Programming is a mind sport in which the participating programmers try to find the best solution to the problem as soon as possible. It helps in developing problem-solving skills which are crucial in software development. In general, C++ is the popular choice for programming but Java is also widely used in the software development industry.Â
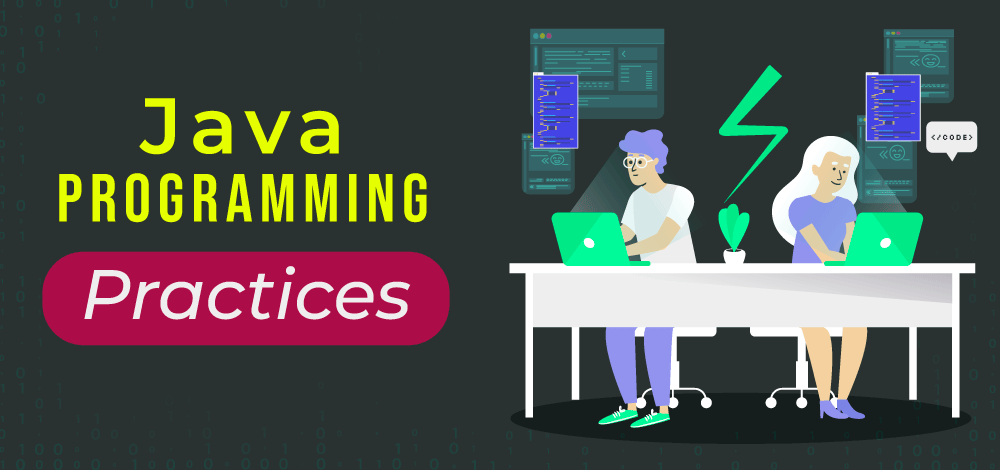
This article is for those programmers who want to improve themselves using programming in Java. In this article, we will discuss the 10 best programming practices that can be followed in the case of both Java Programming as well as Java Development.
But before we start let’s understand What is Java Programming?
What is Java Programming?
Java is one of the most popular programming languages created in 1995, used to create various applications such as Mobile applications, Desktop applications, and other Web applications. Java is easy to learn simple to use and is open-source and free to use. It is easy to use, secure and has huge community support. Java is also an object-oriented language that gives a clear structure to programs and allows code to be reused, lowering development costs.Â
Java Programming Best Practices
To make the most of Java’s capabilities and produce high-quality code, it’s essential to follow best programming practices. Now, let’s explore some of the 10 best Java programming practices that can help you write clean, efficient, and maintainable code.
1. Follow Coding Standards and Best Practices
Following coding standards is essential for the consistency and readability of code in both software code as well as normal programming code as it helps to debug and remove errors in the code. Oracle provides a set of code conventions for writing Java code, which covers topics such as naming conventions, indentation, and commenting. Adhering to these conventions can improve code readability, maintainability, and reduce the likelihood of errors in the code and help in debugging the code.
Example:
Java
public class GFG {
private int variableOne;
private String variableTwo;
public GFG( int variableOne, String variableTwo)
{
this .variableOne = variableOne;
this .variableTwo = variableTwo;
}
public void setVariableOne( int variableOne)
{
this .variableOne = variableOne;
}
public int getVariableOne() { return variableOne; }
public void setVariableTwo(String variableTwo)
{
this .variableTwo = variableTwo;
}
public String getVariableTwo() { return variableTwo; }
}
|
2. Using Efficient Data Structures
In Java programming, choosing the right data structure is critical for the optimal performance of the code. For example, when working with large datasets, it is better to use an array or ArrayList instead of a LinkedList. Similarly, using a PriorityQueue can help improve the efficiency of certain algorithms. Such data structures can help you optimize your code and bring down the time and space complexity of the code.
