Minimum cost to connect all cities
Last Updated :
06 Jan, 2023
There are n cities and there are roads in between some of the cities. Somehow all the roads are damaged simultaneously. We have to repair the roads to connect the cities again. There is a fixed cost to repair a particular road. Find out the minimum cost to connect all the cities by repairing roads. Input is in matrix(city) form, if city[i][j] = 0 then there is not any road between city i and city j, if city[i][j] = a > 0 then the cost to rebuild the path between city i and city j is a. Print out the minimum cost to connect all the cities.
It is sure that all the cities were connected before the roads were damaged.
Examples:
Input : {{0, 1, 2, 3, 4},
{1, 0, 5, 0, 7},
{2, 5, 0, 6, 0},
{3, 0, 6, 0, 0},
{4, 7, 0, 0, 0}};
Output : 10
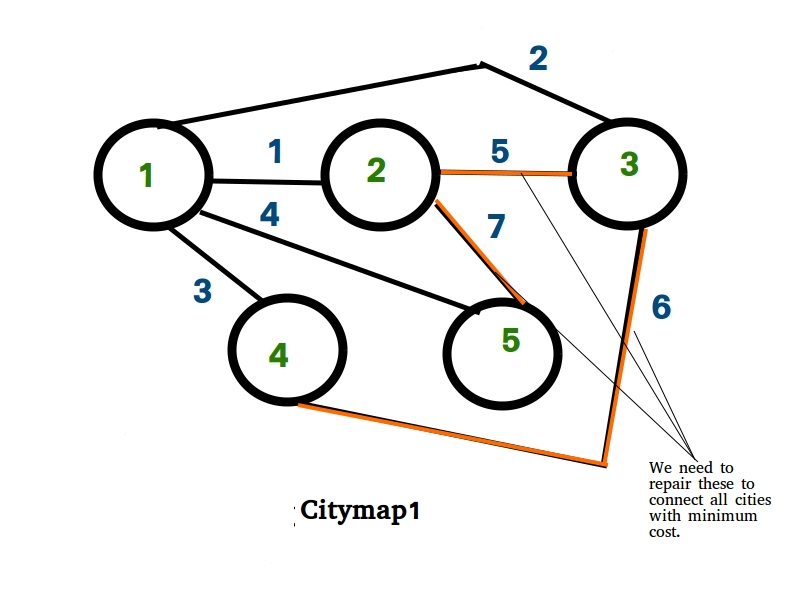
Input : {{0, 1, 1, 100, 0, 0},
{1, 0, 1, 0, 0, 0},
{1, 1, 0, 0, 0, 0},
{100, 0, 0, 0, 2, 2},
{0, 0, 0, 2, 0, 2},
{0, 0, 0, 2, 2, 0}};
Output : 106
Method: Here we have to connect all the cities by path which will cost us least. The way to do that is to find out the Minimum Spanning Tree(MST) of the map of the cities(i.e. each city is a node of the graph and all the damaged roads between cities are edges). And the total cost is the addition of the path edge values in the Minimum Spanning Tree.
