How to use jQuery Each Function in TypeScript ?
Last Updated :
09 Jan, 2024
jQuery is a JavaScript library that can be integrated with TypeScript and the features of both can be used together to enhance the interactivity of the application. In this post, we will learn, how we can use each() method of jQuery in TypeScript with its practical implementation.
Before going to the practical implementation make sure you have the latest version of jQuery installed into your current project. Use the below npm command to install jQuery in TypeScript.
npm install jquery
npm install --save-dev @types/jquery
Import the jQuery into your current file using the below statement.
import variable_name_by_which_you_want_to_import_jQuery from 'jquery'
Example: The below code example explains how you can use the jQuery each() method in TypeScript.
Javascript
import $ from "jquery" ;
declare var global: any;
global.jQuery = $;
import "jquery-ui" ;
$( '.list-item' ).each( function () {
$( this ).on( 'click' , function () {
$( '.result' ).text(`
The ${$( this ).text()}
of the list was clicked!`)
})
});
|
HTML
< ul class = "list" >
< li class = "list-item" >
Item 1
</ li >
< li class = "list-item" >
Item 2
</ li >
< li class = "list-item" >
Item 3
</ li >
< li class = "list-item" >
Item 4
</ li >
< li class = "list-item" >
Item 5
</ li >
</ ul >
< p class = "result" ></ p >
|
Output:
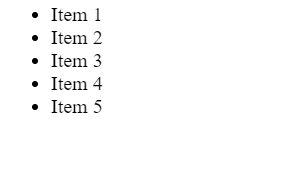
Example 2: The below example shows another practical use case of each method with TypeScript.
Javascript
import $ from "jquery" ;
declare var global: any;
global.jQuery = $;
import "jquery-ui" ;
const arr: (number | string)[] =
[1, 2, 3, "TypeScript" ,
"GeeksforGeeks" ];
$( '#changeText' ).on( 'click' , function () {
$( '.list-item' ).each( function (ind) {
$( this ).text
(`Array item ${ind + 1} =
${arr[ind]}`);
});
})
|
HTML
< ul class = "list" >
< li class = "list-item" >
Item 1
</ li >
< li class = "list-item" >
Item 2
</ li >
< li class = "list-item" >
Item 3
</ li >
< li class = "list-item" >
Item 4
</ li >
< li class = "list-item" >
Item 5
</ li >
</ ul >
< button id = "changeText" >
Change List Item Text
</ button >
|
Output:
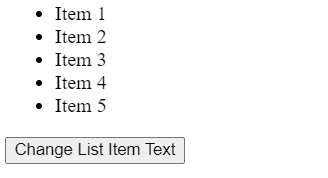
Share your thoughts in the comments
Please Login to comment...