Example:
Java
import java.util.Arrays;
public class GFG {
public static void main(String[] args)
{
int [] arr = { 5 , 8 , 1 , 3 , 7 };
Arrays.sort(arr);
for ( int i = 0 ; i < arr.length; i++) {
System.out.print(arr[i] + " " );
}
}
}
|
3. Using Efficient Algorithms During Coding Contests
In programming, the efficiency of the used algorithms can make a significant difference in the performance of the code. It is essential to use efficient algorithms for solving problems during coding or participating in contests. When searching for an element in an array, it is better to use binary search instead of linear search. An efficient algorithm needs to be used in order to reduce the time complexity of the code and further optimize the code.
Example:
Java
public class GFG {
public static void main(String[] args)
{
int [] arr = { 1 , 3 , 5 , 7 , 9 };
int target = 5 ;
int low = 0 ;
int high = arr.length - 1 ;
int mid;
while (low <= high) {
mid = (low + high) / 2 ;
if (arr[mid] == target) {
System.out.println( "Target found at index "
+ mid);
return ;
}
else if (arr[mid] > target) {
high = mid - 1 ;
}
else {
low = mid + 1 ;
}
}
System.out.println( "Target not found" );
}
}
|
Output
Target found at index 2
4. Minimizing Input and Output Operations
In competitive as well as normal programming, (I/O) operations can be time-consuming which may cause your rank to go down during the contest. Therefore, it is essential to minimize the number of I/O operations as much as possible. You can read all the input data at once and store it in memory, and then perform all the computations before giving the output of the results.
the Example:
Java
import java.util.*;
import java.util.Scanner;
public class GFG {
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int [] arr = new int [n];
for ( int i = 0 ; i < n; i++) {
arr[i] = sc.nextInt();
}
int sum = 0 ;
for ( int i = 0 ; i < n; i++) {
sum += arr[i];
}
System.out.println(sum);
}
}
|
Output
5
1 2 3 4 5
15
5. Avoiding Excessive Object Creation in Java
Creating objects in Java can be expensive in terms of time and memory while participating in contests. Therefore, it is best to avoid excessive object creation whenever possible during contests. One way to do this is to use primitive types instead of wrapper classes.
Example:
Java
public class GFG {
public static void main(String[] args)
{
int x = 5 ;
Integer y = Integer.valueOf(x);
System.out.println(y);
}
}
|
6. Use of Java Standard Library Collections
The Java Standard Library Collections which is comparable with C++ STL provides a wide range of classes and methods functions that can be used for solving various problems efficiently and optimally. Therefore, it is essential to be familiar with the Collections and use its classes and methods wherever possible.
Example:
Java
import java.util.ArrayList;
class GFG {
public static void main(String[] args)
{
ArrayList<Integer> arrList
= new ArrayList<Integer>();
arrList.add( 10 );
arrList.add( 20 );
arrList.add( 30 );
System.out.println(arrList);
}
}
|
7. StringBuilder for String Manipulation
Strings are immutable in Java, which means that each time a string is modified, a new string object is created in memory. This can be inefficient, especially when dealing with large strings. Therefore, it is best to use the StringBuilder class for string manipulation, as it allows for efficient string concatenation and modification and helps you in the contests.
Example:
Java
public class GFG {
public static void main(String[] args)
{
StringBuilder sb = new StringBuilder( "Hello" );
sb.append( " World" );
System.out.println(sb.toString());
}
}
|
8. Use of Bitwise Operators
Bitwise operators can be used to solve problems more efficiently. Bitwise AND, OR, XOR, and shift operations can be used for various bitwise manipulations.
Example:
Java
class GFG {
public static void main(String[] args)
{
int a = 5 ;
int b = 3 ;
int c = a & b;
System.out.println(c);
}
}
|
9. Multithreading
In certain scenarios, multithreading can be used to improve the performance of the code during programming. If the problem during the contest involves processing large data, you can divide the data into smaller chunks and process them in parallel using multiple threads.