Prerequisite: MST Prim’s Algorithm
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
int minnode( int n, int keyval[], bool mstset[]) {
int mini = numeric_limits< int >::max();
int mini_index;
for ( int i = 0; i < n; i++) {
if (mstset[i] == false && keyval[i] < mini) {
mini = keyval[i], mini_index = i;
}
}
return mini_index;
}
void findcost( int n, vector<vector< int >> city) {
int parent[n];
int keyval[n];
bool mstset[n];
for ( int i = 0; i < n; i++) {
keyval[i] = numeric_limits< int >::max();
mstset[i] = false ;
}
parent[0] = -1;
keyval[0] = 0;
for ( int i = 0; i < n - 1; i++) {
int u = minnode(n, keyval, mstset);
mstset[u] = true ;
for ( int v = 0; v < n; v++) {
if (city[u][v] && mstset[v] == false &&
city[u][v] < keyval[v]) {
keyval[v] = city[u][v];
parent[v] = u;
}
}
}
int cost = 0;
for ( int i = 1; i < n; i++)
cost += city[parent[i]][i];
cout << cost << endl;
}
int main() {
int n1 = 5;
vector<vector< int >> city1 = {{0, 1, 2, 3, 4},
{1, 0, 5, 0, 7},
{2, 5, 0, 6, 0},
{3, 0, 6, 0, 0},
{4, 7, 0, 0, 0}};
findcost(n1, city1);
int n2 = 6;
vector<vector< int >> city2 = {{0, 1, 1, 100, 0, 0},
{1, 0, 1, 0, 0, 0},
{1, 1, 0, 0, 0, 0},
{100, 0, 0, 0, 2, 2},
{0, 0, 0, 2, 0, 2},
{0, 0, 0, 2, 2, 0}};
findcost(n2, city2);
return 0;
}
|
Java
import java.util.*;
class GFG{
static int minnode( int n, int keyval[],
boolean mstset[])
{
int mini = Integer.MAX_VALUE;
int mini_index = 0 ;
for ( int i = 0 ; i < n; i++)
{
if (mstset[i] == false &&
keyval[i] < mini)
{
mini = keyval[i];
mini_index = i;
}
}
return mini_index;
}
static void findcost( int n, int city[][])
{
int parent[] = new int [n];
int keyval[] = new int [n];
boolean mstset[] = new boolean [n];
for ( int i = 0 ; i < n; i++)
{
keyval[i] = Integer.MAX_VALUE;
mstset[i] = false ;
}
parent[ 0 ] = - 1 ;
keyval[ 0 ] = 0 ;
for ( int i = 0 ; i < n - 1 ; i++)
{
int u = minnode(n, keyval, mstset);
mstset[u] = true ;
for ( int v = 0 ; v < n; v++)
{
if (city[u][v] > 0 &&
mstset[v] == false &&
city[u][v] < keyval[v])
{
keyval[v] = city[u][v];
parent[v] = u;
}
}
}
int cost = 0 ;
for ( int i = 1 ; i < n; i++)
cost += city[parent[i]][i];
System.out.println(cost);
}
public static void main(String args[])
{
int n1 = 5 ;
int city1[][] = { { 0 , 1 , 2 , 3 , 4 },
{ 1 , 0 , 5 , 0 , 7 },
{ 2 , 5 , 0 , 6 , 0 },
{ 3 , 0 , 6 , 0 , 0 },
{ 4 , 7 , 0 , 0 , 0 } };
findcost(n1, city1);
int n2 = 6 ;
int city2[][] = { { 0 , 1 , 1 , 100 , 0 , 0 },
{ 1 , 0 , 1 , 0 , 0 , 0 },
{ 1 , 1 , 0 , 0 , 0 , 0 },
{ 100 , 0 , 0 , 0 , 2 , 2 },
{ 0 , 0 , 0 , 2 , 0 , 2 },
{ 0 , 0 , 0 , 2 , 2 , 0 } };
findcost(n2, city2);
}
}
|
Python3
def minnode(n, keyval, mstset):
mini = 999999999999
mini_index = None
for i in range (n):
if (mstset[i] = = False and
keyval[i] < mini):
mini = keyval[i]
mini_index = i
return mini_index
def findcost(n, city):
parent = [ None ] * n
keyval = [ None ] * n
mstset = [ None ] * n
for i in range (n):
keyval[i] = 9999999999999
mstset[i] = False
parent[ 0 ] = - 1
keyval[ 0 ] = 0
for i in range (n - 1 ):
u = minnode(n, keyval, mstset)
mstset[u] = True
for v in range (n):
if (city[u][v] and mstset[v] = = False and
city[u][v] < keyval[v]):
keyval[v] = city[u][v]
parent[v] = u
cost = 0
