MaterialApp class in Flutter
Last Updated :
07 Mar, 2023
MaterialApp Class: MaterialApp is a predefined class or widget in a flutter. It is likely the main or core component of a flutter app. The MaterialApp widget provides a wrapper around other Material Widgets. We can access all the other components and widgets provided by Flutter SDK. Text widget, DropdownButton widget, AppBar widget, Scaffold widget, ListView widget, StatelessWidget, StatefulWidget, IconButton widget, TextField widget, Padding widget, ThemeData widget, etc. are the widgets that can be accessed using MaterialApp class. There are many more widgets that are accessed using MaterialApp class. Using this widget, we can make an attractive app that follows the Material Design guidelines.
Here is the constructor of MaterialApp class:
Constructor of MaterialApp class
const MaterialApp(
{Key key,
GlobalKey<NavigatorState> navigatorKey,
Widget home,
Map<String, WidgetBuilder> routes: const <String, WidgetBuilder>{},
String initialRoute,
RouteFactory onGenerateRoute,
InitialRouteListFactory onGenerateInitialRoutes,
RouteFactory onUnknownRoute,
List<NavigatorObserver> navigatorObservers: const <NavigatorObserver>[],
TransitionBuilder builder,
String title: '',
GenerateAppTitle onGenerateTitle,
Color color,
ThemeData theme,
ThemeData darkTheme,
ThemeData highContrastTheme,
ThemeData highContrastDarkTheme,
ThemeMode themeMode: ThemeMode.system,
Locale locale,
Iterable<LocalizationsDelegate> localizationsDelegates,
LocaleListResolutionCallback localeListResolutionCallback,
LocaleResolutionCallback localeResolutionCallback,
Iterable<Locale> supportedLocales: const <Locale>[Locale('en', 'US')],
bool debugShowMaterialGrid: false,
bool showPerformanceOverlay: false,
bool checkerboardRasterCacheImages: false,
bool checkerboardOffscreenLayers: false,
bool showSemanticsDebugger: false,
bool debugShowCheckedModeBanner: true,
Map<LogicalKeySet, Intent> shortcuts,
Map<Type, Action<Intent>> actions}
)
Properties of MaterialApp widget:
- action: This property takes in Map<Type, Action<Intent>> as the object. It controls intent keys.
- backButtonDispatcher: It decided how to handle the back button.
- checkerboardRasterCacheImage: This property takes in a boolean as the object. If set to true it turns on the checkerboarding of raster cache images.
- color: It controls the primary color used in the application.
- darkTheme: It provided theme data for the dark theme for the application.
- debugShowCheckedModeBanner: This property takes in a boolean as the object to decide whether to show the debug banner or not.
- debugShowMaterialGird: This property takes a boolean as the object. If set to true it paints a baseline grid material app.
- highContrastDarkTheme: It provided the theme data to use for the high contrast theme.
- home: This property takes in widget as the object to show on the default route of the app.
- initialRoute: This property takes in a string as the object to give the name of the first route in which the navigator is built.
- locale: It provides a locale for the MaterialApp.
- localizationsDelegate: This provides a delegate for the locales.
- navigatorObserver: It takes in GlobalKey<NavigatorState> as the object to generate a key when building a navigator.
- navigatorObserver: This property holds List<NavigatorObserver> as the object to create a list of observers for the navigator.
- onGenerateInitialRoutes: This property takes in InitialRouteListFactory typedef as the object to generate initial routes.
- onGeneratRoute: The onGenerateRoute takes in a RouteFactory as the object. It is used when the app is navigated to a named route.
- onGenerateTitle: This property takes in RouteFactory typedef as the object to generate a title string for the application if provided.
- onUnknownRoute: The onUnknownRoute takes in RouteFactory typedef as the object to provide a route in case of failure in other metheod.
- routeInformationParse: This property holds RouteInformationParser<T> as the object to the routing information from the routeInformationProvider into a generic data type.
- routeInformationProvider: This property takes in RouteInformationProvider class as the object. It is responsible for providing routing information.
- routeDelegate: This property takes in RouterDelegate<T> as the object to configure a given widget.
- routes: The routes property takes in LogicalKeySet class as the object to control the app’s topmost level routing.
- shortcuts: This property takes in LogicalKeySet class as the object to decide the keyboard shortcut for the application.
- showPerformanceOverlay: The showPerformanceOverlay takes in a boolean value as the object to turn on or off performance overlay.
- showSemantisDebugger: This property takes in a boolean as the object. If set to true, it shows some accessible information.