Example:
Java
class GFG extends Thread {
int start, end;
int [] arr;
int result;
public GFG( int start, int end, int [] arr)
{
this .start = start;
this .end = end;
this .arr = arr;
this .result = 0 ;
}
public void run()
{
for ( int i = start; i <= end; i++) {
result += arr[i];
}
}
public static void main(String[] args)
{
int n = 10000000 ;
int [] arr = new int [n];
for ( int i = 0 ; i < n; i++) {
arr[i] = i;
}
int numThreads = 4 ;
int chunkSize = n / numThreads;
GFG[] threads = new GFG[numThreads];
for ( int i = 0 ; i < numThreads; i++) {
int start = i * chunkSize;
int end = (i + 1 ) * chunkSize - 1 ;
threads[i] = new GFG(start, end, arr);
threads[i].start();
}
int result = 0 ;
for ( int i = 0 ; i < numThreads; i++) {
try {
threads[i].join();
result += threads[i].result;
}
catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(result);
}
}
|
10. Optimizing the Code
It is important to optimize your code for performance during the contest. This can involve various techniques such as loop unrolling, reducing branching, and minimizing variable assignments. It is also essential to test your code thoroughly to identify and fix any performance issues and remove errors so it is necessary that your code can be debugged properly for errors and issues.
Here are two examples of code, one before optimization and the other after optimization:
Non-Optimized Code:
Java
public static int sumOfArray( int [] arr)
{
int sum = 0 ;
for ( int i = 0 ; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
|
Optimized Code:
Java
public static int sumOfArray( int [] arr)
{
int sum = 0 ;
int i = 0 ;
int len = arr.length;
for (; i < len - (len % 4 ); i += 4 ) {
sum += arr[i] + arr[i + 1 ] + arr[i + 2 ]
+ arr[i + 3 ];
}
for (; i < len; i++) {
sum += arr[i];
}
return sum;
}
|
In the optimized code, we are using a generator expression inside the sum() function which creates a generator object that generates the squares of the numbers from 0 to n-1. The “sum()” function then adds up all the squares and returns the total. This is a more concise and efficient way of achieving the same result as the original code.
Advantages of Implementing these practices
- Code Readability: This best practices will help in enhancing your code readability and help you become an better programmer.
- Optimized Performance:The efficient data structures and algorithms will lead  you to better code performance.
- Reduced Errors: Adherence to these best practices minimizes the likelihood of errors in you programs which will help you to write good efficient and error free programs and enhance you to be a better programmer.
- Efficient Memory Usage: Avoiding the excessive object creation helps in efficient memory usage in the code.
- Competitive Coding: With large number of student now practicing programming contests over various platforms such gfg contests, these practices will help programmers perform well in such contests and achieve better ranks.
Conclusion
Java coding best practices in programming focus on writing efficient and optimized code that uses minimal memory and runs quickly and efficiently. These techniques will help you to optimize your code and help you to improve in problem-solving, reach good ranks during contests, and help coders write code that is efficient, maintainable, and competitive. In conclusion, adopting these Java programming practices will not only improve your problem solving skills but also contribute to the development of clean, efficient, and maintainable code.Â
FAQs
1. How do Java naming conventions contribute to better code quality?
Java naming conventions ensure consistency in code style, making it easier for developers to understand and collaborate on projects. Following these conventions also improves code maintainability and aids in code analysis.
2. What is the concept of “magic numbers” and why they should be avoided in Java?
Magic numbers are hardcoded numeric values in code. Avoiding them is essential because they make code less readable and maintainable. Replacing magic numbers with named constants or enums improves code clarity and maintainability.
3. How can these Java programming practices improve software development projects?
Following these best practices results in cleaner, more maintainable code, reduces the likelihood of bugs, and makes collaboration with other developers easier. Ultimately, adhering to these practices enhances software quality and developer productivity.
Share your thoughts in the comments
Please Login to comment...