JavaScriptProgram to Check Two Matrices are Identical or Not
Last Updated :
18 Oct, 2023
We have given two matrices with the data as the input and the task is to check whether these input matrices are identical/same or not. When the number of rows and columns and the data elements are the same, the two matrices are identical. Below is the Example for better understanding:
Example:
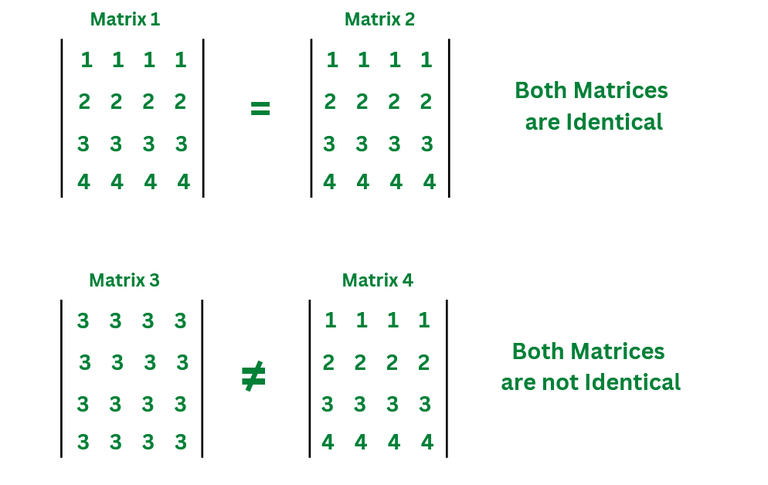
So to check whether the input matrices are identical or not in JavaScript, we have three different approaches that are listed below:
So lets see each of the approach with its implementation:
Approach 1: Using For Looping
In this approach, we use the for loop in JavaScript to go through the element of both the input matrices of (mat1 and mat2). Then we are comparing the values of the adjacent elements. While comparing this, if the elements are not same then the value of same variable is updated as false and the loop is break. If the same value is true then this results into the output of Identical Matrices.
Example: In this example, we will see the use of for loop in JavaScript.
Javascript
let mat1 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4]
];
let mat2 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4]
];
let same = true ;
for (let i = 0; i < mat1.length; i++) {
for (let j = 0; j < mat1[i].length; j++) {
if (mat1[i][j] !== mat2[i][j]) {
same = false ;
break ;
}
}
}
if (same) {
console.log( "Both Matrices are Identical" );
} else {
console.log( "Both Matrices are not Identical" );
}
|
Output
Both Matrices are Identical
In this approach, we are using the inbuilt JSON.stringify() method for checking whether the input matrices are same or not. Here the stringify() method mainly converts the matrices into the JSON strings. Then it compares the JSON strings, if the JSON strings are same then it means matrices are having same values and are in same order. We are printing the output using console.log() function.
Example: In this example, we will see the use of JSON.stringify() in JavaScript.
Javascript
let mat1 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4]
];
let mat2 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4]
];
let same = (m1, m2) =>
JSON.stringify(m1) === JSON.stringify(m2);
if (same(mat1, mat2)) {
console.log( "Both Matrices are Identical" );
} else {
console.log( "Both Matrices are not Identical" );
}
|
Output
Both Matrices are Identical
Approach 3: Using every() and flat() Methods
In this approach, we are using the inbuilt method as every() and flat() in JavaScript. Here, the flat() method converts the matrices into flat arrays which consists of the elements of the matrices. Then by using the every() method, we are going through the elements of this array and checking if the elements of m1 is same as m2. If they are same then it mesna that our input matrices are same, else they are not same.
Example: In this example, we will see the use of every and flat methods in JavaScript.
Javascript
let mat1 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4]
];
let mat2 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4]
];
let same = (m1, m2) =>
m1.flat().every((val, ind) => val === m2.flat()[ind]);
if (same(mat1, mat2)) {
console.log( "Both Matrices are Identical" );
} else {
console.log( "Both Matrices are not Identical" );
}
|
Output
Both Matrices are Identical
Share your thoughts in the comments
Please Login to comment...