JUnit 5 – Test Reports in HTML
Last Updated :
03 Jan, 2024
In this article, we will discuss how to view Test reports of JUnit 5 in HTML formats. By default, JUnit 5 produces test reports in the form of XML files but understanding XML files directly is not possible and we will need some other third-party tool to parse the XML and give us the information about the test results from JUnit 5.
Generating and viewing Test reports in HTML format is very user-friendly to understand and use the test reports for understanding our test outputs. For creating JUnit 5 test reports in HTML format, we will use a maven plugin called “maven-surefire-report-plugin“. This reporting plugin will parse the test output from JUnit 5 and create an HTML site for us in the “target/site” directory to view it in our browser without much hassle.
Prerequisite
- Java
- JUnit 5
- Eclipse IDE and Maven
Project Structure
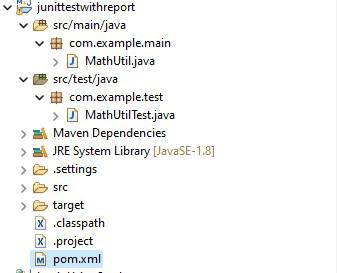
Creating Test Reports in HTML
To create test reports in HTML format for JUnit 5 tests, add the below reporting plugin in your `pom.xml`. The maven-surefire-reporting-plugin will take the reports from “target/surefire-reports” generated by JUnit 5. By default, surefire reports will normally contain a text file and an XML file as our test report. But gathering data from XML and text files from surefire report is not viable, so we will use the reporting plugin to analyze the report and create a HTML site for us.
Step 1: Create a Maven Project
- Open the Eclipse IDE and create a basic Maven project.
- In the project pom.xml, add the below JUnit 5 dependencies and reporting plugin in your pom.xml file.
- This reporting plugin will generate the HTML reports of JUnit 5.
XML
< modelVersion >4.0.0</ modelVersion >
< groupId >com.example</ groupId >
< artifactId >junittestwithreport</ artifactId >
< version >0.0.1-SNAPSHOT</ version >
< packaging >jar</ packaging >
< name >junittestwithreport</ name >
< properties >
< project.build.sourceEncoding >UTF-8</ project.build.sourceEncoding >
</ properties >
< dependencies >
< dependency >
< groupId >org.junit.jupiter</ groupId >
< artifactId >junit-jupiter</ artifactId >
< version >5.10.1</ version >
</ dependency >
</ dependencies >
< build >
< plugins >
< plugin >
< groupId >org.apache.maven.plugins</ groupId >
< artifactId >maven-compiler-plugin</ artifactId >
< version >3.11.0</ version >
</ plugin >
< plugin >
< groupId >org.apache.maven.plugins</ groupId >
< artifactId >maven-site-plugin</ artifactId >
< version >4.0.0-M12</ version >
</ plugin >
</ plugins >
</ build >
< reporting >
< plugins >
< plugin >
< groupId >org.apache.maven.plugins</ groupId >
< artifactId >maven-surefire-report-plugin</ artifactId >
< version >3.2.2</ version >
</ plugin >
</ plugins >
</ reporting >
</ project >
|
Now we have added the reporting plugin for the JUnit 5 testing project.
Step 2: Create a Utility class and a Test class
- In the src/main/java package, create a Java class that will provide a simple function so that it can be tested in JUnit 5.
- We will name it as MathUtil as it is going to provide a function to check a number is prime or not.
- Create the MathUtil class inside a package and add the below code.
Java
package com.example.main;
public class MathUtil {
public static boolean isPrime( int n)
{
for ( int val = 2 ; val * val <= n; val++) {
if (n % val == 0 )
return false ;
}
return n > 1 ;
}
}
|
- Write a simple JUnit testcase to test the above utility class.
- Create a MathUtilTest class in the src/test/java/ directory.
- Write some JUnit test methods to test the isPrime function of MathUtil class.
Java
package com.example.test;
import static org.junit.jupiter.api.Assertions.assertFalse;
import static org.junit.jupiter.api.Assertions.assertTrue;
import com.example.main.MathUtil;
import org.junit.jupiter.api.Test;
public class MathUtilTest {
@Test public void testAPrime()
{
assertTrue(MathUtil.isPrime( 11 ));
}
@Test public void testEvenNumberIsPrime()
{
assertFalse(MathUtil.isPrime( 22 ));
}
@Test public void testNotPrime()
{
assertFalse(MathUtil.isPrime( 80 ));
}
}
|
Step 3: Generating Test reports in HTML
Using Maven site Plugin:
mvn site
- The maven site plugin will generate more reports like dependency, plugins, summary along with Project test report.
- It also looks more modern than the HTML output of Maven Surefire Report Plugin.
