Serving GraphQL over HTTP
Last Updated :
19 Mar, 2024
HTTP or Hypertext Transfer Protocol, is the foundation of Web communication. Being a stateless protocol, each request from a client to the server is independent and doesn’t retain information about past requests. GraphQL is a query language for APIs and a runtime for executing those queries.
Unlike traditional REST APIs with fixed endpoints and predefined structures, GraphQL allows clients to request the specific data they need on the same endpoint.
One common way to serve GraphQL is over HTTP, providing an efficient and flexible means of communication between client and server. In this article, we’ll explore how we can use the HTTP protocol to serve GraphQL and how it differs from traditional RESTful approaches.
Web Request Pipeline
- The web request pipeline refers to the sequence of steps that occur when a client requests a web server.
- The server then processes the request and sends a response to the client.
- The pipeline includes the steps such as routing, authentication, authorization, request handling, and response generation.
- Modern web frameworks use the pipeline architecture which involves various middleware components and requests pass through them.
- Requests which can be inspected, transformed, modified or even terminated in the pipeline.
- GraphQL should be placed after authentication middleware or module. This is to provide access to the same session and user information in the HTTP endpoint.
URL and Routes
- URL stands for Uniform Resource Identifiers, which are strings of characters used to identify resources on the web.
- They consist of two main parts: the protocol (e.g., http:// or https://) and the resource’s location (For Example: ., www.geeksforgeeks.org).
- Routes, on the other hand, are paths defined within a web application that map incoming requests to specific handlers or controllers responsible for processing those requests.
- For example, in a web application for an online store, the route “/products” might be used to display a list of products, while the route “/cart” might be used to manage the shopping cart.
- Now, in case of GraphQL applications, graphql server operates on a single URL of the API endpoint to perform all database operations. This URL is “/graphql” for most cases, however, developers can change the route as per their need.
- In GraphQL, data is organized as an entity graph, which means each piece of data is connected to others in a network, unlike in REST where data is accessed via URLs.
HTTP Methods
HTTP Methods are also known as HTTP which signifies the action to be performed on a resource.
- GET: This method is used to request a representation of specified resource. GET method is used for data retrieval.
- POST: It is a method which is used to submit an entity to the specified resource. POST method submits the data to processed, causing a change in state on the server.
- To get better understanding difference between the GET and POST you can refer this.
- GraphQL server handles HTTP GET and POST methods for operations. Usually we use GET for Query and POST for Mutation.
HTTP GET Request
When we are using a HTTP GET request to execute a GraphQL query,
- The query is specified in the “query” string parameter.
- Query variables can be sent as JSON encoded string in an additional query parameter called “variables“.
- If our query contains multiple operations, then we can use OperationName as a query parameter to specify which operation to be executed.
GET method will be used when we are using Query in graphql to fetch data. Below is the GraphQL query format for “Query” or to retrieve data.
{
"[links] {
"[url]" , [description]"
}
}
The above request in GET will look the following,
http://[apiendpoint]/graphql?query={[links]{[url] , [description}}
HTTP POST Request
GraphQL POST request use the application/json content type. A standard GraphQL POST request have the structure where the request body should be a JSON encoded object with the following fields,
- query: The GraphQL query string.
- operationName: This specifies the named operation to execute in case the query contains multiple operations. (Optional).
- variables: This contains query variables as a JSON object.
GraphQL format for POST:
{
"query": "{ Links { url, description } }",
"variables": null,
"operationName": null
}
HTTP Response
Regardless of the request method, the response should be returned in the body of the response in JSON format. The payload contains,
- data: Contains the data of the GraphQL query execution.
- errors: Contains an array of error objects if any errors occurred during execution.
Response:
{
"data": { },
"errors": [ ]
}
Implementation Using GraphQL in a Java Spring Boot Application for Book Management
Let’s consider the following Java Spring Boot application, where we are using a relational database to store book details and we are using GraphQL dependency to interact with the database.
