Julia – RCall
Last Updated :
10 Aug, 2023
To bridge the gap between the R language, which has been in the scene for a long time and is renowned for its huge collection of statistical and data analysis packages, and one of the newest members, Julia, which is a high-level programming language, the package RCall has been developed. using the RCall package, the developers can use the power and features of both languages simultaneously. use the libraries of R in Julia or vice versa. Even developers can run R code from the Julia prompt, transfer variables, etc. It allows seamless integration of R’s functionality within a Julia environment.
Prerequisites
1. The R language and Julia language both must be installed on the user’s device.
2. To install the RCall package, the user’s device must have R and Julia both installed. Just go to the command line and type julia, and the prompt will change to Julia. Then using the Pkg command we will install RCall.
using Pkg;
Pkg.add(“RCall”)
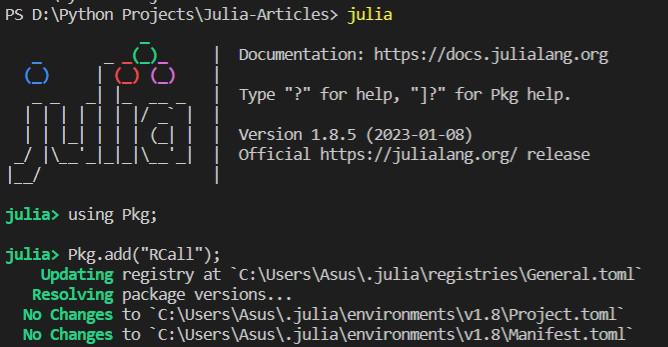
Sometimes there might be an error while installing RCall using Pkg. add(), if the user faces any error, then just run the below command in the same terminal.
Pkg.build(“RCall”)
Then just try to import the package and see if the error has been removed or not.

There is no error while importing the package.
Implementation
Now we will convert the Julia prompt into an R prompt. While in the same command line/ terminal press the SHIFT+$ key together, and the prompt will change from Julia to R. Now to go back to Julia we will just press the BACKSPACE once.
Julia
julia> using RCall;
R> r < - 100
R> r
R> print (r)
|
Output
100
Converting from Julia Code to R
We can switch between the Julia code and the R code. This means, that we can define a variable in Julia and map it to a R variable, and use that variable in R.
Julia
Julia> using RCall;
Julia> var = 1
R> x < - $var
R> x
[ 1 ] 1
|
Output
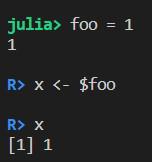
Using @rput and @rget Method
Using Julia Variable in R – @rput method
Using the rput and rget macros, we can transfer variables between R and Julia. The difference here with the previous method is that we can use the same variable we used in Julia in R, rather than mapping it to another variable in R prompt.
Julia
julia> using RCall;
julia> z = 10
10
julia> @rput z
10
R> z
[ 1 ] 10
|
Output
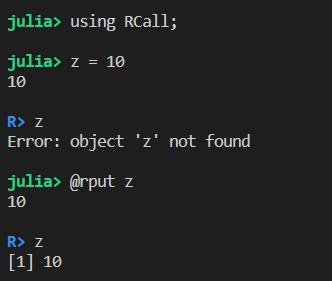
As we can see in the output, if we try to use the variable we used in Julia directly in R without using @rput macro, then we are getting an error. But if we use rput and then try to use the variable in R prompt, we are getting the output properly.
Using R Variable in Julia – @rget Method
Using the @rget macros we can access any variable declared in R in our Julia code, without mapping it to another variable.
Julia
julia> using RCall;
R> val = 20
julia> @rget val
20.0
julia> val
20.0
|
Output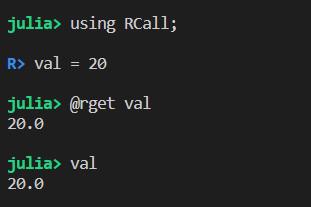
Using R ” ” (R_string) Method in Julia
There is another way to use R in Julia and that is using the R_string method i.e R”<code>”. The <code> section will be evaluated as an R code even though we are running it in Julia.
Syntax :
R”<code>”
We need to make sure that the <code> we are passing inside of R”” should follow the syntax of R language, not Julia.
Example 1: In the below example we will create a vector in R which stores 5 values and print it, all while in Julia Prompt. Also we need to use the RCall package i.e import it, otherwise we will face an error stating @R_str not defined.
Julia
julia> using RCall;
julia> R "c(10,20,30,40,50)"
RObject{RealSxp}
[ 1 ] 10 20 30 40 50
|
Output
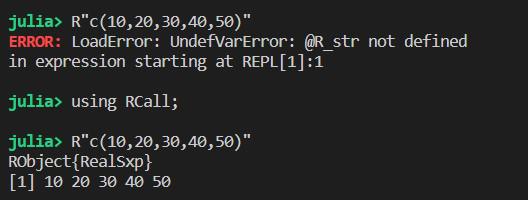
Explanation:
- As we can see in the above output, we tried to use the R_string without importing the RCall package, and we got an error. But after importing the RCall package, the code ran successfully.