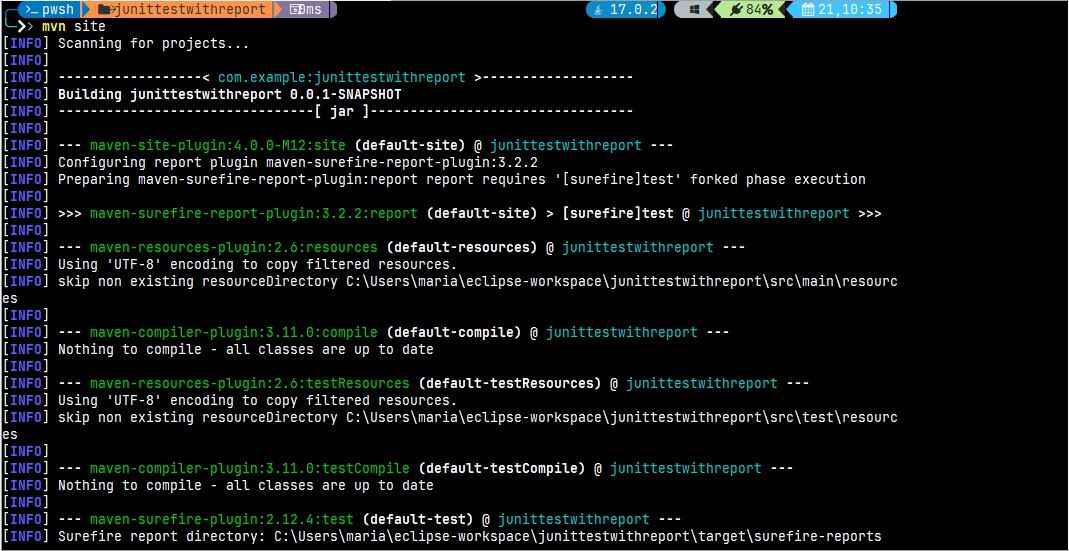
Using Maven Surefire Report Plugin:
mvn surefire-report:report
- The surefire report will only generate the test reports in HTML no other information will be included like the Maven site.
- Both of the plugin will compile and test the project before generating the report, so you won’t need to run mvn test and then proceed with the above commands.
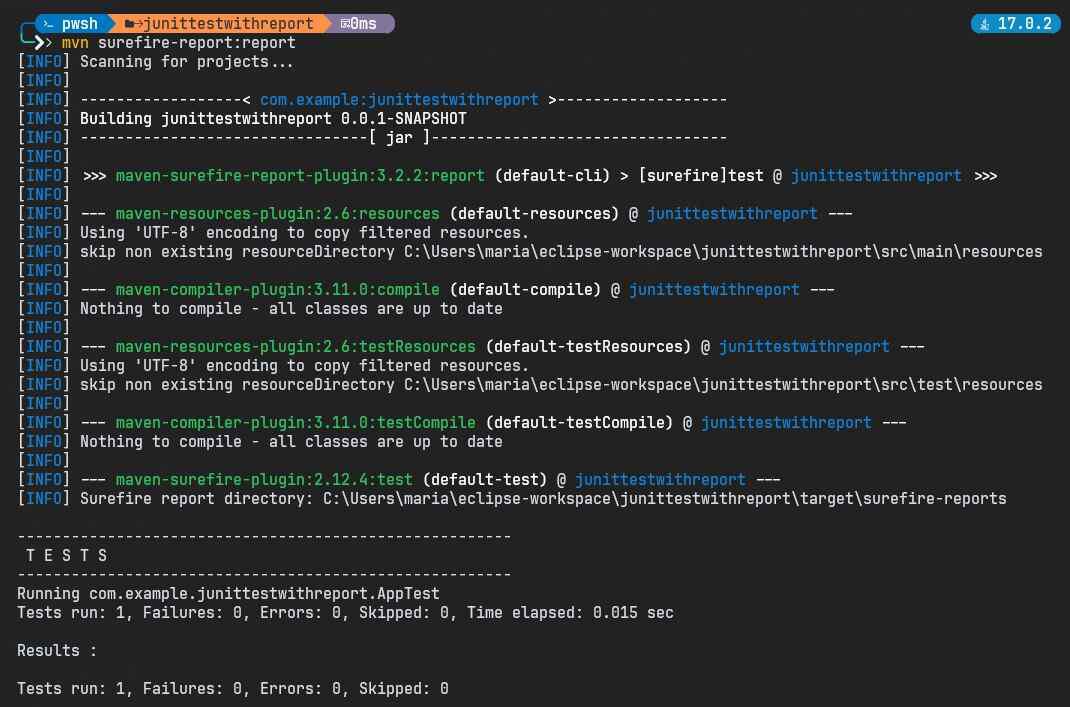
Step 4: Viewing the HTML test reports.
- Once the above command run is done, the report will be saved under “target/site” directory of the project.
- The maven generated site for the project will be the index.html and the test reports will be found in surefire-report.html
Output:
The below output shows the procedure of testing and viewing the JUnit 5 test reports in HTML using Maven site plugin.