for i in range ( 1 , n):
cost + = city[parent[i]][i]
print (cost)
if __name__ = = '__main__' :
n1 = 5
city1 = [[ 0 , 1 , 2 , 3 , 4 ],
[ 1 , 0 , 5 , 0 , 7 ],
[ 2 , 5 , 0 , 6 , 0 ],
[ 3 , 0 , 6 , 0 , 0 ],
[ 4 , 7 , 0 , 0 , 0 ]]
findcost(n1, city1)
n2 = 6
city2 = [[ 0 , 1 , 1 , 100 , 0 , 0 ],
[ 1 , 0 , 1 , 0 , 0 , 0 ],
[ 1 , 1 , 0 , 0 , 0 , 0 ],
[ 100 , 0 , 0 , 0 , 2 , 2 ],
[ 0 , 0 , 0 , 2 , 0 , 2 ],
[ 0 , 0 , 0 , 2 , 2 , 0 ]]
findcost(n2, city2)
|
C#
using System;
class GFG{
static int minnode( int n, int [] keyval,
bool [] mstset)
{
int mini = Int32.MaxValue;
int mini_index = 0;
for ( int i = 0; i < n; i++)
{
if (mstset[i] == false && keyval[i] < mini)
{
mini = keyval[i];
mini_index = i;
}
}
return mini_index;
}
static void findcost( int n, int [,] city)
{
int [] parent = new int [n];
int [] keyval = new int [n];
bool [] mstset = new bool [n];
for ( int i = 0; i < n; i++)
{
keyval[i] = Int32.MaxValue;
mstset[i] = false ;
}
parent[0] = -1;
keyval[0] = 0;
for ( int i = 0; i < n - 1; i++)
{
int u = minnode(n, keyval, mstset);
mstset[u] = true ;
for ( int v = 0; v < n; v++)
{
if (city[u, v] > 0 && mstset[v] == false &&
city[u, v] < keyval[v])
{
keyval[v] = city[u, v];
parent[v] = u;
}
}
}
int cost = 0;
for ( int i = 1; i < n; i++)
cost += city[parent[i], i];
Console.WriteLine(cost);
}
public static void Main( string [] args)
{
int n1 = 5;
int [,] city1 = { { 0, 1, 2, 3, 4 },
{ 1, 0, 5, 0, 7 },
{ 2, 5, 0, 6, 0 },
{ 3, 0, 6, 0, 0 },
{ 4, 7, 0, 0, 0 } };
findcost(n1, city1);
int n2 = 6;
int [,] city2 = { { 0, 1, 1, 100, 0, 0 },
{ 1, 0, 1, 0, 0, 0 },
{ 1, 1, 0, 0, 0, 0 },
{ 100, 0, 0, 0, 2, 2 },
{ 0, 0, 0, 2, 0, 2 },
{ 0, 0, 0, 2, 2, 0 } };
findcost(n2, city2);
}
}
|
Javascript
<script>
function minnode(n, keyval,
mstset)
{
let mini = Number.MAX_VALUE;
let mini_index = 0;
for (let i = 0; i < n; i++)
{
if (mstset[i] == false &&
keyval[i] < mini)
{
mini = keyval[i];
mini_index = i;
}
}
return mini_index;
}
function findcost(n, city)
{
let parent = Array(n).fill(0);
let keyval = Array(n).fill(0);
let mstset = Array(n).fill(0);
for (let i = 0; i < n; i++)
{
keyval[i] = Number.MAX_VALUE;
mstset[i] = false ;
}
parent[0] = -1;
keyval[0] = 0;
for (let i = 0; i < n - 1; i++)
{
let u = minnode(n, keyval, mstset);
mstset[u] = true ;
for (let v = 0; v < n; v++)
{
if (city[u][v] > 0 &&
mstset[v] == false &&
city[u][v] < keyval[v])
{
keyval[v] = city[u][v];
parent[v] = u;
}
}
}
let cost = 0;
for (let i = 1; i < n; i++)
cost += city[parent[i]][i];
document.write(cost + "<br/>" ) ;
}
let n1 = 5;
let city1 = [[ 0, 1, 2, 3, 4 ],
[ 1, 0, 5, 0, 7 ],
[ 2, 5, 0, 6, 0 ],
[ 3, 0, 6, 0, 0 ],
[ 4, 7, 0, 0, 0 ]];
findcost(n1, city1);
let n2 = 6;
let city2 = [[ 0, 1, 1, 100, 0, 0 ],
[ 1, 0, 1, 0, 0, 0 ],
[ 1, 1, 0, 0, 0, 0 ],
[ 100, 0, 0, 0, 2, 2 ],
[ 0, 0, 0, 2, 0, 2 ],
[ 0, 0, 0, 2, 2, 0 ]];
findcost(n2, city2);
</script>
|
Time Complexity: The outer loop(i.e. the loop to add new node to MST) runs n times and in each iteration of the loop it takes O(n) time to find the min node and O(n) time to update the neighboring nodes of u-th node. Hence the overall complexity is O(n2)
Auxiliary Space: O(n)
Share your thoughts in the comments
Please Login to comment...