Remove all style, scripts, and HTML tags using BeautifulSoup
Last Updated :
06 Jan, 2023
Prerequisite: BeautifulSoup, Requests
Beautiful Soup is a Python library for pulling data out of HTML and XML files. In this article, we are going to discuss how to remove all style, scripts, and HTML tags using beautiful soup.
Required Modules:
- bs4: Beautiful Soup (bs4) is a python library primarily used to extract data from HTML, XML, and other markup languages. It’s one of the most used libraries for Web Scraping.
Run the following command in the terminal to install this library-
pip install bs4
- requests: This library is used for making HTTP requests in python.
Run the following command in the terminal to install this library-
pip install requests
Approach:
- Import bs4 library
- Create an HTML doc
- Parse the content into a BeautifulSoup object
- Iterate over the data to remove the tags from the document using decompose() method
- Use stripped_strings() method to retrieve the tag content
- Print the extracted data
Implementation:
Python3
from bs4 import BeautifulSoup
HTML_DOC =
def remove_tags(html):
soup = BeautifulSoup(html, "html.parser" )
for data in soup([ 'style' , 'script' ]):
data.decompose()
return ' ' .join(soup.stripped_strings)
print (remove_tags(HTML_DOC))
|
Output:
Geeksforgeeks is a Computer Science portal.
Removing all style, scripts, and HTML tags from an URL
Approach:
- Import bs4 and requests library
- Get content from the given URL using requests instance
- Parse the content into a BeautifulSoup object
- Iterate over the data to remove the tags from the document using decompose() method
- Use stripped_strings() method to retrieve the tag content
- Print the extracted data
Implementation:
Python3
from bs4 import BeautifulSoup
import requests
page = requests.get(URL)
def remove_tags(html):
soup = BeautifulSoup(html, "html.parser" )
for data in soup([ 'style' , 'script' ]):
data.decompose()
return ' ' .join(soup.stripped_strings)
print (remove_tags(page.content))
|
Output:
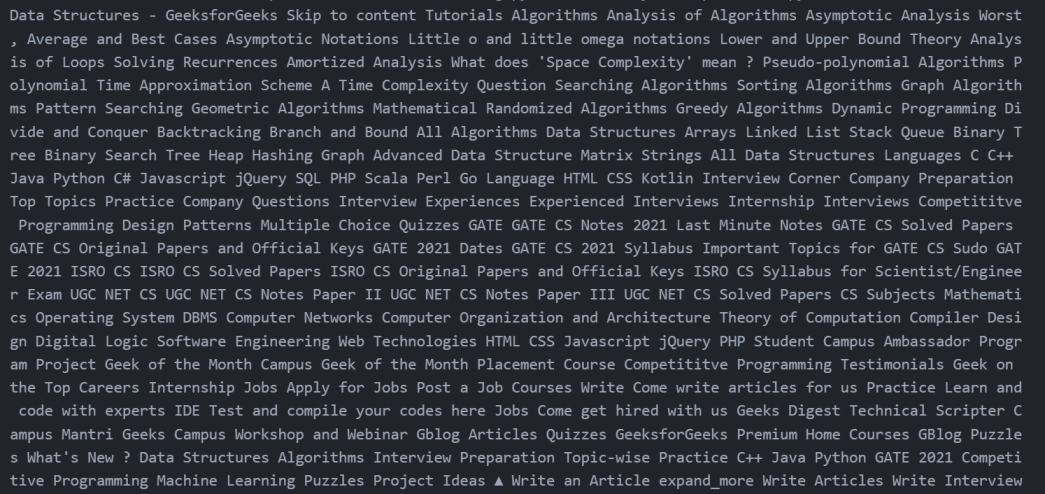
Share your thoughts in the comments
Please Login to comment...