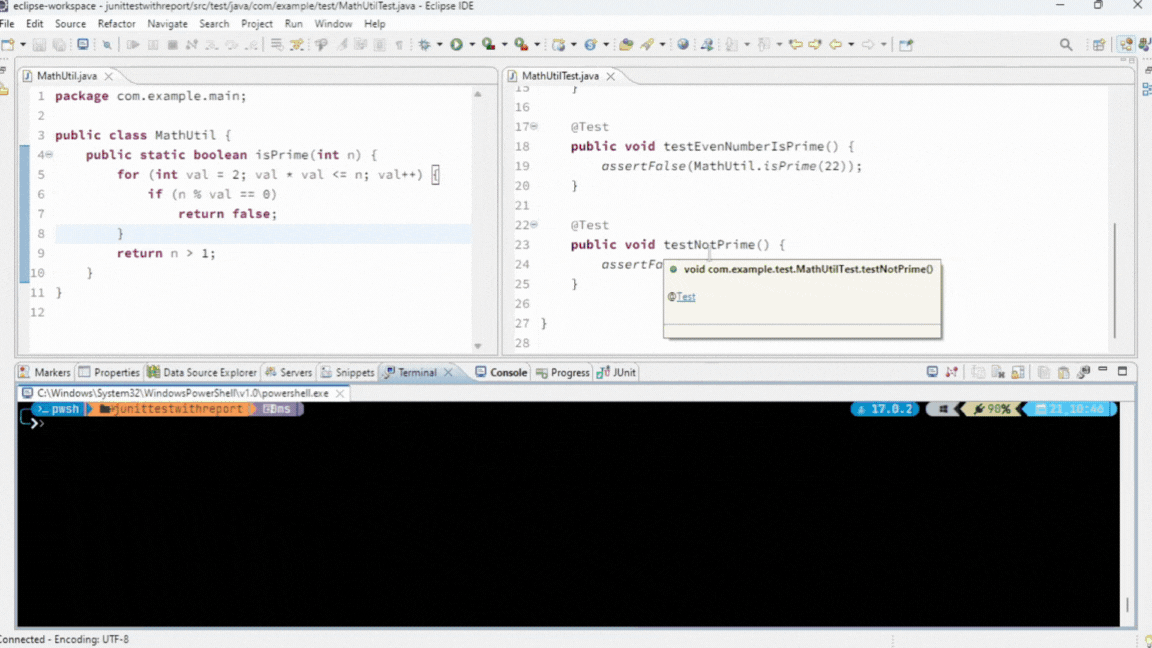
The below image is a sample output of Maven surefire-report plugin, this looks slightly outdated but its an option too if you just need to view the Test reports of JUnit 5.
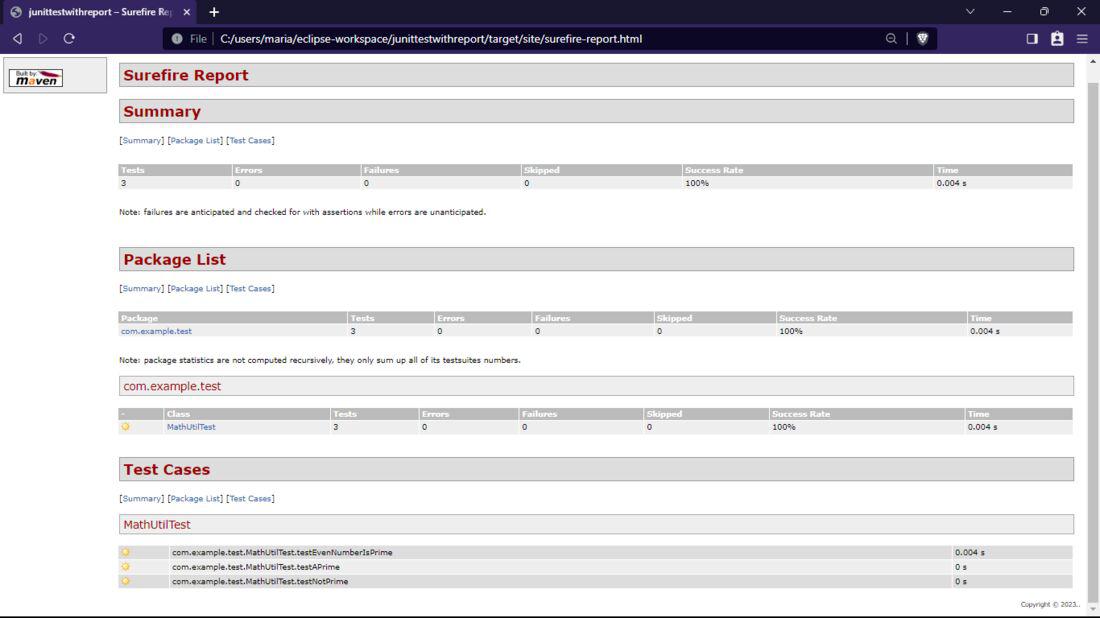
Conclusion
With that we have seen how we can create JUnit 5 Test Reports in HTML with maven-surefire-report-plugin. Add the plugin to your Java developer tool set to highly increase your productivity.
Share your thoughts in the comments
Please Login to comment...