How to use comma as list separator in AngularJS ?
Last Updated :
22 Nov, 2023
In this article, we will use commas as a list separator in AngularJS applications.
In AngularJS, we can use a list separator by simply placing the command between the items in the list. If we have an array of items that we need to display in the application, then we can use ng-repeat to iterate through this array and then display each item which is separated through the comma. So, here we will see 2 different approaches with their implementation.
The below steps will be followed to Install and configure the AngularJS Application:
Step 1: Create a new folder for the project. We are using the VSCode IDE to execute the command in the integrated terminal of VSCode.
mkdir comma-list
cd comma-list
Step 2: Create the index.html file in the newly created folder, we will have all our logic and styling code in this file.
We will understand the above-mentioned approaches with the help of suitable examples.
Using an ng-model and ng-repeat
- We have used the ng-model directive to link the input field with the $scope.inputList variable, enabling user input.
- Then the updateList function uses the ng-change directive when the input field content changes, ensuring it responds to user input.
- Inside the controller, the updateList function processes the user’s input by splitting it into an array using .split(‘,’) and removing the whitespace from each item using .map(item => item.trim()).
- Then we store the processed list of items in the $scope.itemList variable for display in the HTML.
- Using the ng-repeat directive to dynamically generate list items within an unordered list, displaying each item from itemList in the HTML with commas as separators.
Example: Below is an example that demonstrates the use of commas as a list separator in AngularJS using ng-model and ng-repeat Directives.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
text-align: center;
}
.container {
width: 400px;
margin: 0 auto;
}
h1 {
color: green;
}
h3 {
color: blue;
}
input {
width: 100%;
padding: 10px;
margin: 10px 0;
}
ul {
list-style: none;
padding: 0;
}
li {
border: 1px solid #ccc;
padding: 5px;
margin: 5px 0;
background-color: #f7f7f7;
}
</ style >
</ head >
< body ng-controller = "ListController" >
< div class = "container" >
< h1 >GeeksforGeeks</ h1 >
< h3 >
Example 1: Using an ng-model and ng-repeat
</ h3 >
< label for = "inputList" >
Enter a comma-separated list:
</ label >
< input type = "text"
id = "inputList"
ng-model = "inputList"
ng-change = "updateList()" >
< ul >
< li ng-repeat = "item in itemList" >
{{ item }}
</ li >
</ ul >