- supportedLocales: The supportedLocales property keeps hold of the locals used in the app by taking in Iterable<E> class as the object.
- theme: This property takes in ThemeData class as the object to describe the theme for the MaterialApp.
- themeMode: This property holds ThemeMode enum as the object to decide the theme for the material app.
- title: The title property takes in a string as the object to decide the one-line description of the app for the device.
Simple Material App Example:
Here is very simple code in dart language to make a screen that has an AppBar title as GeeksforGeeks.
Dart
import 'package:flutter/material.dart' ;
void main() {
runApp( const GFGapp());
}
class GFGapp extends StatelessWidget {
const GFGapp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'GeeksforGeeks' ,
theme: ThemeData(primarySwatch: Colors.green),
darkTheme: ThemeData(primarySwatch: Colors.grey),
color: Colors.amberAccent,
supportedLocales: { const Locale( 'en' , ' ' )},
debugShowCheckedModeBanner: false ,
home: Scaffold(
appBar: AppBar(title: const Text( 'GeeksforGeeks' )),
),
);
}
}
|
Output:
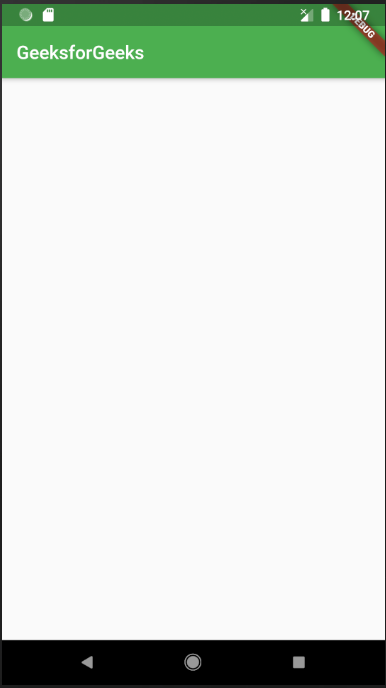
- Here we can see that the text defined in the title of the AppBar is displayed at the top.
- The default theme color is green as we defined.
- runApp() calls the widget GFGapp that returns the MaterialApp widget which specifies theme, localization, title, home widget and more.
Output explanation:
- import statement: The import statement is used to import the libraries that are provided by the flutter SDK. Here we have imported the ‘material.dart’ file. We can use all the flutter widgets that implement the material design by importing this file.
- main() function: Like many other programming languages, we also have a main function in which we have to write the statements that are to be executed when the app starts. The return type of the main function is ‘void’.
- runApp(Widget widget) function: The void runApp(Widget widget) takes a widget as an argument and sets it on a screen. It gives the constraints to the widget to fit into the screen. It makes the given widget the root widget of the app and other widgets as the child of it. Here we have used the MaterialApp as a root widget in which we have defined the other widgets.
- MaterialApp() widget: We have discussed MaterialApp in the beginning. Let us have a look at the different properties of the MaterialApp widget.
- title: This property is used to provide a short description of the application to the user. When the user presses the recent apps button on mobile the text proceeded in titlthe e is displayed.
- theme: This property is used to provide the default theme to the application like the theme color of the application.
For this, we use the inbuilt class/widget named ThemeData(). In Themedata() widget we have to write the different properties related to the theme. Here we have used the primarySwatch which is used to define the default themecolor of the application. To choose the color we used Colors class from the material library. In ThemeData() we can also define some other properties like TextTheme, Brightness(Can enable dark theme by this), AppBarTheme, and many more.
- home: It is used for the default route of the app means the widget defined in it is displayed when the application starts normally. Here we have defined the Scaffold widget inside the home property. Inside the Scaffold we define various properties like appBar, body, floatingActionButton, backgroundColor, etc.
For example, in the appBar property we have used the AppBar() widget which accepts ‘GeeksforGeeks’ as the title, this will be displayed at the top of the application in appbar.
- The other properties in MaterialApp() are debugShowCheckedModeBanner (used to remove the debug tag at the top corner), darkTheme (To request dark mode in application), color (For the primary color of application), routes (For routing table of application), ThemeMode (To determine which theme to be used) and supportedLocales contains a list of languages the app supports, etc.
Conclusion
In this article we looked at MaterialApp widget. It wraps all other Material widgets such as Scaffold, AppBar, Drawer, etc. You can create an app with Material Design Specifications using the MaterialApp widget. We also looked at the constructor and properties of the MaterialApp widget in order to understand what we can achieve using the MaterialApp widget in Flutter.
Share your thoughts in the comments
Please Login to comment...