JUnit 5 – @AfterAll Annotation with Example
Last Updated :
17 Dec, 2023
JUnit 5 is a widely used testing framework in the Java ecosystem. It is the successor of JUnit 4 and is designed to address its limitations. JUnit framework allows the developers to write and run the tests for their Java code. These tests help ensure that the code functions correctly and continues to work as expected as changes are made. JUnit 5 provides a variety of annotations and one such annotation is `@AfterAll`. In this article, let us understand about @AfterAll annotation in JUnit 5.
@AfterAll in JUnit 5
@AfterAll annotation in JUnit 5 is used to mark a method that should run once after all the test methods in a test class have been executed. This annotation is typically used to perform cleanup or teardown operations. We will see the demonstration with a sample project below.
Prerequisites for the Topic
To understand this, we need to have some prerequisites. They are:
- It is essential to have knowledge of at least Java version 8.
- Understanding of build management tools like Maven or Gradle.
- One should be proficient in using a Java Integrated Development Environment (IDE) such as Eclipse, IntelliJ IDEA, or Visual Studio Code.
IDE Setup
Here, we are using Eclipse IDE for Java and Web Developers 2023-06. You may also use other platforms like IntelliJ, Spring suite tool, Spring Initializer, etc.
Step By Step Implementation
Step 1: Creation of Project
- Go to the `file` menu and click new and navigate to `Spring Starters project`. If you don’t find the Spring Starters project immediately after `new`, then click other and find `Spring Starters project`.
- File > new > Spring Starters project.
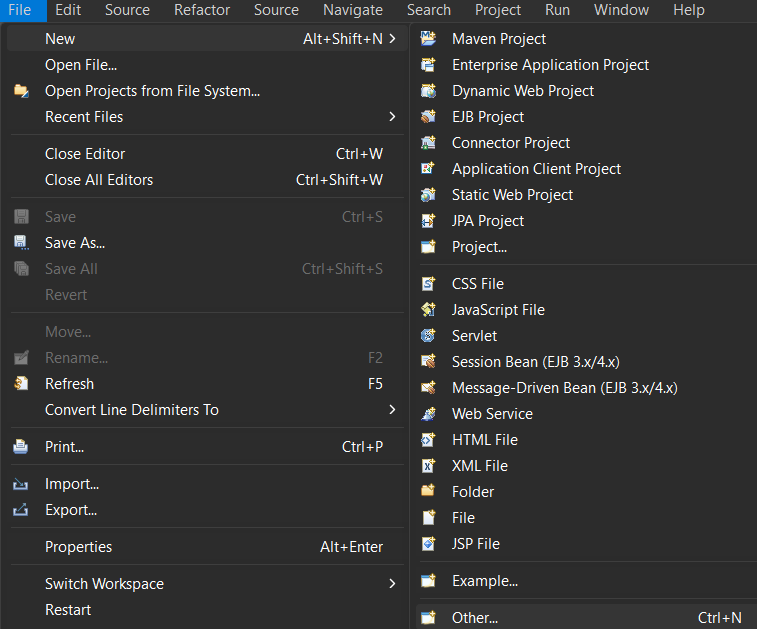
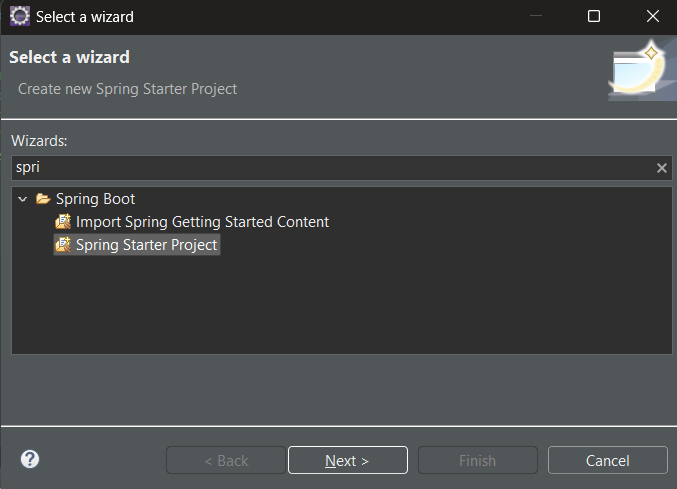
- Name your project and configure the default options given if necessary.
