router-dom using the following command
npm install react-router-dom
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.3",
"react-scripts": "5.0.1",
},
Basic Usage
React Router DOM provides several components for defining routes in your application:
- BrowserRouter: Wraps your application with a router component that listens to changes in the URL.
- Router: Alias for BrowserRouter. (This is used for renaming convenience, if desired.)
- Routes: Container for defining routes in your application.
- Route: Defines a route that renders a component based on the current URL path.
Example:
JavaScript
// App.js
import {
BrowserRouter as Router,
Routes, Route
} from 'react-router-dom';
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
<Routes>
<Route path="/about" element={<About />} />
<Route path="/" element={<Home />} />
</Routes>
</div>
</Router>
);
}
export default App;
Output:
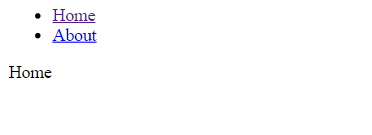
Conclusion
React Router DOM, available via npm, simplifies client-side routing in React applications. It provides essential components like BrowserRouter, Router, Routes, and Route, facilitating URL-based navigation and rendering components based on URL paths. With its intuitive API, React Router DOM enables seamless navigation and state management, enhancing the user experience in single-page applications.
Share your thoughts in the comments
Please Login to comment...