How to truncate the text using Angular Pipe and Slice ?
Last Updated :
18 Dec, 2023
Angular Pipe and Slice can be used to manipulate or transform the data in a specific format. Angular Pipes can be utilized to format or transform data directly before displaying it in the Angular template. The Slice is a built-in method in JavaScript that enables the users to extract a part of the array or string without modifying the original data. Text Truncation is the process of shortening text content.
In this article, we will learn to truncate the text using Angular Pipe and Slice.
Syntax
{{ inputValue | pipename : parameter }}
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Step 3: Creating Pipe
ng generate pipe geek
Project Structure
It will look like the following:
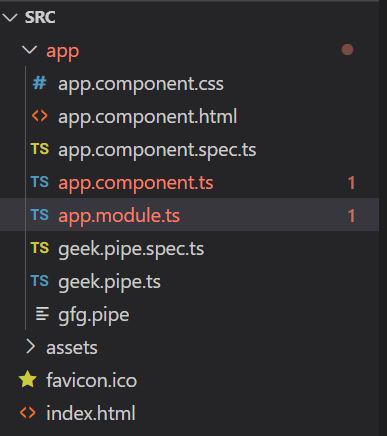
Example 1: In this example, we will use the slice function to truncate the text.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >Truncating text in Angular using Pipe and Slice </ h2 >
< b >Original Text:</ b > {{str}}
< br >
< b >Truncated Text:</ b > {{str | slice:0:6}}
|
Javascript
import { DatePipe } from '@angular/common' ;
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
})
export class AppComponent {
str: string = 'Geeks Premier League 2023' ;
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { FormsModule }
from '@angular/forms'
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
HttpClientModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
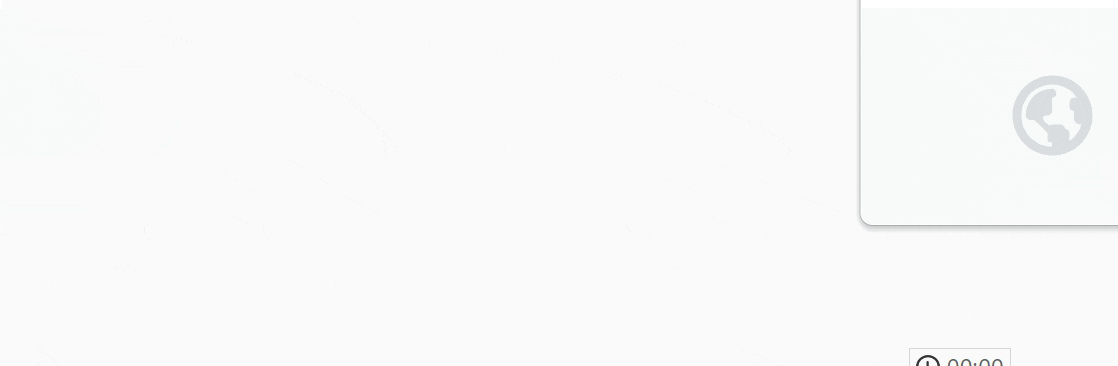
Example 2: In this example, we will use the custom pipe to truncate the text.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >Truncating text in Angular using Pipe and Slice </ h2 >
< b >Original Text:</ b > {{str}}
< br >
< b >Truncated Text:</ b > {{str | truncate:[6]}}
|
Javascript
import { DatePipe } from '@angular/common' ;
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
})
export class AppComponent {
str: string = 'Geeks Premier League 2023' ;
}
|
Javascript
import { Pipe, PipeTransform } from '@angular/core' ;
@Pipe({
name: 'truncate'
})
export class GeekPipe implements PipeTransform {
transform(value: string, args: any[]): string {
const limit =
args.length > 0 ? parseInt(args[0], 10) : 20;
const trail = args.length > 1 ? args[1] : '' ;
return
value.length > limit ? value.substring(0, limit) +
trail : value;
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { FormsModule }
from '@angular/forms'
import { AppComponent }
from './app.component' ;
import { GeekPipe }
from './geek.pipe' ;
@NgModule({
declarations: [
AppComponent,
GeekPipe
],
imports: [
BrowserModule,
HttpClientModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
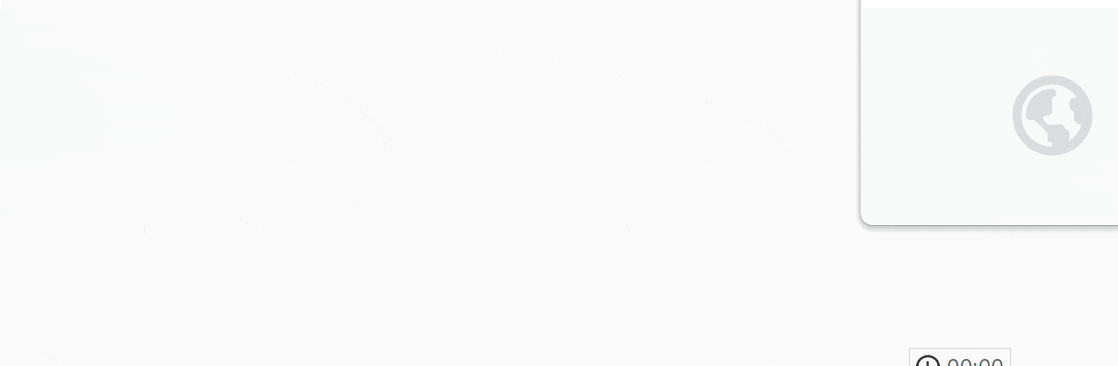
Share your thoughts in the comments
Please Login to comment...