HTML Canvas Lines
Last Updated :
23 Nov, 2023
In this article, we will learn how to make lines in different styles on Canvas in HTML. There are various methods used to draw a line on canvas like beginPath(), moveTo(), lineTo(), and stroke(). There are also various properties like lineWidth, strokeStyle, etc. can be used to give styles to a line with the help of JavaScript.
Syntax
canvas1.lineTo(100, 150);
Canvas Methods for Drawing Lines
Syntax
canvas1.beginPath();
canvas1.moveTo( 0, 0 );
canvas1.lineTo( 120, 150 );
canvas1.stroke();
Methods
- beginPath(): This method defines the new path.
- moveTo(x,y): This method is used to define the starting point.
- lineTo(x,y): This method is used to define the endpoint. Here, x specifies the x-axis coordinates(horizontal) and y specifies the y-axis coordinates(verticle) of the line’s endpoint.
- stroke(): This method is used to draw the line.
Canvas Properties for Drawing Lines
Syntax
canvas2.lineWidth = 10;
canvas1.strokeStyle = "blue";
canvas2.lineCap = "round";
Properties
- lineWidth: In this property, we define the thickness or width of the line.
- strokeStyle: In this property, we define the style of the line.
- lineCap: In this property, we define the cap style that is applied on the top and bottom (start and end) of the line. There are three styles can be used butt, round, and square.
Using the lineTo() method and lineWidth property
The HTML Canvas ‘lineTo’ method delicately connects points, while the ‘lineWidth’ property subtly refines the strokes, resulting in a seamless fusion of precision and style on the digital canvas.
Example 1: In this example, we will draw a line with lineTo() and give the property lineWidth to define the width of the line.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >HTML CANVAS LINES</ title >
< link rel = "stylesheet"
href = "style.css" >
</ head >
< body >
< p >GeeksforGeeks</ p >
< h1 >
HTML CANVAS LINES
</ h1 >
< div class = "flex-class" ></ div >
< canvas height = "200"
width = "200"
class = "canvas-element"
id = "c1" >
</ canvas >
< canvas height = "200"
width = "200"
class = "canvas-element"
id = "c2" >
</ canvas >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
@import url (
body {
font-family : 'Poppins' , sans-serif ;
}
.canvas-element {
border : 2px solid black ;
}
p {
font-size : 50px ;
color : green ;
}
.flex-class {
display : flex;
flex-wrap: wrap;
}
|
Javascript
const canvas1 =
document.getElementById( "c1" ).getContext( "2d" )
const canvas2 =
document.getElementById( "c2" ).getContext( "2d" )
canvas1.beginPath();
canvas1.moveTo(0, 0)
canvas1.lineTo(120, 150)
canvas1.stroke();
canvas2.beginPath();
canvas2.moveTo(10, 10)
canvas2.lineTo(100, 150)
canvas2.lineWidth = 10;
canvas2.stroke();
|
Output:
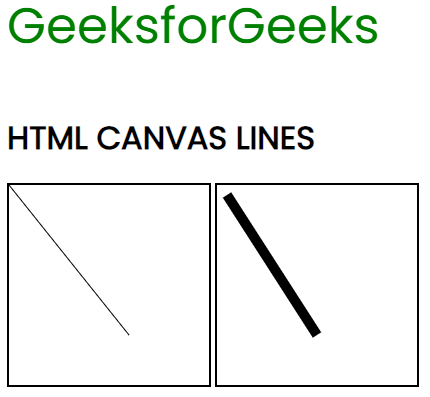
Using the lineTo() method and lineCap property
The ‘lineCap’ property gives your drawings a neat and professional finish and The HTML Canvas ‘lineTo’ method delicately connects points.
Example 2: In this example, we will draw a line with lineTo() with properties.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >HTML CANVAS LINE</ title >
< link rel = "stylesheet"
href = "style.css" >
</ head >
< body >
< p >GeeksforGeeks</ p >
< h1 >
HTML CANVAS LINES
</ h1 >
< div class = "flex-class" ></ div >
< canvas height = "200"
width = "200"
class = "canvas-element"
id = "c1" >
</ canvas >
< canvas height = "200"
width = "200"
class = "canvas-element"
id = "c2" >
</ canvas >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
@import url (
body {
font-family : 'Poppins' , sans-serif ;
}
.canvas-element {
border : 2px solid black ;
}
p {
font-size : 50px ;
color : green ;
}
.flex-class {
display : flex;
flex-wrap: wrap;
}
|
Javascript
const canvas1 =
document.getElementById( "c1" ).getContext( "2d" )
const canvas2 =
document.getElementById( "c2" ).getContext( "2d" )
canvas1.beginPath();
canvas1.moveTo(20, 20)
canvas1.lineTo(70, 150)
canvas1.lineWidth = 3
canvas1.strokeStyle = "blue" ;
canvas1.stroke();
canvas2.beginPath();
canvas2.moveTo(10, 10)
canvas2.lineTo(100, 150)
canvas2.lineWidth = 10
canvas2.strokeStyle = "red"
canvas2.lineCap = "round" ;
canvas2.stroke();
|
Output:
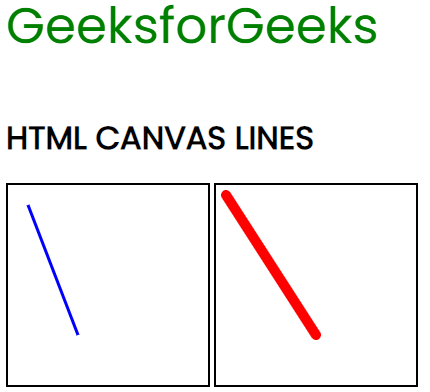
Share your thoughts in the comments
Please Login to comment...