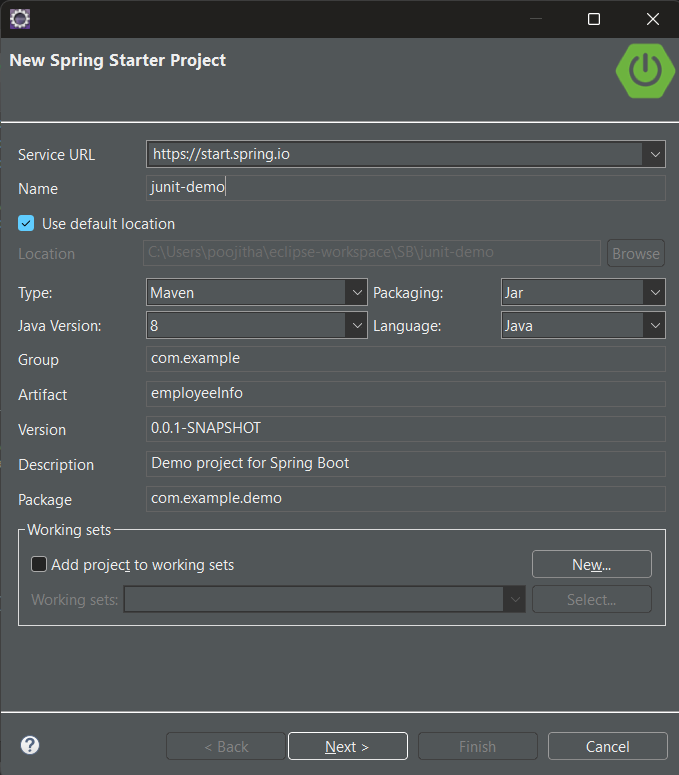
Type: Maven
Packaging: Jar
Java Version: 8
Language: Java
- Make sure that, you have chosen the type as ‘Maven‘ and the version of Java should be at least 8.
- Add dependencies If you need any, otherwise, click `finish`.
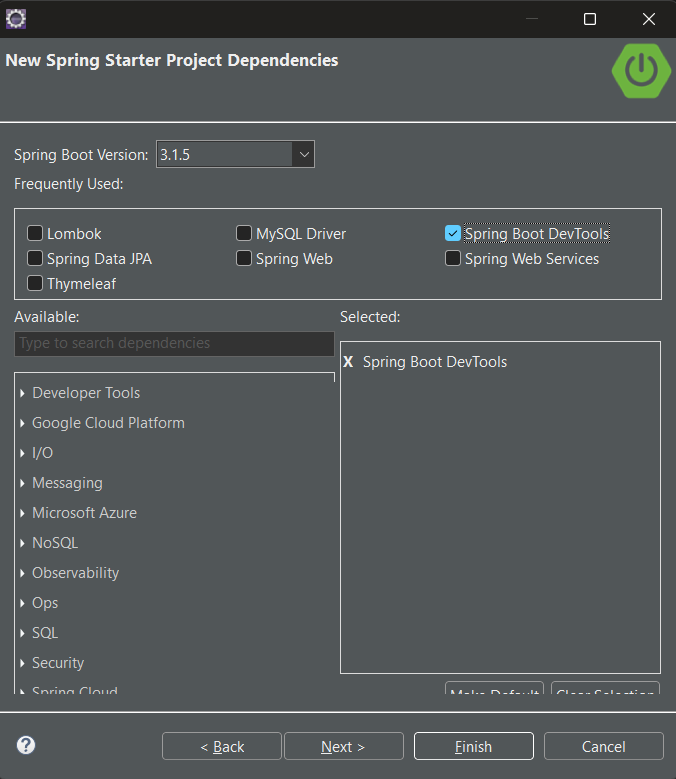
Step 2: Adding dependencies
Let us configure our pom.xml file with the following dependencies:
JUnit Jupiter API:
The JUnit Jupiter API is the part of JUnit 5 framework which provides annotations, assertions, and other features for defining and running test cases and serves as a programming model for writing tests in Java. To add the JUnit Jupiter API in pom.xml file, copy and paste the following code.
XML
< dependency >
< groupId >org.junit.jupiter</ groupId >
< artifactId >junit-jupiter-api</ artifactId >
< version >5.8.0</ version >
< scope >test</ scope >
</ dependency >
|
Project Setup
Now, that the project setup is ready, let us look into an example to understand how to write and run test cases of JUnit using the Maven tool.
Step 1: Create a new package in the `src/test/java`, right-click on `src/test/java` > new > package.
