JSP – File Downloading
Last Updated :
14 Mar, 2024
In this article, we will learn how to download files from the server/file directories using JSP. The JSP code has a response header indicating that text is being returned that should be downloaded rather than displayed.
- We will set the content type header to “APPLICATION/OCTET-STREAM”, and it will indicate that the content is binary data.
- The FileInputStream object reads the contents of a file which is specified by a file path and file name.
- By using FileInputStream, we can read the contents of the file and pass it directly to the output stream.
- This sends the contents of the file to the client’s browser in response.
Implementation to download File using JSP
Below is the Step-by-Step implementation to Download Files using JSP.
Step 1: Prepare sample files.
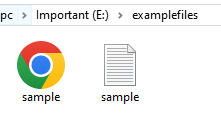
- File Path: E:/examplefiles
- Sample file 1: sample.pdf
- Sample file 2: sample.txt
Note: Replace the filename and filepath with your filename and filepath in the code.
Step 2: Create a new folder named “filedownload”. Create index.html, download-pdf.jsp, download-txt.jsp in the “filedownload” folder and move the folder to tomcat server. Copy and paste the below code in the corresponding files.
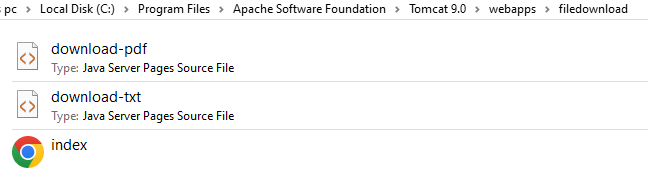
Step 3: Start the tomcat server and navigate to the index.html in the browser.
Note: Replace the port number with your port number.
Hit the below URL in browser:
http://localhost:8080/filedownload/index.html
index.html:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>File downloading in JSP</title>
</head>
<body>
<h1 style="color: green;">GeeksforGeeks</h1>
<button>
<a href="download-pdf.jsp">Download sample.pdf file</a>
</button>
<button>
<a href="download-txt.jsp">Download sample.txt file</a>
</button>
</body>
</html>
In the above HTML file, it creates a webpage with two buttons. Each button links to a JSP file (download-pdf.jsp
and download-txt.jsp
) and it is responsible for downloading a corresponding file (sample.pdf
and sample.txt
).
download-pdf.jsp:
HTML
<%
// Define filename and filepath
String filename = "sample.pdf";
String filepath = "E:\\examplefiles\\";
// Set response to download file
response.setContentType("APPLICATION/OCTET-STREAM");
response.setHeader("Content-Disposition", "attachment; filename=\"" + filename + "\"");
// Input stream for file reading
java.io.FileInputStream fileInputStream = new java.io.FileInputStream(filepath + filename);
// Read file content and stream it to the response output stream
int i;
while ((i=fileInputStream.read()) != -1) {
out.write(i);
}
// Close the FileInputStream to release resources
fileInputStream.close();
%>
The above code downloads a file named “sample.pdf” from “E:\examplefiles\” path. It sets the response headers for file download. Creates a FileInputStream to read the file content and streaming it to the response output stream. At last, it closes the stream.
download-txt.jsp:
HTML
<%
// Define filename and filepath
String filename = "sample.txt";
String filepath = "E:\\examplefiles\\";
// Set response to download file
response.setContentType("APPLICATION/OCTET-STREAM");
response.setHeader("Content-Disposition", "attachment; filename=\"" + filename + "\"");
// Input stream for file reading
java.io.FileInputStream fileInputStream = new java.io.FileInputStream(filepath + filename);
// Read file content and stream it to the response output stream
int i;
while ((i=fileInputStream.read()) != -1) {
out.write(i);
}
// Close the FileInputStream to release resources
fileInputStream.close();
%>
This JSP code downloads a file named “sample.txt” from “E:\examplefiles\” by setting response headers for file download. Then it creates a FileInputStream to read the file content, and streaming it to the response output stream, ensuring to close the stream at the end.
Output:
Below in the output screen we can see the Files are ready to download.
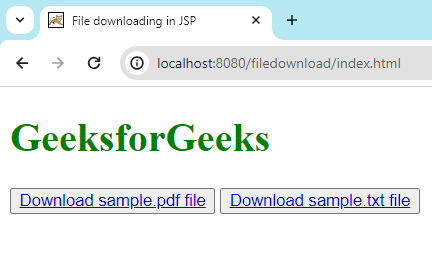
Then we can download the files as we can see in the below image:
Share your thoughts in the comments
Please Login to comment...