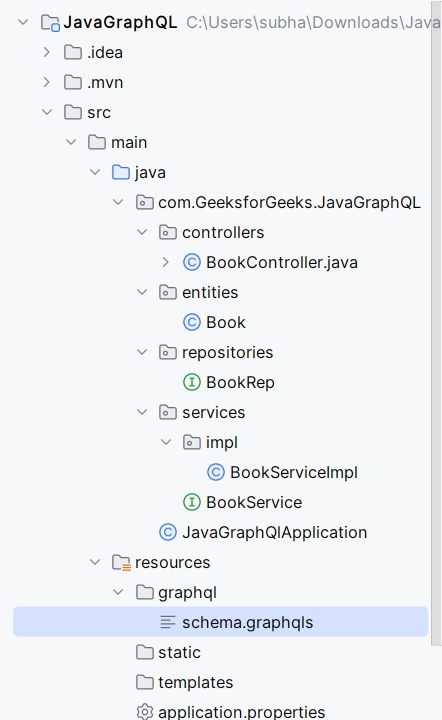
Folder Structure
Following is the database structure we are using here,
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id:
private String title;
private String desc;
private String author;
private double price:
private int pages;
}
Now all GraphQL configurations are done in the schema.graphqls file where we have defined the table structure and query and mutation methods to use when we will use the API endpoint.
Response:
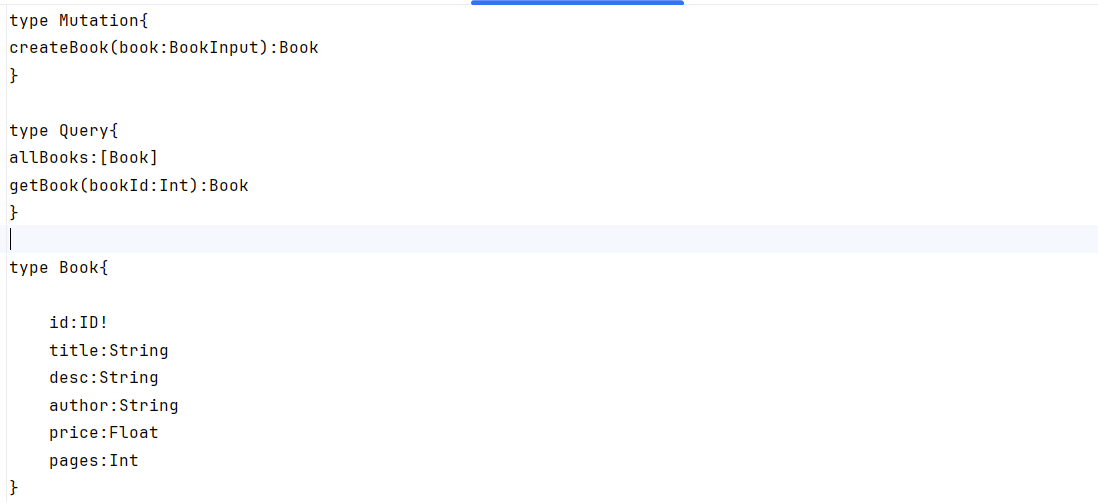
The application is running in port no 9000, so the API for GraphQL is localhost:9000/graphql.

HTTP GET:
Let’s use GET Method to get details of all books in the database. As we are using GraphQL, so we can choose the attribute we need to see. For example let’s check the book’s Id, name, author name, and price.