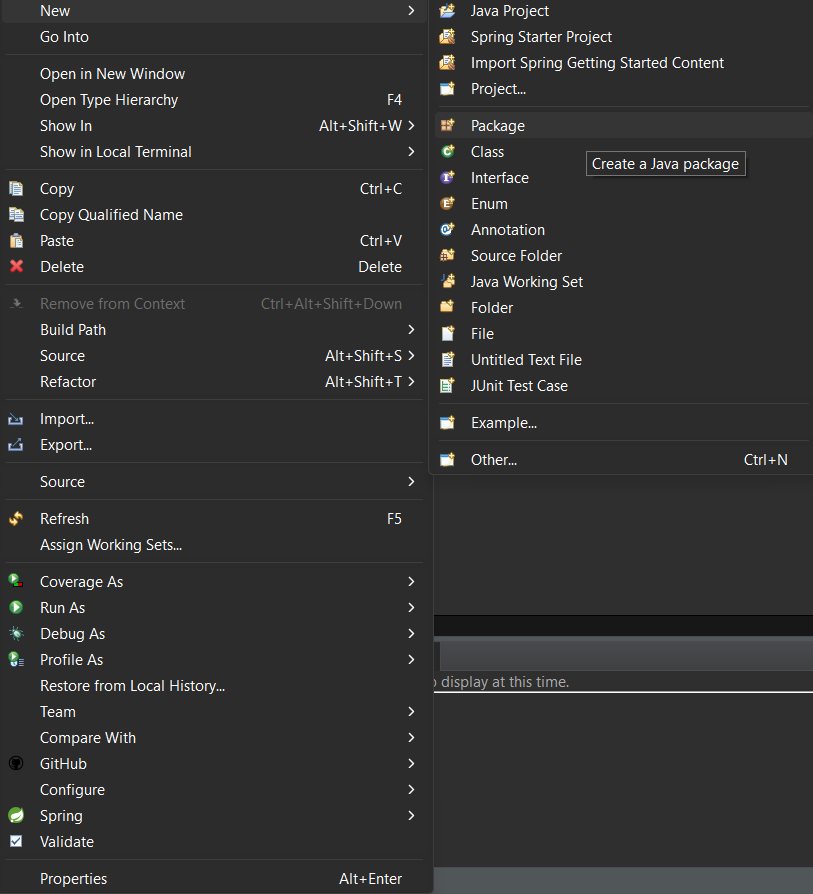
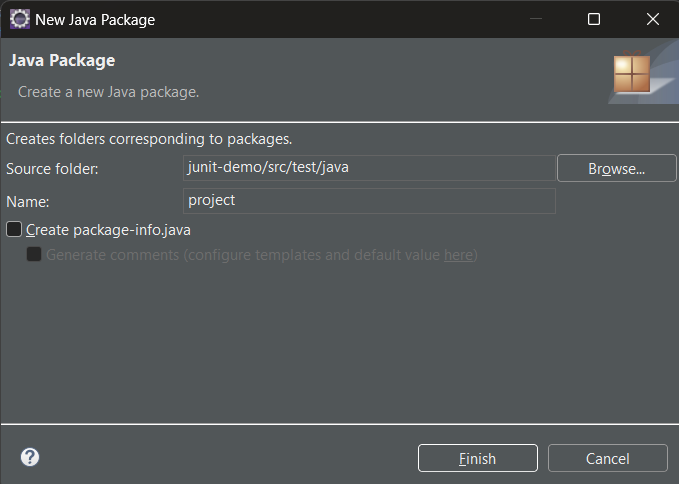
Step 2: Create a class in the package and name it as per your requirement. i.e. `Addition`. To create a