</ div >
< script >
var app = angular.module('myApp', []);
app.controller('ListController', function ($scope) {
$scope.inputList = '';
$scope.updateList = function () {
$scope.itemList = $scope.inputList.split(',').
map(item => item.trim());
};
});
</ script >
</ body >
</ html >
|
Output:
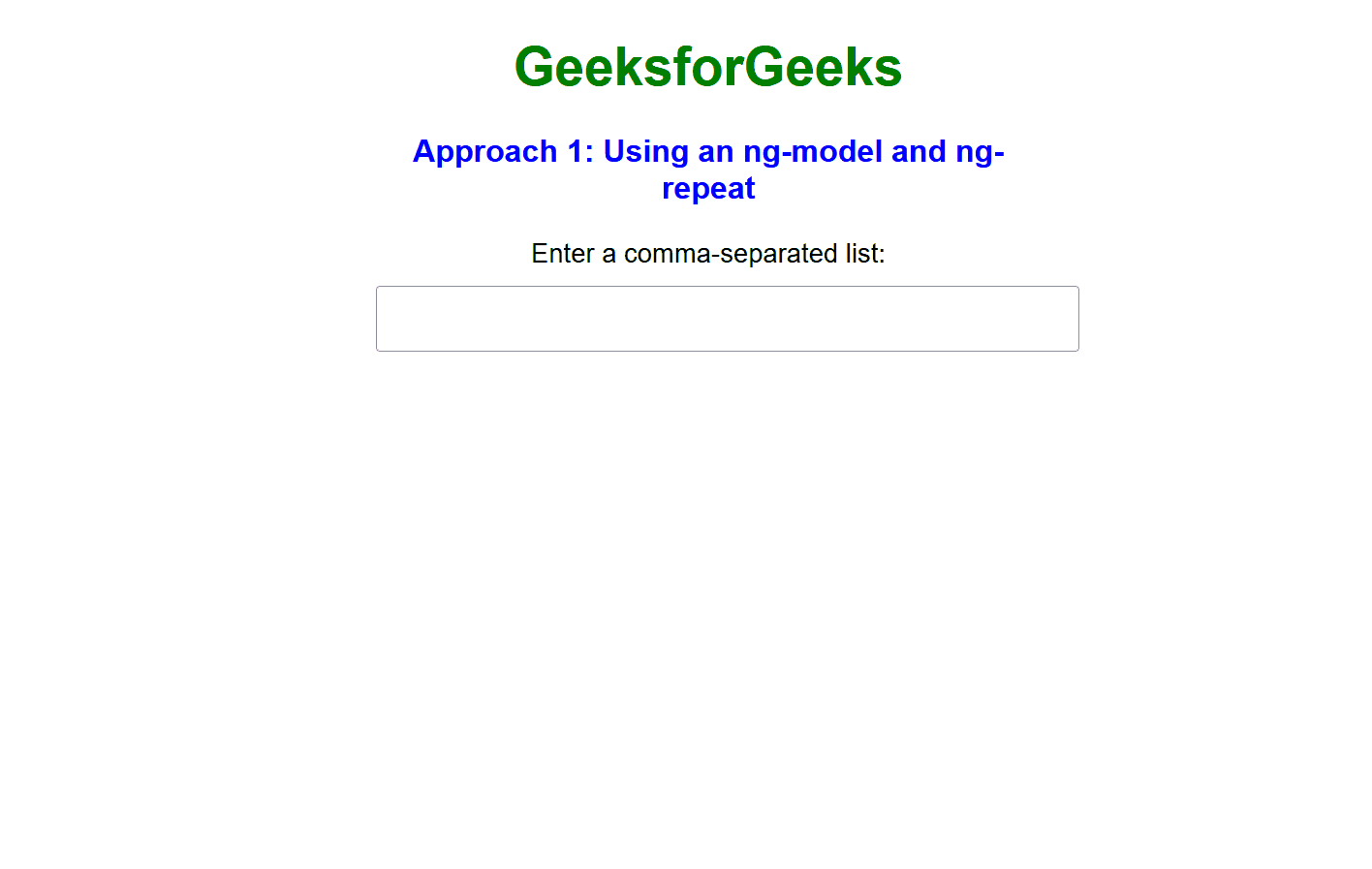
Using ng-change and custom JavaScript function
- In this approach, we have used the ng-change directive that is used to detect changes in the input field and execute the “processInput” function.
- When a user enters a comma-separated list in the input field, this function splits the input into individual items, trims any extra whitespace, and filters out empty items.
- The resulting list of items is displayed in an unordered list.
Example: Below is an example that demonstrates the use of commas as a list separator in AngularJS using ng-change and custom JavaScript functions.
HTML
<!DOCTYPE html>
< html ng-app = "listApp" >
< head >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
text-align: center;
}
h1 {
color: green;
}
#itemInput {
width: 60%;
padding: 10px;
margin: 10px;
font-size: 16px;
}
ul {
list-style-type: none;
padding: 0;
}
ul li {
background-color: #f0f0f0;
margin: 5px;
padding: 10px;
border-radius: 5px;
}
button {
background-color: red;
color: white;
border: none;
padding: 10px 20px;
margin: 10px;
cursor: pointer;
}
</ style >
</ head >
< body ng-controller = "ListController" >
< h1 >GeeksforGeeks</ h1 >
< h3 >
Approach 2: Using ng-change and
custom JavaScript function
</ h3 >
< label for = "itemInput" >
Enter items (comma-separated):
</ label >
< input type = "text"
id = "itemInput"
ng-model = "itemInput"
ng-change = "processInput()"
placeholder = "E.g., Geeks, Article, AngularJS" >
< p >Items entered:</ p >
< ul >
< li ng-repeat = "(index, item) in itemList" >
{{ index + 1 }}. {{ item }}
</ li >
</ ul >
< script >
var app = angular.module('listApp', []);
app.controller('ListController', function ($scope) {
$scope.itemInput = "";
$scope.itemList = [];
$scope.processInput = function () {
var items =
$scope.itemInput.split(',').map(function (item) {
return item.trim();
});
items = items.filter(function (item) {
return item !== '';
});
$scope.itemList = items;
var duplicates = findDuplicates(items);
if (duplicates.length > 0) {
alert("Duplicate items detected: " +
duplicates.join(", "));
}
};
function findDuplicates(arr) {
return arr.filter((item, index) =>
arr.indexOf(item) !== index);
}
});
</ script >
</ body >
</ html >
|
Output:
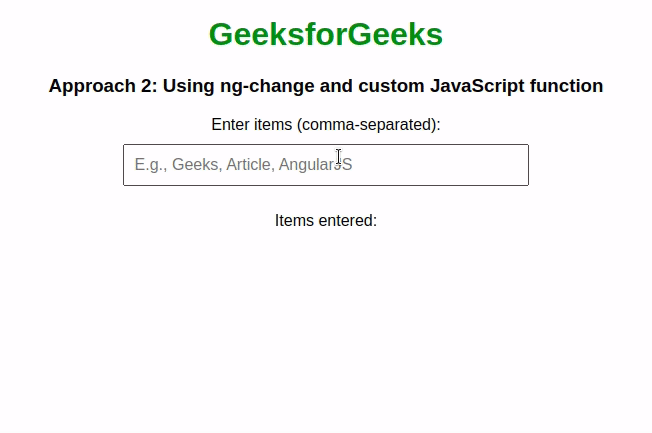
Share your thoughts in the comments
Please Login to comment...