JUnit 5 – @TempDir
Last Updated :
05 Feb, 2024
Junit 5 is a Unit testing framework for the Java programming language. It is a simple framework for writing tests for Java codes using Java. Whenever we write code, we have to test it. Junit is the primary source for writing the test cases. the latest version of Junit is Junit 5 Jupiter.
@TempDir in JUnit 5
@TempDir is an annotation that is used to annotate the fields in a test class of a test method of the type of path or file that should be resolved to the temporary directory, it is introduced in junit5 for the management of temporary directories in the test scenarios. This annotation allows programmers and developers to declare the parameter in the test method. If we use the annotation JUnit should automatically create a temporary directory and provide the path of the directory during the execution of the Annotated method.
@TempDir annotation handles the temporary file-related operations and ensures a clean and simplified environment for each test case.
Now let us understand the steps involved in implementing the Junit 5 @TempDir annotation.
Steps by step Implementation of @TempDir
Step 1: Import Dependencies
To implement the Junit 5 test cases, we need to add the necessary dependencies in to the project. we need junit-jupiter dependency to run implement Junit 5 -@TempDir. We can get the required dependencies from MavenRepository from Google.
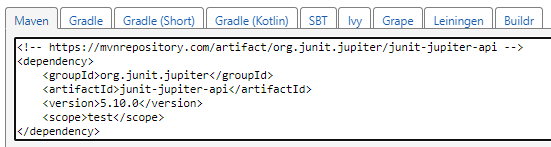
The dependency that we have seen we need to add into the respective project based on the type of the project whether it is a maven project of a gradle project.
Note: You can get the required repositories from MavenRepository website.
JUnit 5 – @TempDir Example
Example1: A simple example to understand how to use @TempDir in JUnit 5 test class.
Java
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.io.TempDir;
import java.io.File;
import java.io.IOException;
import java.nio.file.Path;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class Example1 {
@Test
void testUsingTempDir( @TempDir Path tempDir) throws IOException {
File tempFile = new File(tempDir.toFile(), "example1.txt" );
assertTrue(tempFile.createNewFile());
}
}
|
Explanation of above program:
In the above example program the @TempDir annotation is used to inject a temporary directory (Path tempDir) to the test method. the test creates a file within the temporary directory, and the assertTrue() statement checks whether the file was successfully created or not.
Example2: Program to demonstrate the use of @TempDir with multiple parameters in a JUnit 5 test class.
Java
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.io.TempDir;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class Example2 {
@Test
void testWithMultipleTempDirs( @TempDir Path tempDir1, @TempDir Path tempDir2) throws IOException {
Path file1 = tempDir1.resolve( "file1.txt" );
Files.createFile(file1);
assertTrue(Files.exists(file1));
Path file2 = tempDir2.resolve( "file2.txt" );
Files.createFile(file2);
assertTrue(Files.exists(file2));
}
}
|
Explanation of above program:
In the above example we have declared two parameters, tempDir1, tempDir2 of type path, Now JUnit 5 automatically creates the temporary directories for tempDir1 and tempDir2 before testWithMultipleTempDirs method executed.
Each temporary directory tempDir1 and tempDir2 creates the files file1.txt and file2.txt. After the completion of JUnit 5 test method the temporary directories will clean up automatically.
Note: The parameters for @TempDir should be a type of “java.nio.file.Path ” or “java.io.File”.
Advantages of JUnit 5 – @TempDir
- The major advantages of the @TempDir annotation is its automatic cleanup mechanism, flexibility, readability and also the efficiency.
- Integration with the external libraries which requires the temporary directories during the testing of our test methods.
- With the usage of automatic cleanup mechanism, there is no need for explicit cleanup code at the end of the test methods.
Features of @TempDir
- @TempDir will automatically create the temporary directory before the test method runs.
- JUnit 5 will automatically clean the temporary directory after the test method completes irrespective of the output.
- we can create the single method with multiple temporary directories based on the requirements.
- It eliminates the need of manual setup and removing of the temporary directories.
Conclusion
The @TempDir is a valuable tool for the developers to engage with the unit testing with JUnit 5. The automatic cleanup mechanism provided by @TempDir ensures that each test method operates independently and reducing the interference between the tests. By using the @TempDir we can reduce the boilerplate cleanup code and provides a standard approach to the temporary directory management. @TempDir can contribute the overall code quality and maintainability.
Share your thoughts in the comments
Please Login to comment...