class, right-click on package > new > class
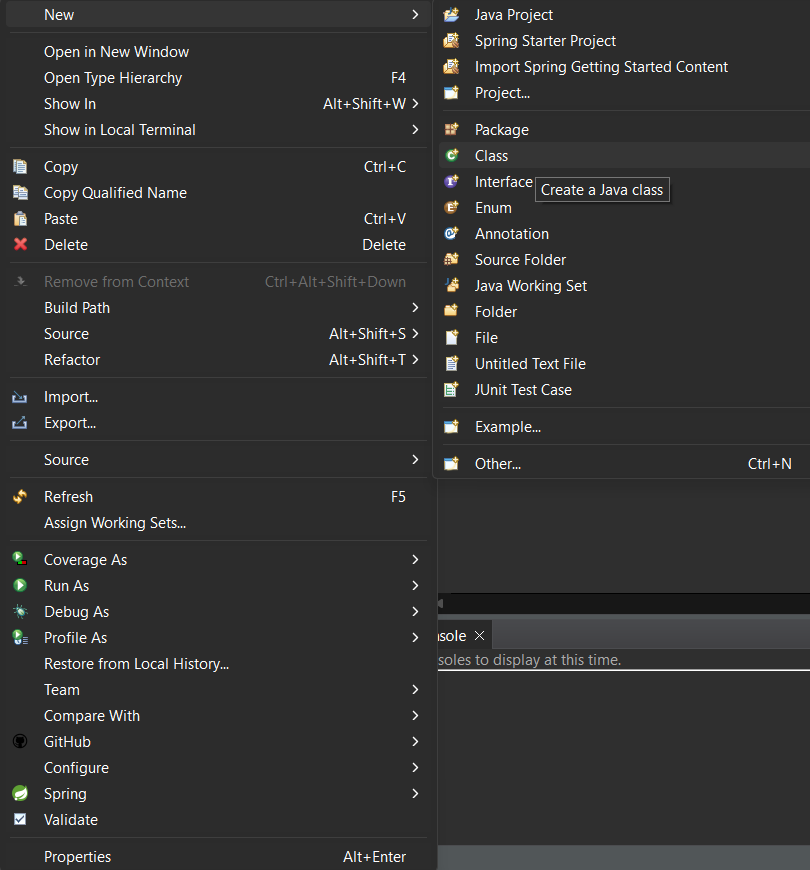
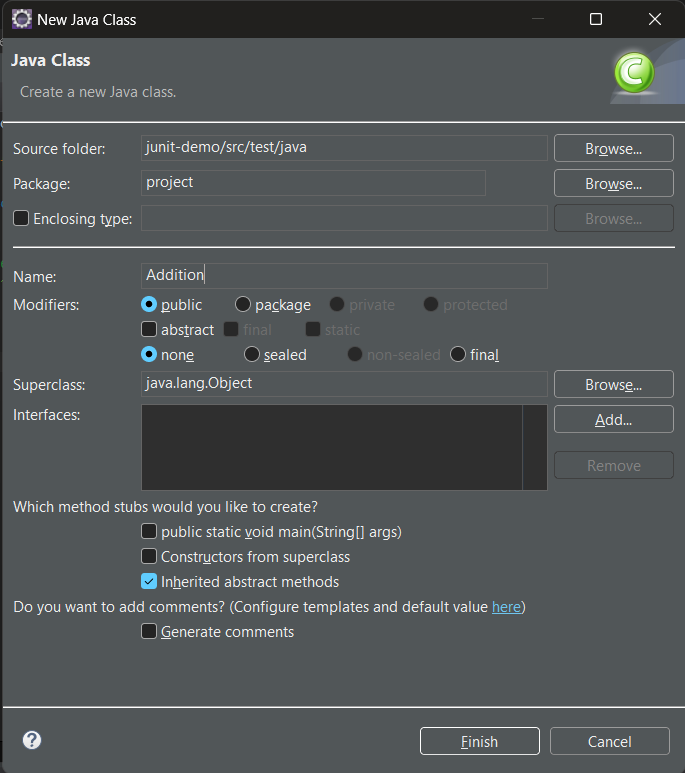
Step 3: write the logic which you want to test in `Addition.java`.
