JavaScript Program for Subtraction of Matrices
Last Updated :
12 Oct, 2023
Subtraction of two matrices is a basic mathematical operation that is used to find the difference between the two matrices. In this article, we will see how we can perform the subtraction of input matrices using JavaScript.
Example:
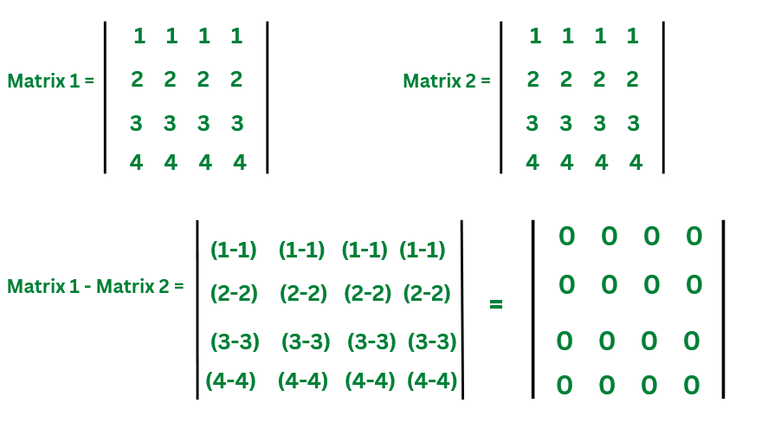
Using Loop in JavaScript
In this approach, we are using for loops to iterate over every individual element of the input matrices. This approach can perform the subtraction of any of the number of matrices, and then we are performing the subtraction of the response element and saving the result of the subtraction in the result matrix.
Syntax:
for (statement 1 ; statement 2 ; statement 3){
code here...
}
Example: In this example, we will be performing the Subtraction of Matrices in JavaScript by using Loops.
Javascript
function MatSubUSingForLoop(inputMat) {
if (inputMat.length < 2) {
return null ;
}
let outputMat = inputMat[0];
for (
let i = 1;
i < inputMat.length;
i++) {
let currMat = inputMat[i];
if (
outputMat.length !==
currMat.length ||
outputMat[0].length !==
currMat[0].length) {
console.log( "Not Possible" );
return -1;
}
for (
let j = 0;
j < outputMat.length;
j++) {
for (
let k = 0;
k < outputMat[j].length;
k++) {
outputMat[j][k] -=
currMat[j][k];
}}}
return outputMat;
}
let inputMatrix1 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
[5, 5, 5, 5],
];
let inputMatrix2 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let inputMatrix3 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let resultMatrix = MatSubUSingForLoop([
inputMatrix1,
inputMatrix2,
inputMatrix3,
]);
for (
let i = 0;
i < resultMatrix.length;
i++) {
console.log(
resultMatrix[i].join( " " ));
}
|
Using the map method in JavaScript
In this approach, we are using the inbuilt ‘map‘ method to create a new output matrix by substracting the input matrices. Here, the code can perform the substraction of any number of the matrices.
Syntax:
map((element, index, array) => { /* … */ })
Example: In this example, we will be performing the subtraction of matrices in JavaScript by using the map method.
Javascript
function matSubUsingMap(inputMat) {
if (inputMat.length < 2) {
return null ;
}
let resultMatrix = inputMat[0];
for (
let i = 1;
i < inputMat.length;
i++) {
let currMat = inputMat[i];
if (
resultMatrix.length !==
currMat.length ||
resultMatrix[0].length !==
currMat[0].length) {
console.log( "Not Possible" )
return null ;
}
resultMatrix = resultMatrix.map(
function (rowMat, i) {
return rowMat.map(
function (
valueMat, j) {
return (
valueMat -
currMat[i][j]);
});
});
}
return resultMatrix;
}
let inputMatrix1 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let inputMatrix2 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let inputMatrix3 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let resultMatrix = matSubUsingMap([
inputMatrix1,
inputMatrix2,
inputMatrix3,
]);
for (
let i = 0;
i < resultMatrix.length;
i++) {
console.log(
resultMatrix[i].join( " " ));
}
|
Output
-1 -1 -1 -1
-2 -2 -2 -2
-3 -3 -3 -3
-4 -4 -4 -4
In this approach, we are using the Array.from() method in JavaScript. By using this function, we create a new matrix by iterating over each row and column of all the input matrices.
Syntax:
Array.from(object, mapFunction, thisValue)
Example: In this example, we will be performing the subtraction of matrices in JavaScript by using Array.from().
Javascript
function subtractMatricesUsingFrom(
inputMat) {
if (inputMat.length < 2) {
return null ;
}
let resultMatrix = inputMat[0];
for (
let i = 1;
i < inputMat.length;
i++) {
let currMat = inputMat[i];
if (
resultMatrix.length !==
currMat.length ||
resultMatrix[0].length !==
currMat[0].length) {
console.log( "Not Possible" );
return null ;
}
resultMatrix = Array.from(
{
length: resultMatrix.length,
},
(_, i) =>
Array.from(
{ length: resultMatrix[i].length, },
(_, j) =>
resultMatrix[i][j] - currMat[i][j]
));
}
return resultMatrix;
}
let inputMatrix1 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let inputMatrix2 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let inputMatrix3 = [
[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3],
[4, 4, 4, 4],
];
let resultMatrix =
subtractMatricesUsingFrom([
inputMatrix1,
inputMatrix2,
inputMatrix3,
]);
resultMatrix.forEach((row) => {
console.log(row.join( " " ));
});
|
Output
-1 -1 -1 -1
-2 -2 -2 -2
-3 -3 -3 -3
-4 -4 -4 -4
Share your thoughts in the comments
Please Login to comment...