Design a Number System Converter in JavaScript
Last Updated :
09 Aug, 2023
A Number System Converter is one that can be used to convert a number from one type to another type namely Decimals, Binary, Octal, and Hexadecimal.
In this article, we will demonstrate the making of a number system calculator using JavaScript. It will have the option to select the number type for input as well as the resulting output. The default input will be 1 that on change will show simultaneous results.
On completion, it will look like this:
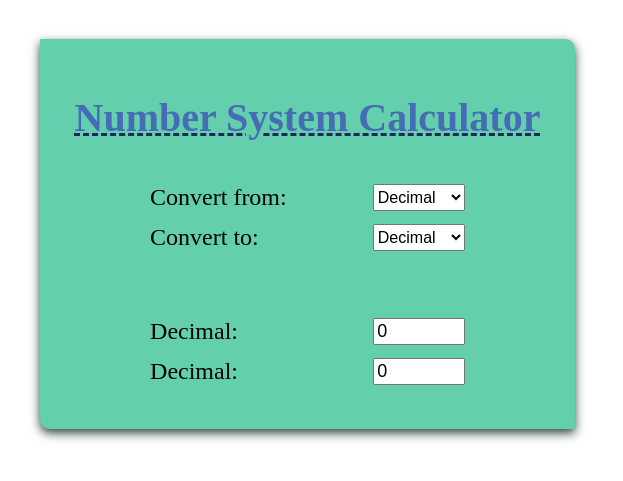
Prerequisites
Project Structure
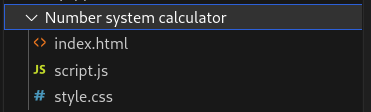
Approach
- Create a basic structure of a number system converter using HTML elements like form, section, and input for taking number input, and select an option element for dropdown input for number type along with relevant classes and ids.
- Style the calculator using CSS properties like margin, padding, background color, color, display, width, font sizes, etc.
- In JavaScript create an update function to update the values when input is added and a reverse function to update the values when other result input is changed to maintain the correct value
- Access the HTML element using HTML DOM methods like document.getElementById and innerText and value methods to access and update the values on the web page.
Example: This code example illustrates the number system calculator using Javascript.
Javascript
let typeFrom = document.getElementById( "typeFrom" );
let typeTo = document.getElementById( "typeTo" );
let res = document.getElementById( "res" );
let input = document.getElementById( "input" );
let inputType = document.getElementById( "inputType" );
let resultType = document.getElementById( "resultType" );
const tags = {
10: "Decimal:" ,
2: "Binary:" ,
8: "Octal:" ,
16: "HexaDecimal:" ,
};
function update() {
inputType.innerText = tags[typeFrom.value];
resultType.innerText = tags[typeTo.value];
res.value = Number(
parseInt(input.value, typeFrom.value)
).toString(typeTo.value);
}
function reverse() {
inputType.innerText = tags[typeFrom.value];
resultType.innerText = tags[typeTo.value];
input.value = Number(
parseInt(res.value, typeTo.value)
).toString(typeFrom.value);
}
update();
|
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" />
< title >Number System Calculator</ title >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 " />
< link rel = "stylesheet" href = "style.css" />
</ head >
< body >
< div class = "root" >
< h1 class = "title" >Number System Calculator</ h1 >
< section >
< div class = "option" >
< span >
< label >Convert from:</ label >
< select id = "typeFrom"
onchange = "reverse()" >
< option value = "10" >
Decimal
</ option >
< option value = "2" >
Binary
</ option >
< option value = "8" >Octal</ option >
< option value = "16" >
HexaDecimal
</ option >
</ select > </ span >< span >
< label >Convert to:</ label >
< select id = "typeTo"
onchange = "update()" >
< option value = "10"
lable = "Decimal" >
Decimal
</ option >
< option value = "2" >
Binary
</ option >
< option value = "8" >Octal</ option >
< option value = "16" >
HexaDecimal
</ option >
</ select >
</ span >
< br />
< br />
< span >
< label id = "inputType" >Enter {Decimal}:</ label >
< input type = "text"
min = "0"
value = "0"
id = "input"
onkeyup = "update()" />
</ span >
< span >
< label id = "resultType" >Result {Octal}:</ label >
< input id = "res"
onkeyup = "reverse()"
type = "text"
min = "0"
value = "0" />
</ span >
</ div >
</ section >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
body {
text-align : center ;
margin : auto ;
}
.title {
font-size : 2.5 rem;
color : rgba( 35 , 95 , 180 , 0.72 );
text-decoration : underline dashed 3px rgb ( 23 , 50 , 92 );
}
.root {
margin-top : 5% ;
display : flex;
flex- direction : column;
max-width : 30 rem;
margin : auto ;
margin-top : 5% ;
box-shadow: 0 4px 10px rgb ( 46 , 63 , 57 );
background-color : rgb ( 122 , 199 , 173 );
border-radius: 0 10px ;
padding : 3% ;
}
section {
font-size : x-large ;
padding : 2% ;
margin : auto ;
text-align : left ;
}
span>label {
width : 80% ;
}
.option {
display : flex;
flex- direction : column;
}
.option>span {
display : flex;
padding : 2% ;
}
select {
width : 33% ;
font-size : medium ;
outline : none ;
}
input {
width : 30% ;
font-size : large ;
}
|
Output:
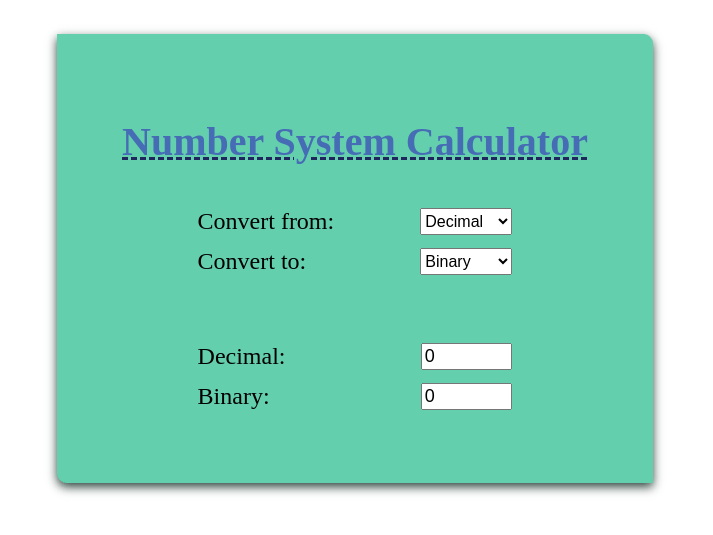
Share your thoughts in the comments
Please Login to comment...