Mouse swipe controls in Angular 9 using HammerJS
Last Updated :
19 Aug, 2020
Angular is an application design framework and development platform for creating efficient and sophisticated single-page apps. It has been changed a lot from its first release. And it keeps adding new features and modifications in its releases which is a good thing. But sometimes what we have used in the previous version stops working in the latest version. Same is the case with HammerJS.
HammerJS is a very good open-source library that can recognise gestures made by touch, mouse and pointer events. You can read more about HammerJS and its documentation here.
In Angular 9, if you use previous methods of adding HammerJS, it will not work because Angular has modified some of its features. So you will go through the whole process of working with HammerJS in Angular 9 from starting.
Approach:
The approach is to install the hammerjs package locally, import it in main.ts and set the Hammer gesture configuration by extending the HammerGestureConfig class. Then you can bind to specific events like swipe, pan, pinch, press, etc. The most important thing is to import the HammerModule in app module file.
Example and Explanation: Swipe Gesture
-
In your angular project, install the hammerjs package locally by running the below command.
npm install --save hammerjs
-
Now, you will need to import the hammerjs module in your main.ts file. If you do not import this, you will get an error in your console. Error: Hammer.js is not loaded, can not bind to XYZ event.
import 'hammerjs';
-
Let us move on to our app.module.ts, here you can add your own configuration of Hammer gestures using HammerGestureConfig class and HAMMER_GESTURE_CONFIG like in the below image.
Also make sure to import HammerModule as it is the current modification which has been done in Angular 9.
Otherwise your project will not work and will not give error too. ( Although this is in typescript, but the editor does not support that yet so ignore and do not get confused. )
app.module.ts
import { NgModule, Injectable } from '@angular/core' ;
import { BrowserModule } from '@angular/platform-browser' ;
import { FormsModule } from '@angular/forms' ;
import * as Hammer from 'hammerjs' ;
import {
HammerModule, HammerGestureConfig, HAMMER_GESTURE_CONFIG}
from '@angular/platform-browser' ;
import { AppComponent } from './app.component' ;
@Injectable()
export class MyHammerConfig extends HammerGestureConfig {
overrides = <any> {
swipe: { direction: Hammer.DIRECTION_ALL },
};
}
@NgModule({
imports: [ BrowserModule, FormsModule, HammerModule ],
declarations: [ AppComponent, HelloComponent ],
bootstrap: [ AppComponent ],
providers: [
{
provide: HAMMER_GESTURE_CONFIG,
useClass: MyHammerConfig,
},
],
})
export class AppModule { }
|
- Now we will create our simple example for Swipe gestures. For app.component.html, you can add the following code.
app.component.html
< div class = "swipe" (swipe)="onSwipe($event)">
< h1 >Swipe Gesture</ h1 >
< p >Works with both mouse and touch.</ p >
< h5 [innerHTML]="direction"></ h5 >
</ div >
|
- Add some styles to your example like this in app.component.css. The important thing to notice is where you want the swipe gesture, set user-select as none.
app.component.css
.swipe {
background-color : #76b490 ;
padding : 20px ;
margin : 10px ;
border-radius: 3px ;
height : 500px ;
text-align : center ;
overflow : auto ;
color : rgb ( 78 , 22 , 131 );
user-select: none ;
}
h 1 ,
p {
color : rgb ( 116 , 49 , 11 );
}
|
- Lastly, add your typescript code in app.component.ts like this.
import { Component } from "@angular/core" ;
@Component({
selector: "my-app" ,
templateUrl: "./app.component.html" ,
styleUrls: [ "./app.component.css" ]
})
export class AppComponent {
direction = "" ;
onSwipe(event) {
const x =
Math.abs(
event.deltaX) > 40 ? (event.deltaX > 0 ? "Right" : "Left" ) : "" ;
const y =
Math.abs(
event.deltaY) > 40 ? (event.deltaY > 0 ? "Down" : "Up" ) : "" ;
this .direction +=
`You swiped in <b> ${x} ${y} </b> direction <hr>`;
}
}
|
Output:
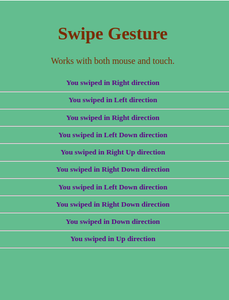
There are several other gestures you can implement using HammerJS just like that. For more information, please read their documentation.
Share your thoughts in the comments
Please Login to comment...