JavaScript Program to Add Two Matrices
Last Updated :
26 Oct, 2023
Given two N x M matrices. Find a N x M matrix as the sum of given matrices each value at the sum of values of corresponding elements of the given two matrices
In this article, we will see how we can perform the addition of two input matrices using JavaScript.
Example:
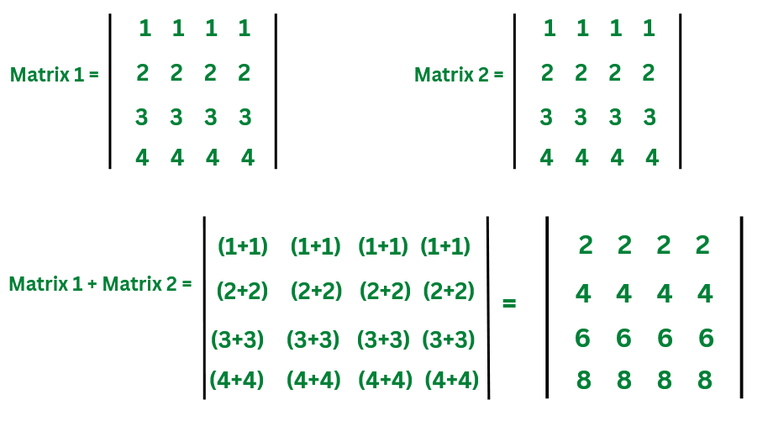
Approach 1: Using Loop in JavaScript
In this approach, we are using for loops to iterate over every individual element of the input matrices. This approach can perform the addition of any of the number of matrices, and then we are performing the addition of the response element and saving the result of the addition in the result matrix.
Syntax:
for (statement 1; statement 2; statement 3) {
code here...
}
Example: In this example, we will be performing the Addition of two Matrices in JavaScript by using Loops.
Javascript
let mat1 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let mat2 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let resmat = [];
for (let i = 0; i < mat1.length; i++) {
let r = "" ;
for (let j = 0; j < mat1[i].length; j++) {
r += mat1[i][j] + mat2[i][j] + " " ;
}
resmat.push(r.trim());
}
resmat.forEach(r => console.log(r));
|
Output
2 2 2 2
4 4 4 4
6 6 6 6
8 8 8 8
Approach 2: Using map() method
In this approach, we are using the inbuilt ‘map‘ method to create a new output matrix by adding the input matrices. Here, the code can perform the addition of two matrices in JavaScript.
Syntax:
map((element, index, array) => { /* … */ })
Example: In this example, we will be performing the Addition of two Matrices in JavaScript by using map method.
Javascript
let mat1 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let mat2 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let resmat = mat1.map((r, i) =>
r.map((v, j) => v + mat2[i][j])
);
for (let i = 0; i < resmat.length; i++) {
console.log(resmat[i].join( ' ' ));
}
|
Output
2 2 2 2
4 4 4 4
6 6 6 6
8 8 8 8
Approach 3: Using the Array.from() method
In this approach, we are using the Array.from() method in JavaScript. By using this function, we create a new matrix by iterating over each row and column of all the input matrices. Before this, we are flattening the input matrices to convert the 2D array into the 1D array so that the element additon will be easily performed.
Syntax:
Array.from(object, mapFunction, thisValue)
Example: In this example, we will be performing the Addition of two Matrices in JavaScript by using Array.from() method.
Javascript
let mat1 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let mat2 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let m = Array.from(mat1.flat());
let n = Array.from(mat2.flat());
let resarr = m.map((v, i) => v + n[i]);
let resmat = [];
while (resarr.length) {
resmat.push(resarr.splice(0, mat1[0].length));
}
resmat.forEach(row =>
console.log(row.join( ' ' )));
|
Output
2 2 2 2
4 4 4 4
6 6 6 6
8 8 8 8
Share your thoughts in the comments
Please Login to comment...