How to Use “Is Not NA” in R?
Last Updated :
19 Dec, 2021
In this article, we will discuss how to use Is Not NA in R Programming Language.
NA is a value that is not a number. The is.na() method is used to check whether the given value is NA or not, we have to use the function for this. Inorder to use is NOT NA, then we have to add the “!” operator to the is.na() function
Syntax:
!is.na(data)
where, data can be a vector/list, etc
Is Not NA in Vector
Here we can use this filter to get the values excluding NA values.
Syntax:
vector[!is.na(vector)]
where, vector is the input vector
Example:
R
vector1 = c (1, 2, 3, NA , 34, 56, 78, NA , NA , 34, NA )
print (vector1)
print (vector1[! is.na (vector1)])
|
Output:
[1] 1 2 3 NA 34 56 78 NA NA 34 NA
[1] 1 2 3 34 56 78 34
Is Not NA in dataframe in a single column
If we want to exclude NA values in dataframe columns, then we can use the dataframe similarly to the vector.
Syntax:
dataframe[!(is.na(dataframe$column_name)), ]
where
- dataframe is the input dataframe
- column_name is the column to remove NA values
R
data = data.frame (marks1= c ( NA , 34, 56, 78),
marks2= c (45, 67, NA , NA ))
print (data)
print (data[!( is.na (data$marks1)), ])
print (data[!( is.na (data$marks2)), ])
|
Output:
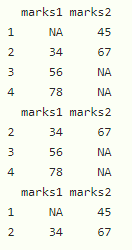
Is Not NA in dataframe in multiple columns
Here we can filter in multiple columns using & operator.
dataframe[!(is.na(dataframe$column1)) & !(is.na(dataframe$column2)),]
Example:
R
data = data.frame (marks1= c ( NA , 34, 56, 78),
marks2= c (45, 67, NA , NA ))
print (data)
print (data[!( is.na (data$marks1)) & !( is.na (data$marks2)), ])
|
Output:
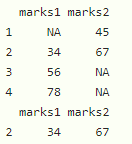
Remove all NA
Here we are going to remove NA’s in the entire dataframe by using na.omit() function
Syntax:
na.omit(dataframe)
Example:
R
data = data.frame (marks1= c ( NA , 34, 56, 78),
marks2= c (45, 67, NA , NA ))
print (data)
print ( na.omit (data))
|
Output:
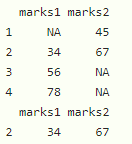
Share your thoughts in the comments
Please Login to comment...