Java
package project;
public class Addition {
public int sum( int a, int b) {
return a+b;
}
}
|
Step 4: Now, create a test case inside the package by right click on the package > new > others > java > JUnit > JUnit test case
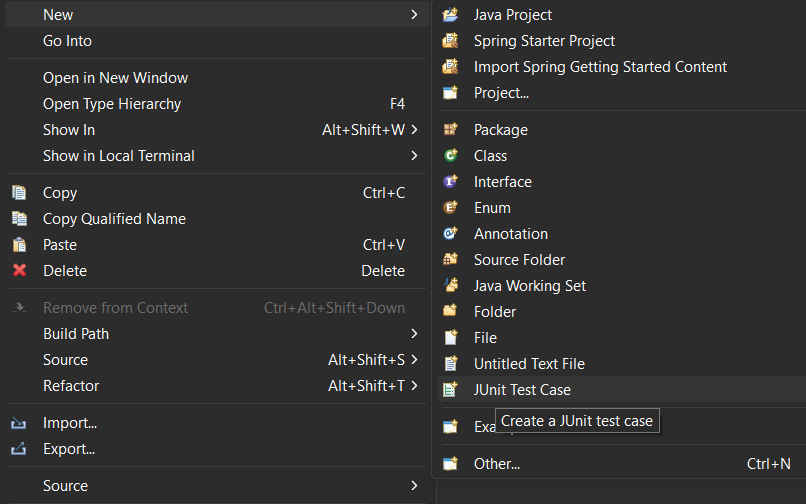
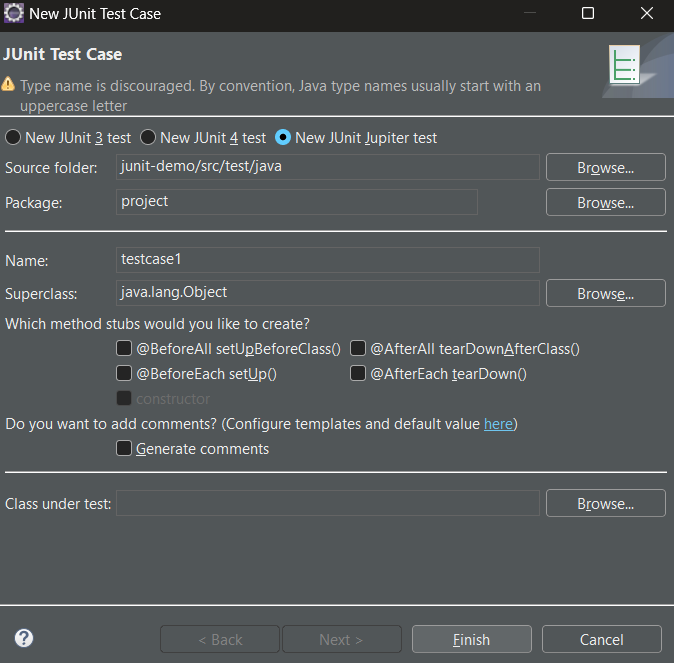
Example of JUnit 5 – @AfterAll Annotation
Test Script: Let us write the first test case i.e. `testcase1`.
Java
package project;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.Test;
import static org.junit.Assert.assertEquals;
public class testcase1 {
@Test
void test1() {
Addition addition= new Addition();
int actualResult=addition.sum( 10 , 20 );
int expectedResult= 30 ;
assertEquals(actualResult, expectedResult);
}
@Test
void test2() {
Addition addition= new Addition();
int actualResult=addition.sum( 10 , 20 );
int expectedResult= 40 ;
assertEquals(actualResult, expectedResult);
}
@AfterAll
static void cleanUp() {
System.out.println( "Cleanup after all tests" );
}
}
|
Steps to run the test case:
To run the test case, right-click on the file click on the run as option, and select JUnit test.

We can see the output of the test case in the JUnit tab of the terminal.
Output:
Even though the result of the tests are success or fail, irrespective of the status of tests, the method which is annotated with @Afterall is executed.
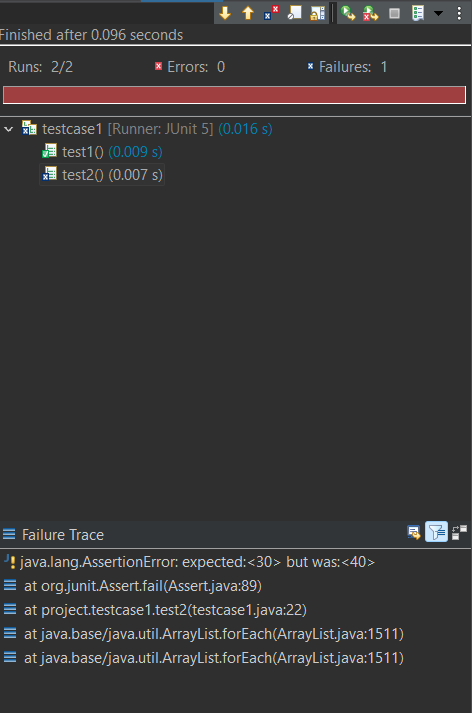
The above output represents that in the defined two tests, there is a failure test and the Failure trace of the test2 is provided.

The above output represent the console view in the terminal, which refers to the successful execution of cleanUp method after the execution of all the tests.
Explanation of the above Application:
In the above script, we have created two tests namely `test1` method and `test2` method annotated with `@Test`. Followed by that we have defined `cleanUp` method annotated with `@AfterAll`, which refers that the `cleanUp` method will be executed only after execution of the tests.
Share your thoughts in the comments
Please Login to comment...