How to use MUI DataGrid Component with Nested Data in React.js ?
Last Updated :
04 Dec, 2023
DataGrid component in Material-UI is a table component used to display and manage tabular data in React.js applications. It provides a wide range of features and customization options for building complex and interactive data tables. In this article, we will learn how to use the MUI DataGrid component with nested data in ReactJS.
Prerequisites
Steps to create React Application and Installing the required Modules:
Step1: Create a React Application using the following command:
npx create-react-app folder-name
Step 2: Once the project is created move to the project folder by entering the following command in the Terminal:
cd folder-name
Step 3: Now, install the MUI library in the project.
npm install @mui/material @emotion/react @emotion/styled
Step 4: Now, Install the MUI DataGrid package which is required for creating the DataGrid Component by using the following command:
npm install @mui/x-data-grid
Project Structure:Â
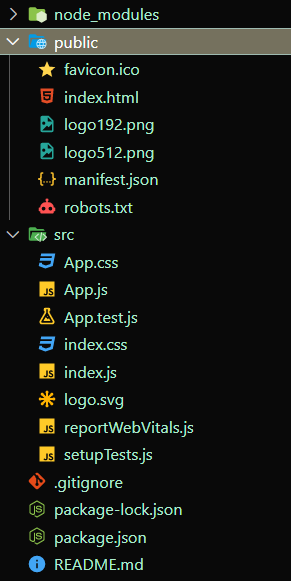
Folder Structure
Example: In this, we use the columns prop to define the structure of the DataGrid and its sub-components. Each column can have its own renderCell function, which takes in the value of the cell and returns a custom rendering for that value. This is useful for rendering nested data, as you can define a column for each level of nesting
Javascript
import { DataGrid } from "@mui/x-data-grid" ;
const columns = [
{ field: "id" , headerName: "ID" , width: 70 },
{ field: "name" , headerName: "Name" , width: 130 },
{
field: "address" ,
headerName: "Address" ,
width: 300,
renderCell: (params) => {
return (
<>
<div>{params.value.street}</div>
<div>
{params.value.city},{params.value.state}{ " " }
{params.value.zip}
</div>
</>
);
},
},
];
const rows = [
{
id: 1,
name: "Geek" ,
address: {
street: "123 GeeksForGeeks" ,
city: "Anytown" ,
state: "Great" ,
zip: "12345" ,
},
},
{
id: 2,
name: "Author" ,
address: {
street: "456 Writer" ,
city: "Writertown" ,
state: "New" ,
zip: "54321" ,
},
},
];
export default function App() {
return (
<div style={{ height: 400, width: "100%" }}>
<DataGrid rows={rows} columns={columns} />
</div>
);
}
|
Step to Run Application:Â
npm start
Output: Now open your browser and go to http://localhost:3000/, and you can see the following output:
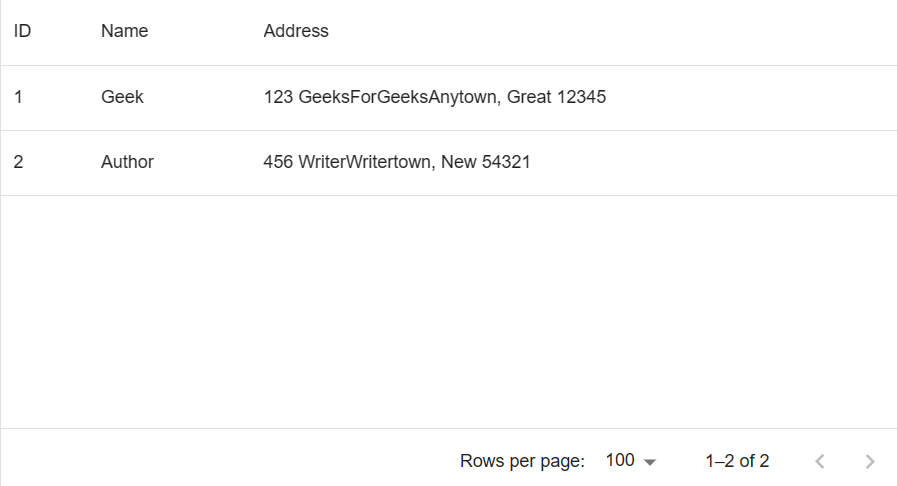
DataGridÂ
Explanation: In the above example, we are displaying the nested data with the use of the renderCell function. The renderCell function returns the component of the data for each row. Hence, the nested data (i.e. street, state, pincode inside the address object) is rendered in the particular cell. Â
Share your thoughts in the comments
Please Login to comment...