How to Toggle a Class in Vue.js ?
Last Updated :
27 Sep, 2023
In this article, we will use Vue.js component with a simple HTML template containing <div>
and button elements. The goal is to toggle the presence of a CSS class on the <div>
element when the button is clicked. By understanding the concepts of data binding, conditional rendering, and event handling in Vue.js, you’ll be able to create more interactive and visually appealing user interfaces.
Let’s dive into the implementation step-by-step and see how Vue.js makes class toggling a breeze. By the end of this article, you’ll have a solid grasp of this fundamental feature, allowing you to build more sophisticated and dynamic Vue.js applications. So, let’s get started!
Approach:
Step 1: Setting up the HTML template
First, let’s set up the HTML template. We’ll use a <div>
element where we want to toggle a class based on a condition. Additionally, we’ll include a button that triggers the class toggling. Here’s the initial HTML code:
<template>
<div>
<img src="https://media.geeksforgeeks.org/gfg-gg-logo.svg" alt="">
</div>
<button @click="toggleClass">Toggle Class</button>
</template>
In the above code, we use the :class
directive to bind a class dynamically to the <div>
element. The class binding is defined using an object, where the keys represent the class names, and the values are the conditions that determine whether the class should be applied.
Step 2: Defining the data property
Next, we need to define the data property that holds the condition for toggling the class. In the Vue.js component, we’ll define a data
object that includes the isActive
property. This property will control whether the class is applied or not. Here’s the code:
Javascript
export default {
data() {
return {
isActive: false
};
},
}
|
In the above code, we initialize the isActive
property to false
, indicating that the class 'my-class'
is not applied initially.
Step 3: Creating the toggleClass Method
To toggle the class, we need to create a method that will be triggered when the button is clicked. In the Vue.js component, define a method called toggleClass
that will toggle the value of isActive
. Here’s the code:
HTML
< script >
export default {
data() {
return {
isActive: false
};
},
methods: {
toggleClass() {
this.isActive = !this.isActive;
}
}
}
</ script >
< style >
body {
text-align: center;
}
.my-class {
display: none;
}
button {
margin: 1rem 0;
}
</ style >
|
In the above code, the toggleClass
method is defined. It uses the negation operator (!
) to toggle the value of isActive
. If isActive
is false
, it becomes true
, and vice versa. This method will be executed when the button is clicked.
Step 4: Binding the class and button event
Now that we have the necessary methods and data property, let’s bind the class and the button event to their respective elements in the HTML template. Here’s the updated code:
<button @click="toggleClass">Toggle Class</button>
In the above code, the :class
directive binds the class 'my-class'
to the <div>
element. It is conditionally applied based on the value of isActive
. The @click
directive on the button element triggers the toggleClass
method when the button is clicked.
By utilizing the :class
directive and a data property, we were able to dynamically bind a class to an element based on a condition. Additionally, we created a method that toggles the condition when the button is clicked. This approach provides an interactive way to toggle classes in your Vue.js application.
Example:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" />
< script src =
</ script >
< style >
body {
padding: 0;
margin: 0;
box-sizing: border-box;
}
</ style >
</ head >
< body >
< script type = "module" src = "/index.js" ></ script >
</ body >
</ html >
|
Output:
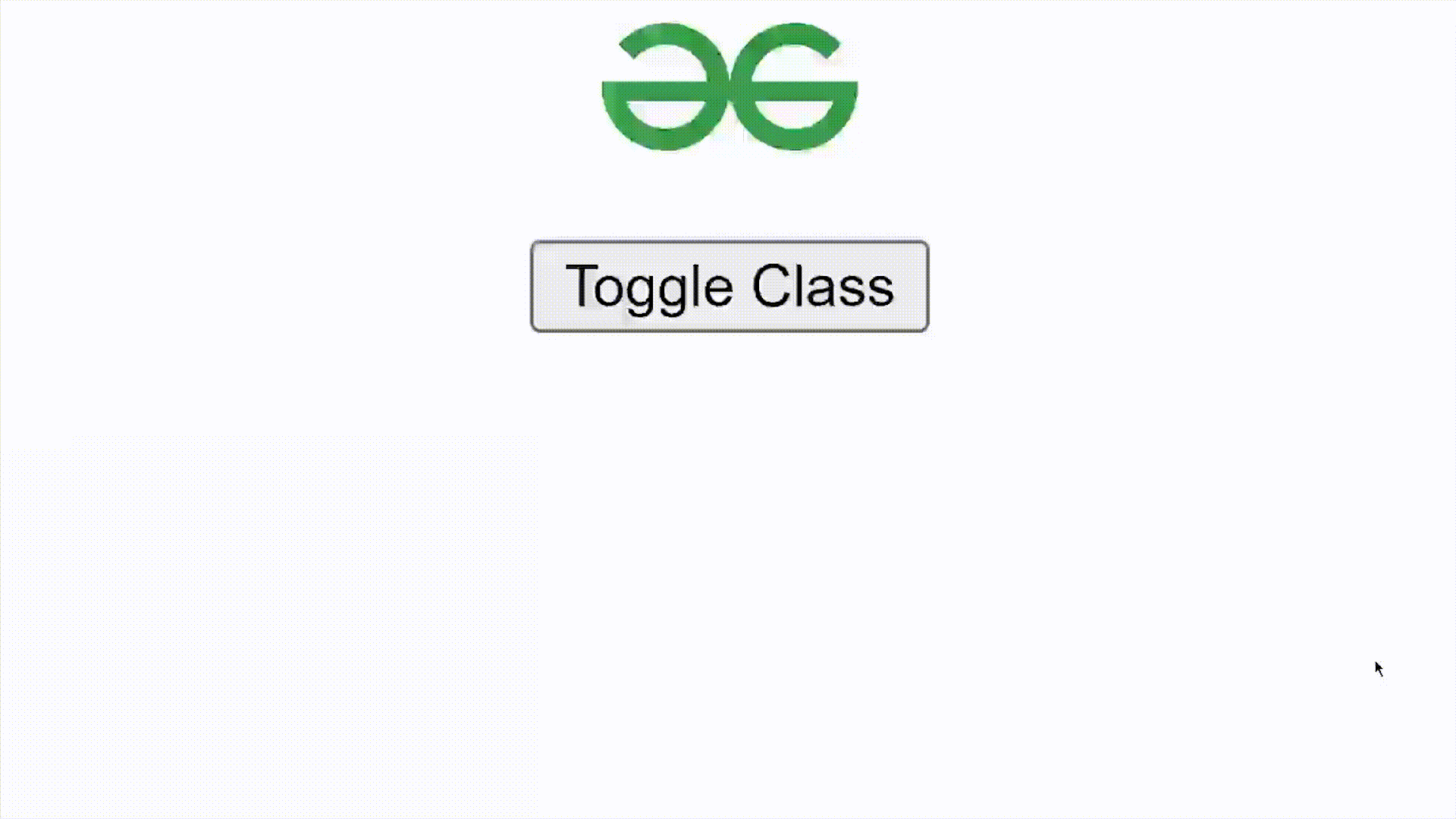
Share your thoughts in the comments
Please Login to comment...