JUnit 5 – Test Suites with Example
Last Updated :
09 Feb, 2024
JUnit 5 encourages a modular approach to test creation with its test suites. These suites function as containers, bundling multiple test classes for easier management and collective execution within a single run. In this article, we will learn about JUnit 5 – Test Suites.
Test Suite
In JUnit 5, a test suite is a way to combine multiple test classes and run them together as a single unit. The @Suite annotation on the test class is used to define a test suite. You can also include specific test classes in the suite using annotations like @SelectClasses, @SelectPackages, or @SelectClasspathRoots, that help to filter out the test classes you want to run together. Now we go through an example, to execute a test suite.
Example of JUnit 5 – Test Suites
Here we take a Java program that has methods add() and subtract() to perform addition and subtraction of two numbers. We have written test classes for both methods separately as AdditionTest and SubtractionTest. Now we can run both of them collectively as a single unit, under a test suite CalculatorTestSuite which is annotated by @Suite, and then @SelectClasses which includes both our test classes.
Calculator.java File:
Java
public class Calculator {
public static int add( int a, int b) {
return a + b;
}
public static int subtract( int a, int b) {
return a - b;
}
}
|
AdditionTest.java File:
Java
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
class AdditionTest {
@Test
void testAddition() {
Calculator calculator = new Calculator();
assertEquals( 5 , calculator.add( 2 , 3 ));
}
}
|
SubtractionTest.java File:
Java
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
class SubtractionTest {
@Test
void testSubtraction()
{
Calculator calculator = new Calculator();
assertEquals( 2 , calculator.subtract( 5 , 3 ));
}
}
|
CalculatorTestSuite.java File:
Java
import org.junit.platform.suite.api.SelectClasses;
import org.junit.platform.suite.api.Suite;
@Suite
@SelectClasses ({AdditionTest. class , SubtractionTest. class })
public class CalculatorTestSuite {
}
|
Output:
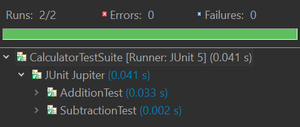
Output
Share your thoughts in the comments
Please Login to comment...