GET
localhost:9000/graphql?query={allBooks{id title author price}}
Response:
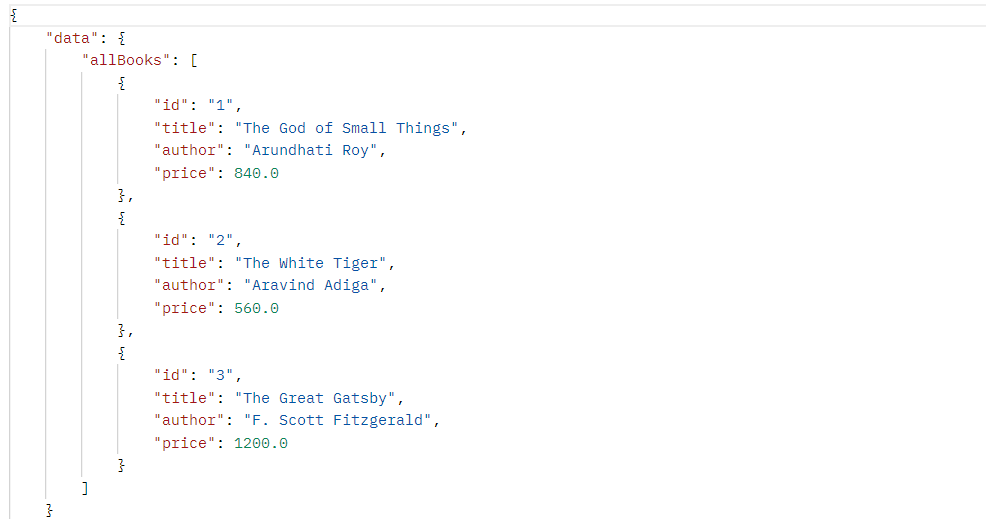
HTTP POST:
We can use POST method to manipulate data and retrieve data also. Let’s try a GraphQL mutation where we are going to send a book details to store in the database using POST Method.
Response:
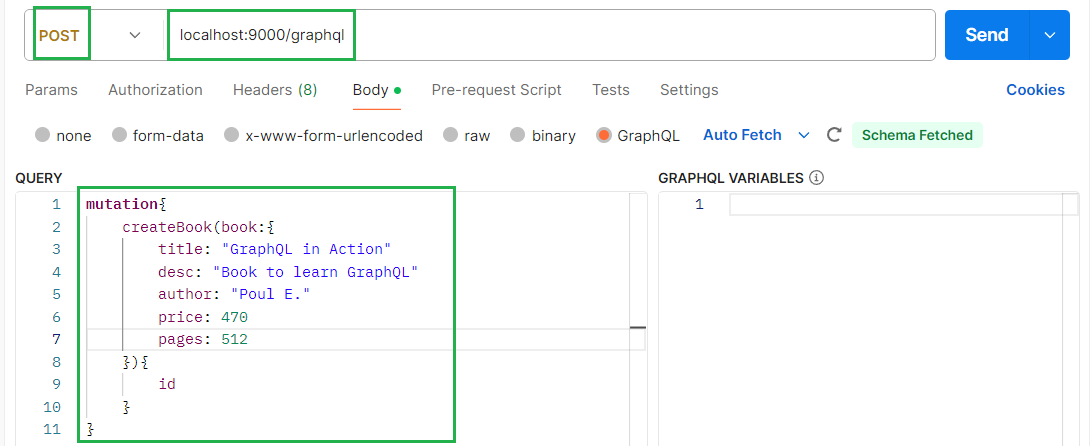
Mutation:
mutation{
createBook(book:{
title: "GraphQL in Action"
desc: "Book to learn GraphQL"
author: "Poul E."
price: 470
pages: 512
}){
id
}
}
Response:
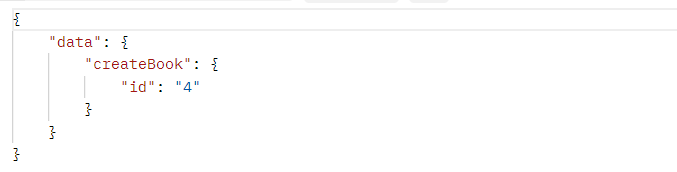
Conclusion
Using GraphQL provides developers the privilege to communicate with database using a single HTTP endpoint. HTTP methods like GET and POST is used to interact where GET is used to fetch data and POST is to send data. Responses follow a consistent JSON structure containing “data” and “error”. Following conventions and using best practices, developers ensure consistent and better communication between client and servers, integrating GraphQL into web applications.
Share your thoughts in the comments
Please Login to comment...