JavaScript BaseX Converter: Converting Numbers Between Different Bases
Last Updated :
22 Sep, 2023
In the world of computer science and mathematics, numbers are represented in various base systems such as decimal, binary, hexadecimal, and octal. Converting numbers between these different base systems is a common requirement. For example, you may need to convert a decimal number to binary, a binary number to octal, or even convert between less common bases like base-15. Performing these conversions manually can be time-consuming and prone to errors. To address this need, we present the BaseX Converter, a handy tool that allows you to convert numbers from one base to another effortlessly.
Example:
1. Input: Converting (13)₁₆ to base 10
Let’s take the example of converting the number (13)₁₆ to base 10 (decimal). We can break down the conversion process as follows:
First, we multiply each digit of the number by the corresponding power of 16:
(13)₁₆ = 1 * 16^1 + 3 * 16^0
Next, we calculate the results of the above multiplication:
(13)₁₆ = 16 + 3
Finally, we add up the results to obtain the decimal equivalent:
(13)₁₆ = 19₁₀
Therefore, (13)₁₆ is equal to (19)₁₀ in base-10.
2. Input: Converting (10101)₂ to base-8:
Now, let’s convert the binary number (10101)₂ to base 8 (octal). The conversion process involves grouping the binary digits into sets of three, starting from the rightmost digit, and converting each group to its octal equivalent:
(10101)₂ = 010 101
The grouped binary digits are then converted to octal:
010₂ = 2₈
101₂ = 5₈
Combining the octal digits, we get:
(10101)₂ = (25)₈
Therefore, (10101)₂ is equal to (25)₈ in base-8.
Prerequisites:
Approach:
- Set up the HTML structure with input fields for the number, from base, and to base, along with a button and a result display area.
- Implement the convert() function:
- Retrieve the input values from the respective fields.
- Convert the input number from the specified “fromBase” to decimal using parseInt().
- Convert the decimal number to the specified “toBase” using toString().
- Update the result display area with the converted result.
- Style the webpage using CSS to create a visually appealing layout.
- When the user clicks the “Convert” button, the convert() function is executed, performing the conversion and updating the result display area with the converted value.
Example: In this example, we will illustrate the base converter using HTML, CSS and JS.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
BaseX Calculator
</ title >
< style >
body {
font-family: Arial, sans-serif;
}
h1 {
text-align: center;
}
#converter {
margin: 20px auto;
width: 300px;
text-align: center;
}
label {
display: block;
margin: 5px 5px;
}
input[type="number"] {
width: 100%;
padding: 5px;
box-sizing: border-box;
}
select {
width: 100%;
padding: 5px;
box-sizing: border-box;
margin-bottom: 10px;
}
button {
padding: 10px 20px;
background-color: #4caf50;
color: white;
border: none;
cursor: pointer;
margin: 5px 5px;
}
#result {
margin-top: 20px;
text-align: center;
}
</ style >
</ head >
< body >
< h1 >BaseX Calculator</ h1 >
< div id = "converter" >
< label >Number:</ label >
< input type = "number" id = "numberInput" />
< label >From Base:</ label >
< input type = "number" id = "fromBaseInput" />
< label >To Base:</ label >
< input type = "number" id = "toBaseInput" />
< button onclick = "convert()" >
Convert
</ button >
</ div >
< div id = "result" ></ div >
< script >
function convert() {
let numberInput =
document.getElementById("numberInput").value;
let fromBase =
parseInt(document.getElementById("fromBaseInput").value);
let toBase =
parseInt(document.getElementById("toBaseInput").value);
let result = "";
// Convert the number from the "fromBase" to decimal
let decimalNumber =
parseInt(numberInput, fromBase);
// Convert the decimal number to the "toBase"
result =
decimalNumber.toString(toBase);
document.getElementById("result").innerHTML =
"Result: " + result;
}
</ script >
</ body >
</ html >
|
Output:
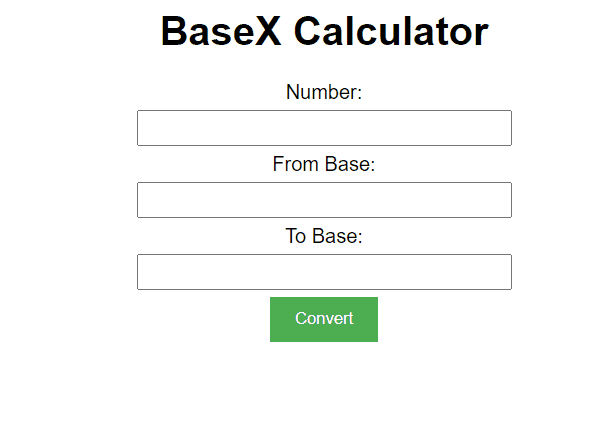
Share your thoughts in the comments
Please Login to comment...