How to use OS with Python List Comprehension
Last Updated :
06 Mar, 2024
One such module is the ‘os’ module, which allows interaction with the operating system. When combined with list comprehensions, the ‘os’ module becomes a potent tool for handling file and directory operations concisely and expressively. In this article, we will see how we can use the os module with list comprehension in Python.
OS with Python List Comprehension
Below are some of the example for using os with List Comprehension in Python:
- Listing Files in a Directory
- Filtering Files with a Specific Extension
- Getting Subdirectories
File Structure
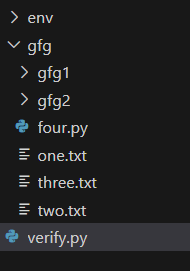
file structure
Listing Files in a Directory
In this example, in below code the Python OS module to list and display the names of files within the specified ‘gfg’ directory. The ‘list_files’ function employs list comprehension to filter only files (not directories) and then prints the resulting list.
Python3
import os
def list_files(directory_path):
files = [ file for file in os.listdir(directory_path) if os.path.isfile(
os.path.join(directory_path, file ))]
print ( "List of files in the directory:" )
for file in files:
print ( file )
if __name__ = = "__main__" :
directory_path = "gfg"
list_files(directory_path)
|
Output:
List of files in the directory:
four.py
one.txt
three.txt
two.txt
Filtering Files with a Specific Extension
In this example, below code uses the ‘os’ module to filter and display the names of files with the ‘.txt’ extension within the specified ‘gfg’ directory. The ‘filter_file’ function employs list comprehension to select only files with the target extension, and then it prints the resulting list.
Python3
import os
def filter_file(directory_path, target_extension):
filtered_files = [ file for file in os.listdir(directory_path) if file .endswith(
target_extension) and os.path.isfile(os.path.join(directory_path, file ))]
print (f "List of {target_extension} files in the directory:" )
for file in filtered_files:
print ( file )
if __name__ = = "__main__" :
directory_path = "gfg"
target_extension = ".txt"
filter_file(directory_path, target_extension)
|
Output:
List of .txt files in the directory:
one.txt
three.txt
two.txt
Getting Subdirectories
In this example, below code uses the ‘os’ module to retrieve and display the names of subdirectories within the specified ‘gfg’ parent directory. The ‘get_subdirectories’ function employs list comprehension to filter only items that are directories, and then it prints the resulting list.
Python3
import os
def get_subdirectories(parent_directory):
subdirectories = [subdir for subdir in os.listdir(
parent_directory) if os.path.isdir(os.path.join(parent_directory, subdir))]
print ( "List of subdirectories in the parent directory:" )
for subdir in subdirectories:
print (subdir)
if __name__ = = "__main__" :
parent_directory = "gfg"
get_subdirectories(parent_directory)
|
Output:
List of subdirectories in the parent directory:
gfg1
gfg2
Share your thoughts in the comments
Please Login to comment...