What is TypeError: Converting Circular Structure to JSON ?
Last Updated :
22 Mar, 2024
The “TypeError: Converting circular structure to JSON” error occurs when attempting to stringify objects with circular references using JSON.stringify(). We’ll see the reasons for this error and various solutions to handle circular structures during JSON serialization.
What is TypeError: Converting circular structure to JSON?
The “TypeError: Converting circular structure to JSON” error occurs when attempting to stringify an object with circular references using JSON.stringify(). JSON format does not support circular structures, so this error indicates that the serialization process cannot handle the circular references within the object being converted to JSON.
Error:
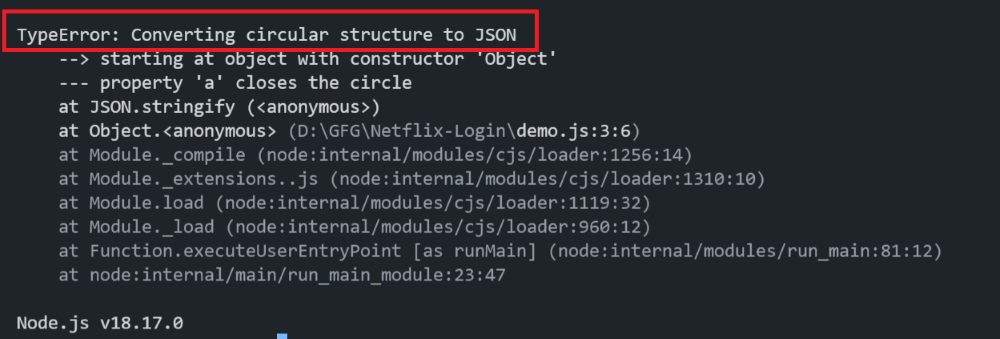
Why does “TypeError: Converting circular structure to JSON” occur?
Below, are the reasons for “TypeError: Converting circular structure to JSON” occurring:
- Circular Reference in Object or Array
- Circular Reference in Class
Reason 1: Circular Reference in Object or Array
In this scenario, a circular reference is created within the object obj, where the obj.circular property points back to the obj itself. When attempting to convert obj to JSON using JSON.stringify, the error “TypeError: Converting circular structure to JSON” occurs because JSON does not support circular references by default.
Example: The below code will generate the “TypeError: Converting circular structure to JSON” error.
JavaScript
const obj = {};
obj.circular = obj;
const jsonString = JSON.stringify(obj);
Output:
Hangup (SIGHUP)
/home/guest/sandbox/Solution.js:3
const jsonString = JSON.stringify(obj); // Error occurs here
^
TypeError: Converting circular structure to JSON
--> starting at object with constructor 'Object'
--- property 'circular' closes the circle
at JSON.stringify (<anonymous>)
at Object.<anonymous> (/home/guest/sandbox/Solution.js:3:25)
at Module._compile (node:internal/modules/cjs/loader:1198:14)
at Object.Module._extensions..js (node:inte...
Reason 2: Circular Reference in Class
In this scenario, a circular reference is created within the errorClass constructor, where the this.self property points back to the errorClass instance itself. When attempting to stringify obj using JSON.stringify, the error “TypeError: Converting circular structure to JSON” occurs because JSON serialization does not handle circular references automatically in class instances.
Example: The below code will generate the “TypeError: Converting circular structure to JSON” error.
JavaScript
class errorClass {
constructor() {
this.self = this;
}
}
const obj = new errorClass();
JSON.stringify(obj);
Output:
Hangup (SIGHUP)
/home/guest/sandbox/Solution.js:7
JSON.stringify(obj);
^
TypeError: Converting circular structure to JSON
--> starting at object with constructor 'errorClass'
--- property 'self' closes the circle
at JSON.stringify (<anonymous>)
at Object.<anonymous> (/home/guest/sandbox/Solution.js:7:6)
at Module._compile (node:internal/modules/cjs/loader:1198:14)
at Object.Module._extensions..js (node:internal/modules/cjs/loader:1252:10)
at Module.load (node:i...
Methods to solve “TypeError: Converting circular structure to JSON“
Below are the solution for “TypeError: Converting circular structure to JSON”:
- Use a replacer function to handle circular references
- Implementing Custom toJSON method in Class
Solution 1: Use a replacer function to handle circular references
In this solution, a replacer function is used in JSON.stringify to handle circular references. The function checks if a value is an object and not null, then replaces circular references with a placeholder value (‘circular reference’). This prevents the “TypeError: Converting circular structure to JSON” error by handling circular structures during the JSON stringify operation.
Example: The below code will solve the “TypeError: Converting circular structure to JSON” error.
JavaScript
const obj = {};
obj.circular = obj;
const res = JSON.stringify(obj, (key, value) => {
if (typeof value === 'object' && value !== null) {
if (value instanceof Array) {
return value.map(
(item, index) =>
(index === value.length - 1 ?
'circular reference' : item));
}
return { ...value, circular: 'circular reference' };
}
return value;
});
console.log(res);
Output{"circular":"circular reference"}
Solution 2: Implementing Custom toJSON method in Class
In this solution, we are implementing a custom toJSON method in the errorClass to handle the circular reference scenario. This custom method returns a JSON object with a placeholder value (‘[Circular Reference]‘) for the circularly referenced property, allowing JSON.stringify to serialize the object without giving the error.
Example: The below code will solve the “TypeError: Converting circular structure to JSON” error.
JavaScript
class errorClass {
constructor() {
this.self = this;
}
toJSON() {
return { self: '[Circular Reference]' };
}
}
const obj = new errorClass();
const res = JSON.stringify(obj);
console.log(res);
Output{"self":"[Circular Reference]"}
Share your thoughts in the comments
Please Login to comment...