- Here the c() is a built-in R function that concatenates elements passed as its parameters into a Vector object. We can use any R function inside the R””, it is not that only this c() will work and nothing else.
Example 2: In this example, we will see another function rather than c() inside of R_string. In this function, we will use the sum() function to find the sum of the first 10 natural numbers and print it.
Julia
julia> R "sum(1:10)"
RObject{IntSxp}
[ 1 ] 55
|
Output
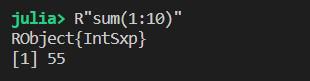
RCall API
1. rcopy() method
The rcopy() method is used to convert the RObjects into Julia objects. We will use the R_string method inside of rcopy() so that the output the R_string returns will be converted into Julia objects. we will use the rcopy() function in the Julia prompt not in the R prompt.
Example 1:
Julia
julia> rcopy(R "c(10,20)" )
2 - element Vector{Float64}:
10.0
20.0
|
Output
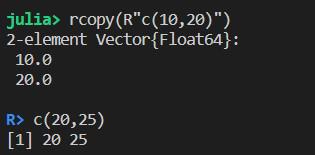
Example 2:
Julia
R "arr <- c(1,2,3,4)"
julia_arr = rcopy(R "arr" )
|
Output
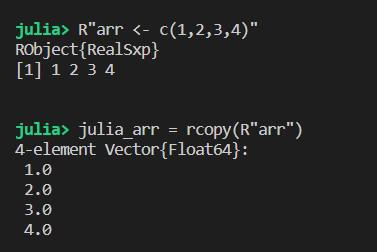
2. @reval
The reval function is used to evaluate R codes in the Julia environment. It is visible in the function name that it stands for R-eval means evaluating R codes in Julia’s environment. It takes a string parameter that consists of some R code. Evaluates the expression and return the result in Julia object.
Julia
using RCall
total = reval( "sum(c(1,2,3,4,5))" )
|
Output
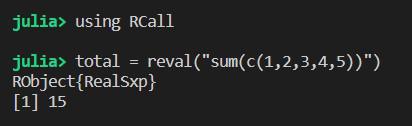
3. @rcall
The rcall function of RCall package is used to call any R functions in the current Julia environment. It requires the RCall package to be imported and used.the
Julia
using RCall
res = rcall(:mean, 1 : 5 )
|
Output
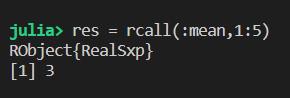
4. @robject
The robject function is used to convert a Julia variable or object into R object. It is useful to transfer results or anything computed in Julia’s environment into the R environment.
Julia
value = 10
typeof(value)
value = robject( 10 )
typeof(value)
|
Output
Int64
RObject{IntSxp}
[1] 10
RObject{IntSxp}
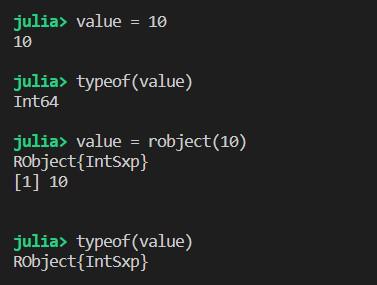
The @rlibrary and @rimport Macros
1. @rlibrary
The @rlibrary macro lets the user import the installed R packages into the current session in the Julia prompt. RCall package must be installed and imported to use it in our code.
Julia
julia> @rlibrary datasets
julia> R "head(iris)"
|
In the above code, we are importing the datasets package of R in our current Julia session using the rlibrary macro. Then we are using the iris dataset and the head() function of R to print the first 5 rows of the iris dataset.
Output
RObject{VecSxp}
Sepal.Length Sepal.Width Petal.Length Petal.Width Species
1 5.1 3.5 1.4 0.2 setosa
2 4.9 3.0 1.4 0.2 setosa
3 4.7 3.2 1.3 0.2 setosa
4 4.6 3.1 1.5 0.2 setosa
5 5.0 3.6 1.4 0.2 setosa
6 5.4 3.9 1.7 0.4 setosa
2. @rimport
The work of @rimport is similar to as of @rlibrary, but this allows to import of specific functions rather than the entire package. Also, it allows the users to import using an alias
Example –
Julia
julia> @rimport base as bs
julia> bs. sum ([ 10 , 20 , 30 ])
|
Output
60
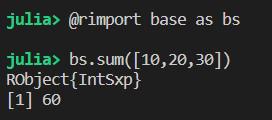
Share your thoughts in the comments
Please Login to comment...